Course Description
This course provides an introduction to design patterns in embedded C programming. Design patterns are proven solutions to common problems that arise during software design. In this course, you will learn about various design patterns that can be applied to embedded systems programming using the C language.
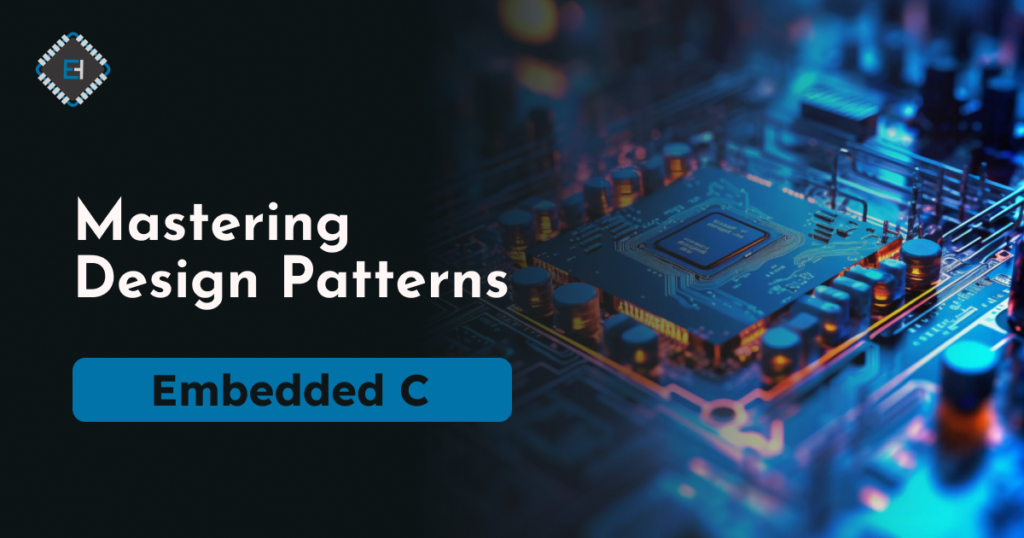
Course Objectives
- Understand the fundamentals of design patterns.
- Learn how design patterns can be applied to embedded systems programming.
- Gain hands-on experience through practical examples and exercises.
Module 1: Introduction to Design Patterns
1.1 What Are Design Patterns?
- Definition and importance of design patterns
- Categories of design patterns
1.2 Why Use Design Patterns in Embedded Systems?
- Benefits of using design patterns
- Challenges in embedded systems programming
1.3 Overview of Embedded Systems
- Characteristics of embedded systems
- Common applications and examples
Module 2: Basic Embedded C Programming Concepts
2.1 Introduction to Embedded C
- Differences between C and embedded C
- Embedded C programming basics
2.2 Data Types and Variables
- Primitive data types
- User-defined data types
- Variable declaration and initialization
2.3 Control Structures
- Conditional statements
- Loops
- Switch case statements
Module 3: Creational Design Patterns
3.1 Object Pattern
- Definition and implementation
- Use cases in embedded systems
3.2 Opaque Pattern
- Definition and implementation
- Use cases in embedded systems
3.3 Singleton Pattern
- Definition and implementation
- Use cases in embedded systems
3.4 Factory Pattern
- Definition and implementation
- Use cases in embedded systems
Module 4: Structural Design Patterns
4.1 Adapter Pattern
- Definition and implementation
- Use cases in embedded systems
4.2 Proxy Pattern
- Definition and implementation
- Use cases in embedded systems
4.3 Decorator Pattern
- Definition and implementation
- Use cases in embedded systems
Module 5: Behavioral Design Patterns
5.1 Observer Pattern
- Definition and implementation
- Use cases in embedded systems
5.2 State Pattern
- Definition and implementation
- Use cases in embedded systems
5.3 Strategy Pattern
- Definition and implementation
- Use cases in embedded systems
Module 6: Practical Applications and Case Studies
6.1 Case Study 1: Implementing a Real-Time System
- Applying design patterns to a real-world example
6.2 Case Study 2: Developing an Embedded Communication Protocol
- Design patterns for efficient data communication
6.3 Hands-on Exercises
- Practical exercises to reinforce learning
Module 7: Conclusion and Further Learning
7.1 Summary of Key Concepts
- Recap of design patterns covered
- Importance in embedded systems programming
7.2 Resources for Further Learning
- Recommended books, articles, and online courses
7.3 Course Assessment
- Final quiz
- Feedback and course evaluation
Additional Resources
- Recommended reading materials
- Online forums and communities
- Software tools and IDEs for embedded C programming
This course structure provides a comprehensive overview of design patterns in embedded C programming. Each module is designed to build upon the previous one, ensuring a structured and cohesive learning experience.
Interested
Good