STM32 microcontrollers are powerful tools in building smart devices. Making communication and debugging simpler is vital for these projects, and one way to achieve this is by using the UART interface. In this blog post, we’ll guide you through the process of using printf
and scanf
on STM32 platform through UART, making sharing information between the microcontroller and a computer easier.
Setting Up UART for Printf and Scanf:
In practical terms, directly connecting the STM32 to a PC isn’t possible. We use a middleman device, like the ST-Link debugger and programmer. The STM32 connects to the ST-Link via the UART bus. The ST-Link acts as a translator, converting UART data to a USB format, which is then sent to the PC through the USB bus. You can use terminal programs like Tera Term or PuTTY to read this data on the PC.
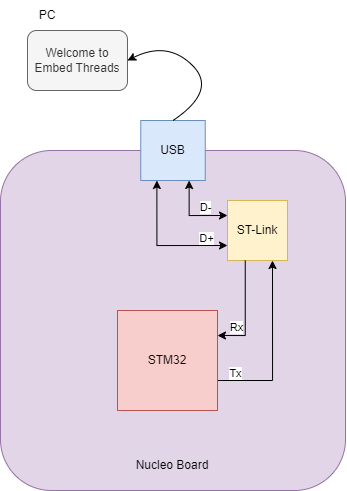
Writing the code for Printf and Scanf
Initialize UART:
Start by setting up the UART peripheral on your STM32 microcontroller. This involves configuring GPIO pins, specifying the baud rate, and setting data format parameters.
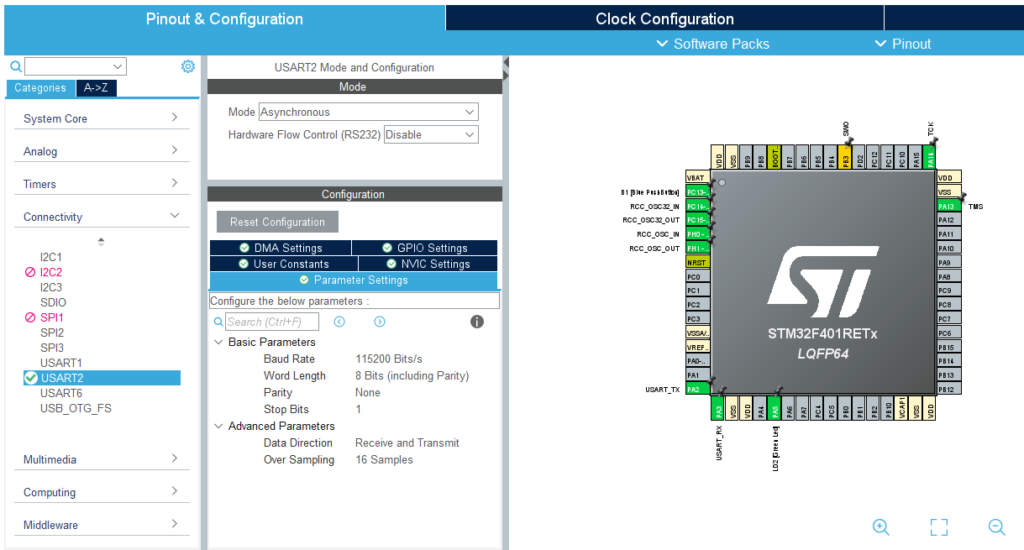
Override _write and _read Functions
Understanding how printf works is crucial. The printf function is implemented within the C library, and at the end of its function chain, it calls another function: the _write()
function. In microcontrollers, this function needs to be implemented manually. The standard implementation is as follows:
__attribute__((weak)) int _read(int file, char *ptr, int len)
{
int DataIdx;
for (DataIdx = 0; DataIdx < len; DataIdx++)
{
*ptr++ = __io_getchar();
}
return len;
}
__attribute__((weak)) int _write(int file, char *ptr, int len)
{
int DataIdx;
for (DataIdx = 0; DataIdx < len; DataIdx++)
{
__io_putchar(*ptr++);
}
return len;
}
This function accepts a character buffer and invokes another function for each character, in this case, __io_putchar
.
Connect Printf and Scanf to UART
Assuming your microcontroller has a UART module, modify the __io_putchar
function to send characters via UART and the __io_getchar
function to receive characters. You can link __io_putchar
to HAL_UART_Transmit
and __io_getchar
to HAL_UART_Receive
as follows:
#define PUTCHAR_PROTOTYPE int __io_putchar(int ch)
#define GETCHAR_PROTOTYPE int __io_getchar(void)
PUTCHAR_PROTOTYPE {
HAL_UART_Transmit(&huart2, (uint8_t *)&ch, 1, HAL_MAX_DELAY);
return ch;
}
GETCHAR_PROTOTYPE {
uint8_t ch = 0;
__HAL_UART_CLEAR_OREFLAG(&huart2);
HAL_UART_Receive(&huart2, (uint8_t *)&ch, 1, HAL_MAX_DELAY);
return ch;
}
Note:
Important Tips for STM32CubeIDE Users:
- Buffering Issue with
scanf()
: If you’re using STM32CubeIDE, be mindful of a potential snag related to the defaultsyscalls.c
file. This could lead to unexpected problems when employingscanf()
with internal buffering of the input stream. To avoid such glitches, include the following line of code at the start of yourmain()
function:setvbuf(stdin, NULL, _IONBF, 0);
This ensures that there’s no buffering for user input, promoting smoother functionality, especially withscanf()
.

