In the world of computer brain-building, processors are like super-smart puzzle solvers. To make them even better, we use something called Finite State Machines (FSMs). In this blog post, we’re going to talk about why we add FSMs to the processor’s brain (RTL) and how we can do it in simple steps.
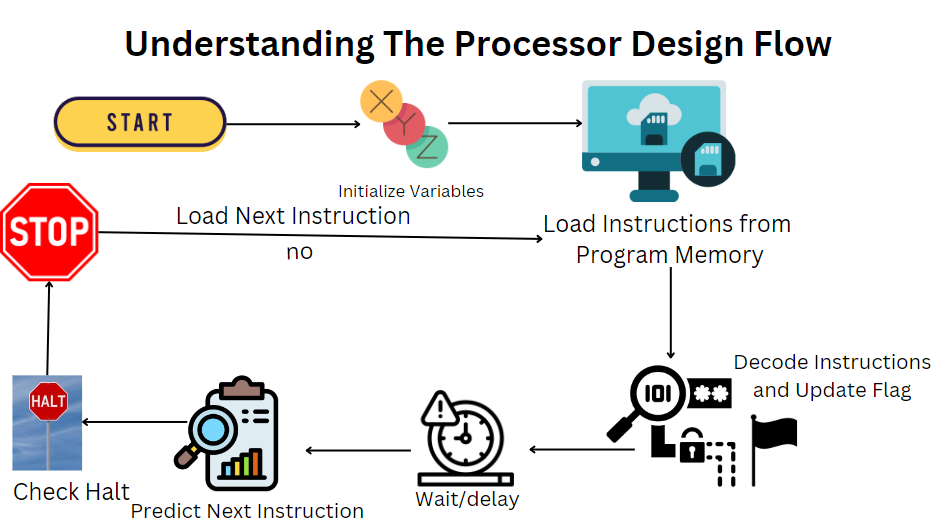


Reset Detector
always@(posedge clk)
begin
if(sys_rst)
state <= idle;
else
state <= next_state;
end

idle: begin
IR = 32'h0;
PC = 0;
next_state = fetch_inst;
end
fetch_inst: begin
IR = inst_mem[PC];
next_state = dec_exec_inst;
end
dec_exec_inst: begin
decode_inst();
decode_condflag();
next_state = delay_next_inst;
end
With this we have decoded the instruction, we will be giving a delay for next instruction to be loaded
which we will discuss soon!

next_inst: begin
next_state = sense_halt;
if(jmp_flag == 1'b1)
PC = `isrc;
else
PC = PC + 1;
end
sense_halt: begin
if(stop == 1'b0)
next_state = fetch_inst;
else if(sys_rst == 1'b1)
next_state = idle;
else//////////////////next state decoder + output decoder
always@(*) * because it is a combinational block
begin
case(state)
idle: begin
IR = 32'h0;
PC = 0;
next_state = fetch_inst;
end
fetch_inst: begin
IR = inst_mem[PC];
next_state = dec_exec_inst;
end
dec_exec_inst: begin
decode_inst();
decode_condflag();
next_state = delay_next_inst;
end
delay_next_inst:begin
end
next_inst: begin
next_state = sense_halt;
if(jmp_flag == 1'b1)
PC = `isrc;
else
PC = PC + 1;
end
sense_halt: begin
if(stop == 1'b0)
next_state = fetch_inst;
else if(sys_rst == 1'b1)
next_state = idle;
else
next_state = sense_halt;
end
default : next_state = idle;
endcase
end
next_state = sense_halt;
end
Integrating delay in above combinational circuit will not work..
Because it is sequential so we will create a new always clock block with positive edge of clock
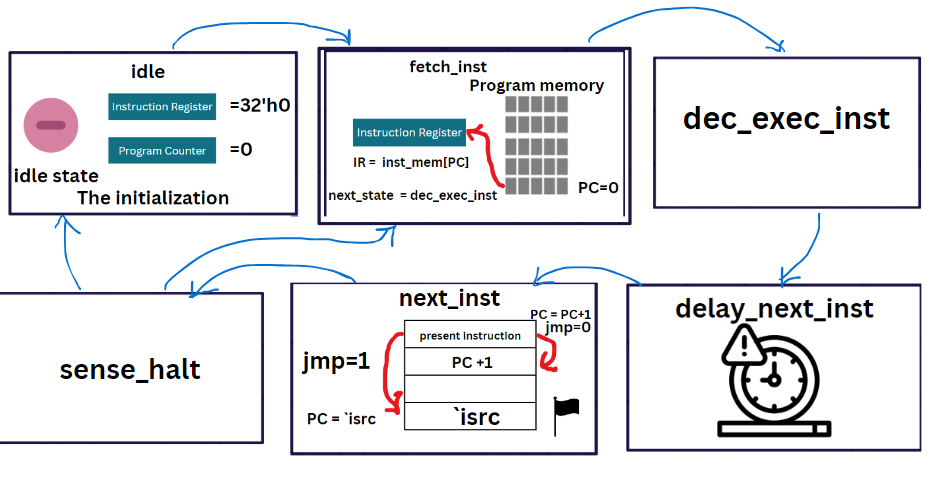
Now we know that next instruction will be fetched after count of 4

