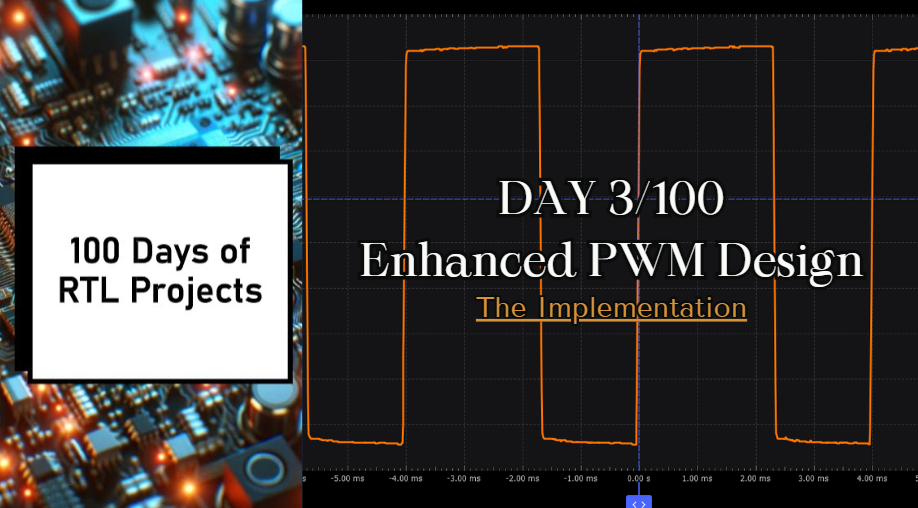
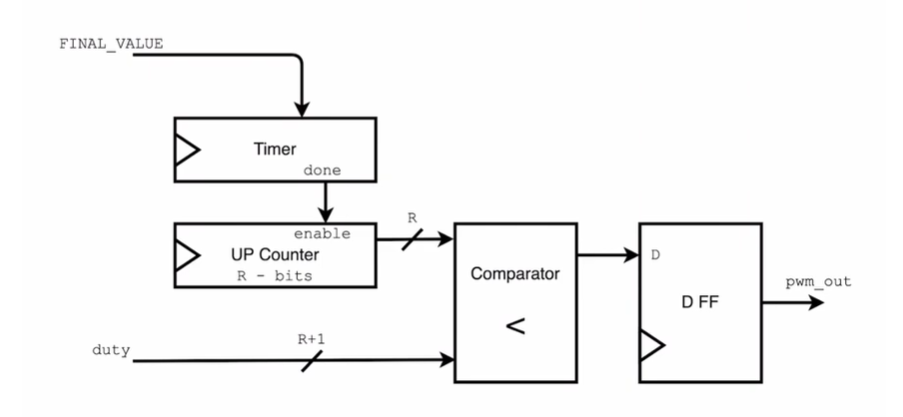
module pwm_basic
#(parameter R = 8)(
input clk,
input reset_n,
input [R – 1:0] duty,
output pwm_out
);
// Up Counter
reg [R - 1:0] Q_reg, Q_next;
always @(posedge clk, negedge reset_n)
begin
if (~reset_n)
Q_reg <= 'b0;
else
Q_reg <= Q_next;
end
// Next state logic
always @(*)
begin
Q_next = Q_reg + 1;
end
// Output logic
assign pwm_out = (Q_reg < duty);
endmodule
This is our previous design let us see how we can enhance it
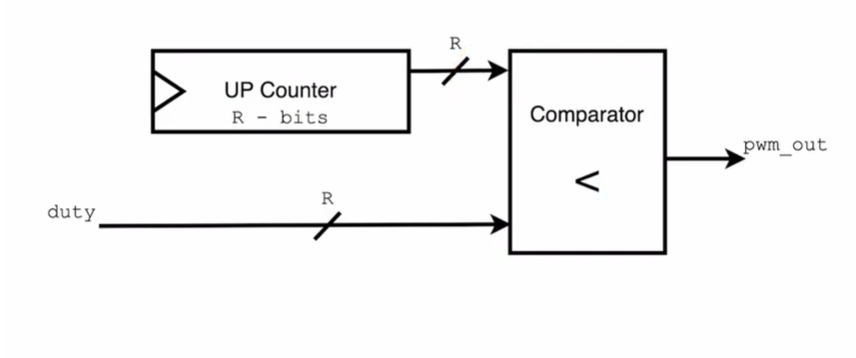
First we will code the input timer module
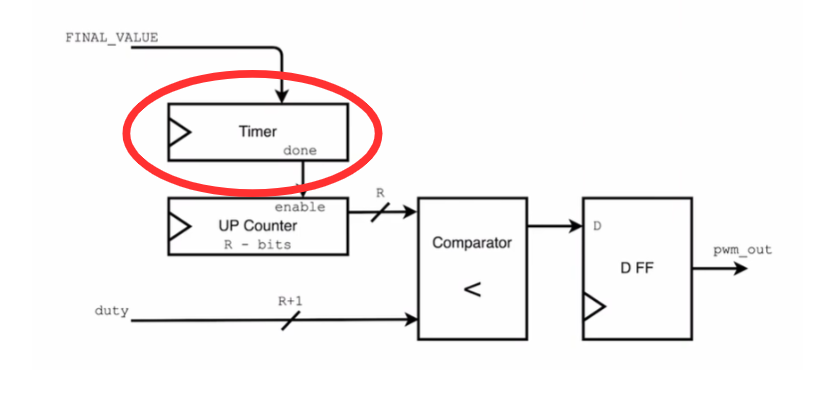
module timer_input
#(parameter BITS = 4)(
input clk,
input reset_n,
input enable,
input [BITS – 1:0] FINAL_VALUE,
// output [BITS – 1:0] Q,
output done
);
reg [BITS - 1:0] Q_reg, Q_next;
always @(posedge clk, negedge reset_n)
begin
if (~reset_n)
Q_reg <= 'b0;
else if(enable)
Q_reg <= Q_next;
else
Q_reg <= Q_reg;
end
// Next state logic
assign done = Q_reg == FINAL_VALUE;
always @(*)
Q_next = done? 'b0: Q_reg + 1;
endmodule
This is a synchronous reset block triggered on the positive edge of the clock and the negative edge of the reset signal.
begin if (~reset_n) Q_reg <= 'b0;
If the reset signal is active (low), the counter is reset to zero.
else if(enable) Q_reg <= Q_next;
If the enable signal is active, the current state of the counter (Q_next
) is loaded into Q_reg
.
else Q_reg <= Q_reg;
Otherwise, the counter remains in its current state.
assign done = Q_reg == FINAL_VALUE;
The done
output is asserted when the counter reaches the FINAL_VALUE
.
always @(*) Q_next = done? 'b0: Q_reg + 1;
This is the next state logic. It increments the counter (Q_reg
) by 1 unless the done
signal is asserted, in which case the counter resets to 0.