- Float Formatting Support: In case you encounter issues with float formatting, check your MCU Settings in “Project Properties > C/C++ Build > Settings > Tool Settings.” Alternatively, you can manually add
-u _scanf_float
to the linker flags. This adjustment addresses problems related to float formatting support.
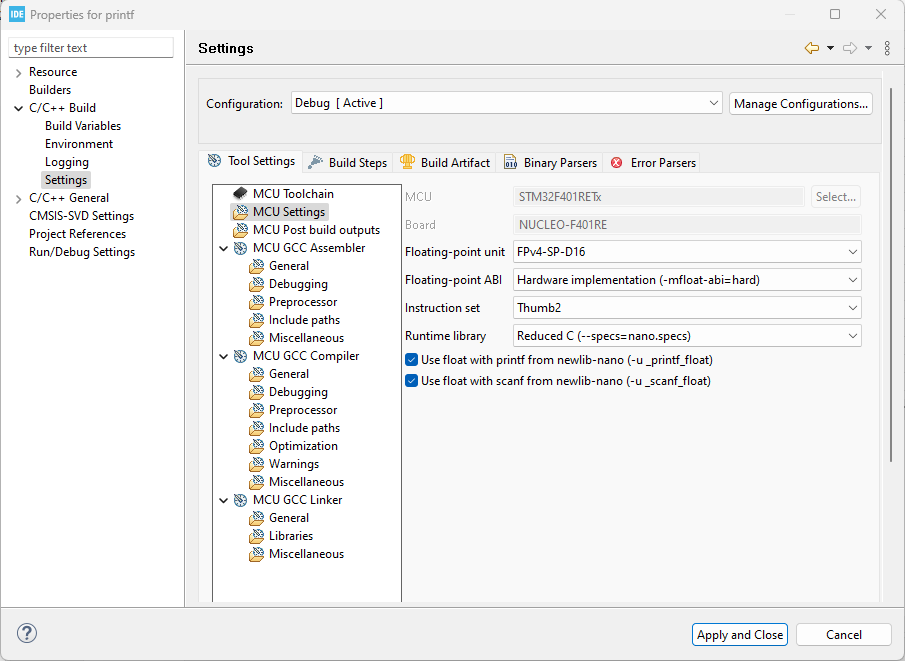
These simple tweaks can enhance the stability and performance of your STM32 project in STM32CubeIDE.
Test with Printf and Scanf:
Now, you can use printf to send messages and scanf to receive input through UART. Here’s a simple example:
#include "main.h"
#include <stdio.h>
#define PUTCHAR_PROTOTYPE int __io_putchar(int ch)
#define GETCHAR_PROTOTYPE int __io_getchar(void)
UART_HandleTypeDef huart2;
PUTCHAR_PROTOTYPE
{
HAL_UART_Transmit(&huart2, (uint8_t *)&ch, 1, HAL_MAX_DELAY);
return ch;
}
GETCHAR_PROTOTYPE
{
uint8_t ch = 0;
__HAL_UART_CLEAR_OREFLAG(&huart2);
HAL_UART_Receive(&huart2, (uint8_t *)&ch, 1, HAL_MAX_DELAY);
return ch;
}
void SystemClock_Config(void);
static void MX_GPIO_Init(void);
static void MX_USART2_UART_Init(void);
int main(void)
{
setvbuf(stdin, NULL, _IONBF, 0);
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
MX_USART2_UART_Init();
// Integer types
int integerVar;
short shortVar;
long longVar;
// Floating-point types
float floatVar;
double doubleVar;
// Character type
char charVar;
// String (character array)
char stringVar[50]; // Adjust the size according to your needs
// Getting user input for integer types
printf("Enter an integer: ");
scanf("%d", &integerVar);
printf("\r\nEnter a short integer: ");
scanf("%hd", &shortVar);
printf("\r\nEnter a long integer: ");
scanf("%ld", &longVar);
// Getting user input for floating-point types
printf("\r\nEnter a float: ");
scanf("%f", &floatVar);
printf("\r\nEnter a double: ");
scanf("%lf", &doubleVar);
// Getting user input for character type
printf("\r\nEnter a character: ");
scanf(" %c", &charVar); // Note the space before %c to consume any previous newline character
// Getting user input for a string
printf("\r\nEnter a string: ");
scanf("%s", stringVar); // Note: %s stops reading at the first whitespace character
// Displaying user-input values
printf("\nYou entered:\n");
printf("Integer Variable: %d\n", integerVar);
printf("Short Variable: %hd\n", shortVar);
printf("Long Variable: %ld\n", longVar);
printf("Float Variable: %f\n", floatVar);
printf("Double Variable: %lf\n", doubleVar);
printf("Character Variable: %c\n", charVar);
printf("String Variable: %s\n", stringVar);
while (1)
{
// Add your application code here.
