When programming in the C language, variables play a pivotal role in storing and managing data. They come in various flavors, each serving a specific purpose within the program’s scope. In this blog, we’ll delve into the world of local, global, extern, and static variables in C, exploring their definitions, usage, and implications.
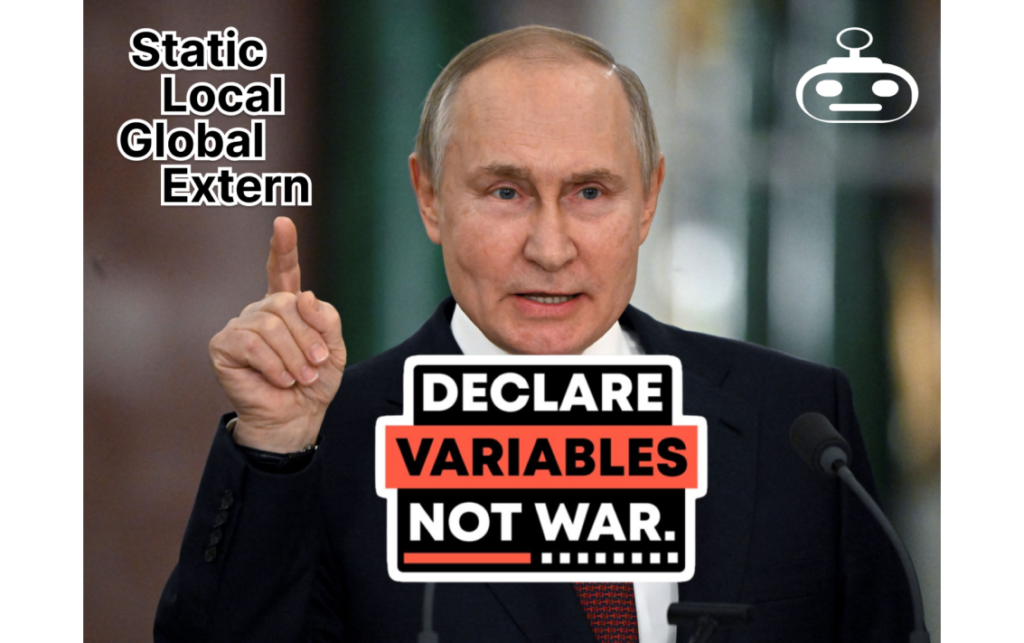
1. Local Variables:
Local variables are declared within a function or a code block and are accessible only within that particular scope. They are allocated memory when the function is called or the block is executed, and their memory is released when the function/block exits. Local variables are useful for temporary data storage and are isolated from other parts of the program, preventing unintended interference.
Example:
#include <stdio.h>
void exampleFunction() {
int localVar = 10;
printf("Local variable: %d\n", localVar);
}
int main() {
exampleFunction();
// localVar is not accessible here
return 0;
}
2. Global Variables:
Global variables are declared outside of any function and have a global scope, meaning they can be accessed and modified from any part of the program. They are initialized once when the program starts and retain their values until the program terminates. Global variables should be used cautiously, as they can lead to potential issues like unintended modification or naming conflicts.
Example:
#include <stdio.h>
int globalVar = 20;
void modifyGlobal() {
globalVar += 5;
}
int main() {
printf("Global variable: %d\n", globalVar);
modifyGlobal();
printf("Modified global variable: %d\n", globalVar);
return 0;
}
3. Extern Variables:
An extern
variable is declared with the extern
keyword and is used to access a global variable that is defined in a different file. The extern
keyword indicates that the variable is defined elsewhere and prevents the compiler from allocating memory for it. It’s particularly useful when you want to share variables across multiple files in a program.
Example:
// file1.c
#include <stdio.h>
int count;
void demo()
{
count++;
}
In this file, we declare a global variable count
without initializing it.
// file2.c
#include <stdio.h>
extern int count;
int main()
{
printf("count: %d\n", count);
demo();
printf("count: %d\n", count);
demo();
printf("count: %d\n", count);
return 0;
}
Output:
Count: 0
Count: 1
Count: 2
In this file, we use the extern
keyword to indicate that count
is defined in another file. We then print the value of count
, which is initialized to 0. We call the demo()
function, which increments the value of count
. We then print the value of count
again, which is now 1. We call the demo()
function again and print the value of count
, which is now 2.
4. Static Variables:
Static variables, when declared inside a function, retain their values between function calls. They are initialized only once, the first time the function is called, and then they maintain their values across subsequent calls. Static variables have a local scope but a longer lifetime compared to regular local variables.
Example:
#include <stdio.h>
void counter() {
static int count = 0;
count++;
printf("Count: %d\n", count);
}
int main() {
counter();
counter();
return 0;
}
Output:
Count: 1
Count: 2
Understanding these types of variables in C is crucial for effective memory management, scope control, and preventing unintended side effects. By using them appropriately, you can write more structured, modular, and maintainable code.
Leave a comment