Now we will connect timer module with counter , Let us see how
module pwm_improved
#(parameter R = 8, TIMER_BITS = 15)
(
input clk,
input reset_n,
input [R:0] duty, // Control the Duty Cylce,this is the modified duty cycle
input [TIMER_BITS - 1:0] FINAL_VALUE, // Control the switching frequency
output pwm_out
);
// Up Counter
reg [R - 1:0] Q_reg, Q_next;
wire tick;
always @(posedge clk, negedge reset_n)
begin
if (~reset_n)
Q_reg <= 'b0;
else
Q_reg <= Q_next;
end
// Next state logic
always @(*)
begin
Q_next = Q_reg + 1;
end
// Output logic
assign pwm_out = (Q_reg < duty);
// Prescalar Timer
timer_input #(.BITS(TIMER_BITS)) timer0 (
.clk(clk),
.reset_n(reset_n),
.enable(1'b1),
.FINAL_VALUE(FINAL_VALUE),
.done(tick)
);
endmodule

Now let us connect timer output to up counter
module pwm_improved
#(parameter R = 8, TIMER_BITS = 15)(
input clk,
input reset_n,
input [R:0] duty, // Control the Duty Cylce
input [TIMER_BITS – 1:0] FINAL_VALUE, // Control the switching frequency
output pwm_out
);
reg [R - 1:0] Q_reg, Q_next;
reg d_reg, d_next;
wire tick;
// Up Counter
always @(posedge clk, negedge reset_n)
begin
if (~reset_n)
begin
Q_reg <= 'b0;
end
else if (tick)
begin
Q_reg <= Q_next; ///this is how we connect the timer output i.e tick with counter,when we get new output from timer the counter is updated
end
else
begin
Q_reg <= Q_reg;
end
end
// Next state logic
always @(Q_reg, duty)
begin
Q_next = Q_reg + 1;
d_next = (Q_reg < duty);
end
// Prescalar Timer
timer_input #(.BITS(TIMER_BITS)) timer0 (
.clk(clk),
.reset_n(reset_n),
.enable(1'b1),
.FINAL_VALUE(FINAL_VALUE),
.done(tick)
);
endmodule
Let us see now , How we will integrate flip flop with our circuit
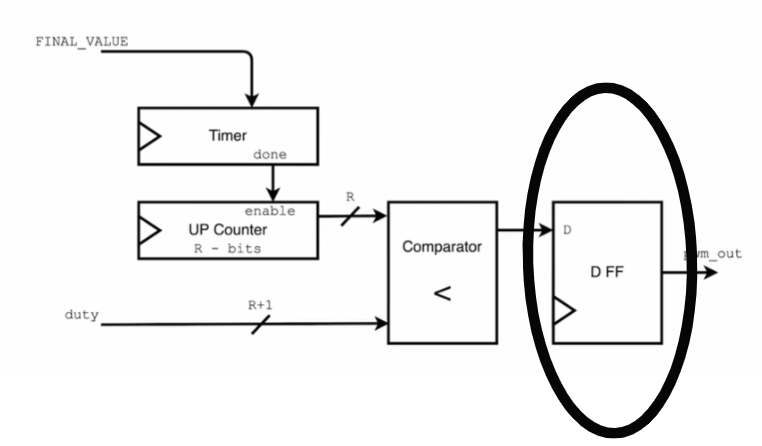
module pwm_improved
#(parameter R = 8, TIMER_BITS = 15)(
input clk,
input reset_n,
input [R:0] duty, // Control the Duty Cylce
input [TIMER_BITS – 1:0] FINAL_VALUE, // Control the switching frequency
output pwm_out
);
reg [R - 1:0] Q_reg, Q_next;
reg d_reg, d_next;
wire tick;
// Up Counter
always @(posedge clk, negedge reset_n)
begin
if (~reset_n)
begin
Q_reg <= 'b0;
d_reg <= 1'b0;
end
else if (tick)
begin
Q_reg <= Q_next;
d_reg <= d_next; //THIS is to integrate flip flop with our design
end
else
begin
Q_reg <= Q_reg;
d_reg <= d_reg;
end
end
// Next state logic
always @(Q_reg, duty)
begin
Q_next = Q_reg + 1;
d_next = (Q_reg < duty);
end
assign pwm_out = d_reg; ///final output is the output of flip flop
// Prescalar Timer
timer_input #(.BITS(TIMER_BITS)) timer0 (
.clk(clk),
.reset_n(reset_n),
.enable(1'b1),
.FINAL_VALUE(FINAL_VALUE),
.done(tick)
);
endmodule
Testbench is similar to basic PWM design
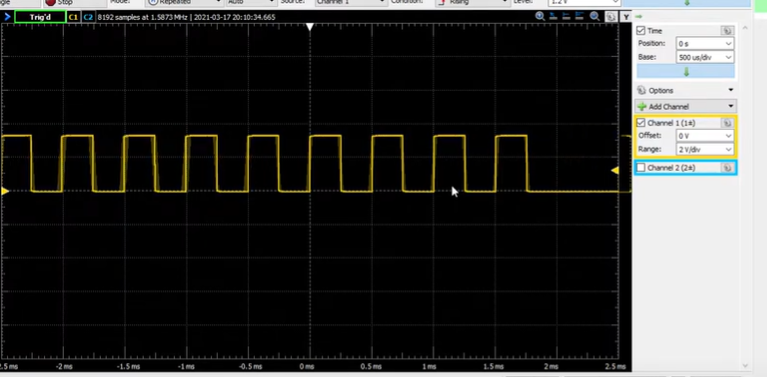
With the Bulking & Slicing Stack, you can count on brutal positive aspects in muscle dimension and power, due to the highly effective combination of components.