delay_next_inst:begin
if(count < 4)
next_state = delay_next_inst;
else
next_state = next_inst;
end
always@(posedge clk)
begin
case(state)
idle : begin
count <= 0;
end
fetch_inst: begin
count <= 0;
end
dec_exec_inst : begin
count <= 0;
end
delay_next_inst: begin
count <= count + 1;
end
next_inst : begin
count <= 0;
end
sense_halt : begin
count <= 0;
end
default : count <= 0;
endcase
However if you’ve only taken prednisone for a quick time, you could possibly
cease remedy without having to progressively reduce your dose.
Prednisone could also be used during pregnancy
at the lowest dose and for the shortest time period wanted.
For example, in case you have fluid retention or high blood pressure
during your being pregnant, prednisone might make your situation worse.
Always follow your doctor’s directions when stopping prednisone treatment.
This is simply because of the close to certainty that exceeding the beneficial dose will quickly bring
indicators of virilization. Your experience stage and targets
will considerably decide the dosage you’ll be
comfortable with. Because of liver toxicity issues, greater
doses than this are only recommended to be accomplished when injecting Winstrol.
Most folks utilizing Winstrol whereas chopping will probably wish
to add one other steroid to aid in larger lean best mass building steroid preservation than Winstrol can provide.
Winstrol does promote nitrogen retention and increased protein synthesis, however not to the point the place it’ll noticeably promote lean features – despite its excessive anabolic rating.
Nonetheless, abrupt discontinuation of prednisone with out medical supervision just isn’t really
helpful. These female-specific prednisone unwanted facet
effects in ladies talked about are comparatively uncommon side effects.
“Prednisone can each cause bleeding when it shouldn’t, and it could stop bleeding [during a period],” explains Megan Milne, Pharm.D.,
a scientific pharmacist who makes a speciality of prednisone
unwanted effects. “Most concerning for girls still anticipating a period is when their periods don’t happen.”
If you discover irregular intervals or absent intervals whereas on prednisone, contact your healthcare supplier
for medical advice. Your girlfriend may take the same anabolic steroid that you do and have completely different
reactions.
If you do check positive, you might be banned
from competing professionally. Testosterone may improve a woman’s
risk for breast cancer, heart disease, and blood clotting.
Additional analysis is needed to completely perceive
how testosterone may be linked to these circumstances.
I can’t mention enough instances that the higher the standard or purity of your Anavar is, the less of
it you’ll have to take to get the specified effects and outcomes.
However, with the assistance of in-depth interviews and help for
reflection, substantive meanings have emerged.
In-depth interviews as a technique have been shown to
be a power so as to gain a deeper understanding. The method is time-consuming which is a weakness and requires knowledge in interview know-how.
In common, the side effects of prednisone are the identical in both females and males.
But, females could discover some modifications to their menstrual
cycle throughout their prednisone treatment. They may have a better danger of osteoporosis whereas
taking prednisone. Prednisone causes related side effects in males and females, but
a few of its side effects could only occur in (or are more common in) females.
But, each analysis and our expertise indicate that
Anadrol would not have the same androgenic impact on ladies.
Ought To Primobolan be well-tolerated within the initial 4 weeks,
it is possible to increase the dosage to 75mg every day for the subsequent 2 weeks.
Additionally, in later cycles, a every day dose of 75mg may be considered, extending
the cycle length to 8 weeks, as opposed to the preliminary
6-week cycle. While these side effects can range from person to person, it’s essential
to bear in mind of them, particularly when contemplating the influence on women’s
well being. In this article I will explain the common unwanted side effects of prednisone in women and provide some insights on how to
manage them with a free gift on the end.
You may discover that the muscle contracts or shakes by itself and no amount of stretching can help.
Anabolic steroids, also referred to as “roids,” are a man-made
compound that mimic the male sex hormone, testosterone.
This synthetic creation of testosterone captures both the anabolic and androgenic benefits of the hormone.
Clenbuterol, while not a steroid, is commonly mistakenly classified as one because of its significant fat-burning properties.
Steroids can induce infertility in women by interfering with the pure technology of ovulatory hormones.
Steroids can increase the danger of coronary heart disease and stroke, particularly in girls who have used these medications for an extended
period. Kesimpta (ofatumumab) is used to deal with relapsing forms
of a quantity of sclerosis. Do not take different medicines unless
they have been mentioned with your physician. This
consists of prescription or nonprescription (over-the-counter [OTC]) medicines
and herbal or vitamin supplements. Make sure any physician or dentist
who treats you knows that you are utilizing this medicine.
Keep Away From people who are sick or have infections and
wash your arms often.
Tapering dose packs beginning at high doses and tapering day by day over 7 to 9
days are commercially obtainable and can be used in these conditions as nicely.
Clinicians must make every effort to use the glucocorticoids on the lowest potential dose and for the shortest possible length in these
instances. A gradual taper shall be attempted in sufferers with
prolonged exposure to glucocorticoids to forestall
adrenal disaster. As one of the least androgenic
steroids available on the market, Anavar does not convert estrogen into DHT when taken in small doses.
Nonetheless, at doses of 100mg or extra,
it may start to have an result on hair development and hair follicles.