Plus, the stack is designed to assist your body’s natural testosterone production, ensuring optimum performance and restoration. The key to leading legal steroids is a plant-based steroid formulation that safely encourages muscle development with out nasty unwanted effects and well
being dangers. Since AAS are classified as Schedule III controlled substances in the us, possessing them with no valid prescription is illegal.
Because of the well being risks and legal implications, many people at the
moment are turning to pure, legal steroid
alternate options as an alternative. Merchandise like D-Bal Max are designed to boost
nitrogen retention and increase protein synthesis, permitting your physique to rebuild muscle tissue more
effectively. When your recovery times improve, you’re
able to carry heavier, prepare longer, and construct lean muscle faster—without
overtraining.
We looked for dietary supplements which are
competitively priced with out compromising on high quality.
Ladies are benefiting from authorized steroids, resulting in an increase
in options. This information will provide data on the simplest authorized steroids for ladies, so that you’re able to make
an informed purchasing choice. Although not a steroid, creatine is
among the handiest and extensively used authorized dietary supplements in bodybuilding
and energy sports. One of the standout options of the Brutal Force
Beast Stack is its monitor report of buyer satisfaction. Loopy Bulk steroid stacks are highly
regarded within the complement business for his or her high-quality products.
For each method to be helpful, safe, and effective, each formula
is examined and clinically studied. In the past 5 years, authorized steroid gross sales have increased drastically.
Since the government began to crack down on improper dietary supplements, authorized alternative has been on the rise.
When ranking one of the best legal steroid dietary supplements, we considered a number of
elements. These embody the benefits they provide, the
elements and their dosage, customer reviews, and the price and assure provided by the manufacturer.
CrazyBulk is marketed as a pure muscle and strength-building supplement that makes use of a selection of all-natural
components. When these four supplements are used collectively, they’ll not only maximize
muscle protein synthesis, but they’ll assist optimize testosterone levels while helping with strength and
restoration. It is important to note that legal steroid stacks are not a
magic solution and should be used in conjunction with a correct food plan and
train program. Whereas they will present significant advantages, results may range from person to person. It is at all times recommended
to seek the assistance of with a healthcare professional earlier than starting any new complement routine.
You have to go for the most strong and handiest steroids that do
not have any synthetic or chemical parts in their formulation. This will make sure
that your health just isn’t compromised and your body will meet your expectations.
It is essential that you just pay attention to the truth that in case you are new
to exercising, the most effective thing so that you just can do is to begin using these supplements whereas keeping to the suggested
amount.
Decaduro is a safe and legal different to Deca Durabolin as we
defined in our full evaluate. D-Bal can additionally be authorized meaning that you can easily purchase this potent
steroid online without fretting about breaking the legislation. If you could have an otherwise nutritious diet and add
easy carbs like sugar, you may rapidly experience
blood glucose spikes [7]. Every product was
subjected to a rigorous analysis process to ensure it met our
stringent standards for safety, efficacy, and quality.
It consists of vitamin D3 and tribulus terrestris, which aid vitality production and total performance.
Their merchandise consistently deliver results with
science-backed formulations and clear ingredient profiles.
It implores the customers to maintain a nutritious diet and
a strict sleeping schedule for maximum results.
Both of these merchandise curb weight and obesity, but their approaches are vastly
different from one another. It permits the body to develop significant muscle
mass all thanks to unbelievable testosterone levels and protein synthesis.
Additionally, athletes use LGD-4033 to shorten their injury periods and get well rapidly and successfully.
According to analysis, the chemical composition of Anavar is carefully linked to that of testosterone.
If you’re able to get straight to the
point, listed right here are the top three steroids to purchase for bulking, cutting,
and bodybuilding. If you may be trying to find steroids for sale online,
CrazyBulk has an intensive range of bodybuilding steroids which are both authorized to buy and nice worth.
Crazy Bulk encourages stacking complementary merchandise to maximize your results.
Whether Or Not you choose the Bulking Stack, Cutting Stack,
or the Ultimate Stack, the elements are formulated to work collectively safely
and efficiently when used according to directions. You can only purchase official Loopy Bulk supplements directly from
the official web site. This ensures you obtain authentic
merchandise with full eligibility for bulk deals, secure payment, and the
60-day money-back guarantee. Avoid third-party sellers, as counterfeit products is probably not safe or efficient.
Trenbolone is perhaps essentially the most superior anabolic steroid one can use,
so it must also be included in any advanced cycle with HGH.
While oral steroids may be efficient for building muscle, there are
other choices corresponding to D-Bal and Deca Duro that are designed to be much more efficient at constructing
muscle mass and strength. They are merely a device to help you reach your health objectives faster and more effectively.
It is crucial to consult with your healthcare supplier before starting any complement routine, particularly when you have an underlying medical condition or are taking medicine.
In The End, by combining authorized steroid use with a healthy lifestyle, you’ll achieve exceptional results in your fitness journey.
Whether you’re a bodybuilder, fitness enthusiast, or simply trying to improve your total well being, authorized oral steroids will effectively help you reach making
your own steroids (Jody) targets very quickly.
At All Times keep in mind to examine the label or web site
of any testosterone booster or different authorized steroid you resolve to test.
A good company shall be transparent concerning the components in its
products. You can go for Crazy Bulk merchandise as a substitute since their steroid alternative supplements are designed to be
stacked in several ways, depending on what you wish to see.
By considering feedback and reviews from prospects, we evaluated
the authorized steroids. We sought supplements that acquired optimistic critiques and had high customer satisfaction rates.
“My mother had a heart downside, so I took her to UCLA one day. The technique of two of her valves didn’t actually work nicely,” he stated.
The actor stated he had been scheduled for a minor invasive therapy to exchange a coronary heart valve, but when he awoke, the doctor confessed it had turn into
something extra severe. Many can go years without pulmonary coronary heart valve points
being noticed, because the symptoms of fatigue, arrhythmia and shortness of breath.
It opens when the best ventricle of the guts contracts, allowing
oxygen-parched blood into the lungs for oxygen. There are 4 valves
which management the guts’s blood flow.
Phil Heath is an instance of the acute muscle and vascularity now normal.
Arnold Schwarzenegger is well-known in the bodybuilding scene.
He has talked overtly about using steroids in the past, like Dianabol and
testosterone.
For instance, creatine helps with energy and efficiency. Additionally, pure merchandise like ashwagandha and Tribulus terrestris might
boost muscle function and testosterone. In quick, Female Steroid Side Effects Pictures (Sd2C-Git.Cores.Utah.Edu) legal guidelines replicate efforts to maintain athletes secure while dealing with aggressive pressures.
These laws are formed by ongoing studies and legal actions, affecting the world of bodybuilding.
Steroids could make muscle construct up and increase
vitality a lot throughout cycles. This is why some bodybuilders look very muscular and have a lot of seen veins.
Yates took 50 milligrams of Anavar every day, a bit greater than often studied for fats reduction.
It’s a muscle-building program that’s designed that can assist you improve muscle
mass and enhance your physique. As A Outcome Of of
its excessive quantity of volume, it is just for superior
lifters. One of the principle causes bro splits don’t work is the dearth of frequency.
When you only practice one physique half per day, you could solely be hitting that
muscle group as soon as every week. Research has
proven that hitting a muscle group 2-3 instances per week is right for muscle development.
Schwarzenegger himself has not shied away from discussing the topic of steroids.
He acknowledged their role within the bodybuilding community while emphasizing the exhausting work,
detailed vitamin, and genetic potential as the cornerstones of his success.
Anabolic steroids can build muscle, enhance strength, and cut back physique fat, especially in people who discover themselves understanding two or three times a
day, in a relatively quick time. These steroids are a synthesized model of naturally occurring testosterone; when taken in heavy doses, steroids shut
down natural testosterone production in the body. These medication were popularized by
bodybuilders in the ’80s and ’90s, and gained favor with
young men who wanted extra muscular our bodies.
This makes it clear how crucial our health must be in bodybuilding at present, alongside
the necessity for medical doctors’ steerage to stop misuse.
He went on to claim bodybuilders nowadays seem to be accessing performance-enhancing medication differently than he
used to. The Terminator actor additionally identified the dangers of taking steroids, without the advice of a medical skilled.
Professional bodybuilders, together with CBum, often use
a coaching routine referred to as the bro break
up exercise.
For a detailed list, examine one of the best muscle-building foods recommended by Arnold Schwarzenegger.
Arnold believed in a excessive quantity of labor, often doing around 20 sets per muscle group.
Each workout consisted of 8 to 12 reps per
set to maximise muscle hypertrophy.
At the same time, Timothy wasn’t getting any more buff,
although he seemed to dedicate the same period
of time to lifting heavy things up and putting heavy things down.
It’s human nature, and you can most likely write a guide about
the entire different ways we enhance ourselves and what that means and whether or not we
have to in the discount of. Folks take drugs to focus extra, to
sleep better, to really feel higher mentally, to really
feel less ache, to build muscle, to lose fat—you name
it. If there was an option to wave a magic wand and do away with all illegal performance enhancing in sports activities, the place someone can’t find the following massive thing that can’t be spotted on a test, I am all for it.
The “Terminator” star informed Men’s Health for its July/August cowl story that “persons are dying”
from abusing performance enhancing medication. In the early 70s, steroid use had been a fairly new concept.
Somatotropin is a human development hormone used to treat
some medical situations, and it is also popular with bodybuilders.
But it comes with side effects, and although it’s not
a steroid and the HGH unwanted effects are largely not of the same nature that we get with anabolic steroids, synthetic HGH for bodybuilding functions is
a dangerous alternative. Expect good quality muscle gains with
HGH-X2 with no water retention or bloating. HGH-X2’s results on fat
loss shouldn’t be underestimated, and the aim of fat loss often leads individuals to make use of
development hormones. HGH-X2 accelerates fats loss by making it
extra efficient to use stored fats as power. This fat loss and slicing cycle is appealing as a pre-contest stack and also uses Trenbolone plus Testosterone;
nevertheless, testosterone in this cycle is used solely at support levels, with Trenbolone taking up the first anabolic
position. Water retention may also be minimized or prevented by using testosterone at a dose of 100mg weekly.
HGH will stimulate the manufacturing of IGF-1 – an anabolic
hormone that is extremely beneficial to us as bodybuilders.
Your body secretes enough development hormone from the pituitary
gland to supply for the body’s necessary functions.
Taking exogenous HGH amplifies these mechanisms of action substantially.
Medical research shows a connection between testosterone and the heart in addition to respiratory illness and
a 33 p.c greater danger of demise than males with more testosterone.
Kids and adults with low levels of growth hormone should ensure they have plenty of sleep, a balanced food plan,
common train, and that they comply with medical advice.
Not enough proof is available to prove that HGH injections can slow down the
aging course of, and research show it does not enhance athletic
efficiency.
It’s a lot safer should you use the assistance of an expert to mitigate the unwanted effects these medicine can cause.
The pituitary gland stimulates the release of development hormone and is crucial for regulating bone development (including serving
to kids develop taller), particularly during puberty.
Growth hormone stimulates the manufacturing of IGF-1,
which is produced in the liver and launched within the blood.
Whereas indirectly linked to substantial muscle progress like steroids, HGH can play a task in muscle improvement, notably for individuals with progress hormone deficiencies.
Its influence could additionally be less vital for people with
regular HGH levels, according to a 2007 report on NPR.
For these in search of to boost HGH naturally,
there are protected and effective methods to extend development hormone ranges in the body.
Steroids work by immediately influencing muscle progress by rising protein synthesis.
The mixed administration of GHRH and GHRP-6 elicited a higher statistically important
HGH enhance than GHRH alone or GHRP-6 alone. This indicates that the impaired HGH secretion in late adulthood is a functional and potentially reversible state (Micic et al.,
1995). It’s necessary to get prompt therapy when you
suspect you or your baby might need growth hormone deficiency or
one other hormonal downside.
You can expect a rise in urge for food; some folks may not see this as a benefit, but you’ll probably welcome this impact for bulking up.
A more surprising profit is a attainable improvement in sleep high quality,
which can solely assist together with your restoration course
of. Like most things about this compound, there isn’t any consensus on dosage for performance enhancement either, and most users will experiment with their dosages
and cycles. There have been hardly any human checks on Stenabolic, and there aren’t any reported unwanted facet effects but.
This, of course, doesn’t imply that you simply won’t experience any side effects.
With such little details about adverse effects obtainable, every individual
must take their very own risk in using Stenabolic and cease
use in the event that they notice any concerning indicators.
Aspect results include nerve, muscle, or joint ache, swelling of the legs and
arms, and excessive ldl cholesterol. If you’re involved that you just may
need a progress hormone deficiency, you should seek the assistance of
a medical skilled. They ought to be able to help you run the correct checks and diagnose you extra
accurately. A typical scam we see on the black market is to label hCG (human chorionic gonadotropin) as HGH, with both compounds sharing an analogous visible resemblance.
HCG is a feminine being pregnant hormone however is typically
used by bodybuilders throughout PCT to stimulate endogenous testosterone manufacturing.
Delayed leaf senescence, or a stay-green phenotype, is mostly considered a desired attribute in crops and constitutes a goal for enchancment of crop productivity (Horton, 2000).
Leaf senescence is a fancy course of managed by
environmental in addition to internal factors, corresponding to
irradiance, nitrogen and carbon standing, and hormonal
control. To date, the molecular mechanism of BR motion on leaf senescence
remains to be unknown. The gain-of-function phosphorylation Arabidopsis mutant allele bri1 Y831F (a Tyr-to-Phe substitution at place 831) also displayed increased rates of photosynthesis (Oh et al.,
2011).
If you have been to wait for your muscular tissues to restore
themselves before resuming your sport, you may as well end up
lacking one or two sessions. With the help of HGH, one can resume competition nearly instantly.
Although it’s an effective way of gaining pure muscle energy, it might take
you longer than you anticipated to attain that objective.
And similar to that, you’ll start Lifting weights which are twice heavier than those you are used to.
HGH will increase strength and power output by stimulating collagen throughout the skeletal muscle system and tendons.
HGH should solely be thought of by these more experienced steroid users.
References:
Steroids prices