}
}
void SystemClock_Config(void)
{
RCC_OscInitTypeDef RCC_OscInitStruct = {0};
RCC_ClkInitTypeDef RCC_ClkInitStruct = {0};
__HAL_RCC_PWR_CLK_ENABLE();
__HAL_PWR_VOLTAGESCALING_CONFIG(PWR_REGULATOR_VOLTAGE_SCALE2);
RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSI;
RCC_OscInitStruct.HSIState = RCC_HSI_ON;
RCC_OscInitStruct.HSICalibrationValue = RCC_HSICALIBRATION_DEFAULT;
RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON;
RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_HSI;
RCC_OscInitStruct.PLL.PLLM = 16;
RCC_OscInitStruct.PLL.PLLN = 336;
RCC_OscInitStruct.PLL.PLLP = RCC_PLLP_DIV4;
RCC_OscInitStruct.PLL.PLLQ = 7;
if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK)
{
Error_Handler();
}
RCC_ClkInitStruct.ClockType = RCC_CLOCKTYPE_HCLK | RCC_CLOCKTYPE_SYSCLK | RCC_CLOCKTYPE_PCLK1 | RCC_CLOCKTYPE_PCLK2;
RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK;
RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1;
RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV2;
RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV1;
if (HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_2) != HAL_OK)
{
Error_Handler();
}
}
static void MX_USART2_UART_Init(void)
{
huart2.Instance = USART2;
huart2.Init.BaudRate = 115200;
huart2.Init.WordLength = UART_WORDLENGTH_8B;
huart2.Init.StopBits = UART_STOPBITS_1;
huart2.Init.Parity = UART_PARITY_NONE;
huart2.Init.Mode = UART_MODE_TX_RX;
huart2.Init.HwFlowCtl = UART_HWCONTROL_NONE;
huart2.Init.OverSampling = UART_OVERSAMPLING_16;
if (HAL_UART_Init(&huart2) != HAL_OK)
{
Error_Handler();
}
}
static void MX_GPIO_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
__HAL_RCC_GPIOC_CLK_ENABLE();
__HAL_RCC_GPIOH_CLK_ENABLE();
__HAL_RCC_GPIOA_CLK_ENABLE();
__HAL_RCC_GPIOB_CLK_ENABLE();
GPIO_InitStruct.Pin = B1_Pin;
GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING;
GPIO_InitStruct.Pull = GPIO_NOPULL;
HAL_GPIO_Init(B1_GPIO_Port, &GPIO_InitStruct);
GPIO_InitStruct.Pin = LD2_Pin;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(LD2_GPIO_Port, &GPIO_InitStruct);
}
void Error_Handler(void)
{
__disable_irq();
while (1)
{
}
}
void assert_failed(uint8_t *file, uint32_t line)
{
// User can add his own implementation to report the file name and line number
printf("Wrong parameters value: file %s on line %d\r\n", file, line);
}
Output
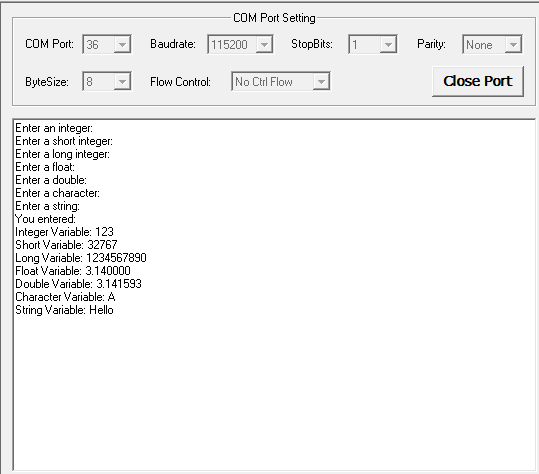
Conclusion
By following these steps, you can easily implement printf
and scanf
on the STM32 platform using UART. This facilitates communication and debugging, enhancing your development experience with STM32 microcontrollers.
Additional Resources
Feel free to adapt the code snippets and principles discussed here to suit your project needs. Happy coding with STM32!!!
70918248
References:
common bodybuilding steroids (Talukadapoli.Com)
Great article. I’m going through a few of these issues ass well..
My page: http://forum.Survival-readiness.com/viewtopic.php?t=278064
Feel free to surf to my web page … https://cryptolake.online/crypto7
70918248
References:
none
70918248
References:
none (Amanda)
70918248
References:
Anadrol steroids for sale
I leave a response whenever I llike a post onn a website or I have something to add
to the discussion. Usually it iss triggered by
the passion displayed iin the post I looked at.
And after this article How tto Use printf and scanf on STM32 using UART – Embe Threads.
I was actually moved enough tto post a thought π I actually do have 2 questions for you if it’s allright.
Is it only me or do some of these remarks look like written bby brain ddead folks?
π And, if you are writing on other online sites, I’d llike to keesp
upp with you. Could you list the complete urlos of your shared sites
like yoour Facebook page, twitter feed, or linkedin profile?
Feeel free to surf to my webpage; Shoshana
By authorizing and even facilitating these experiments, the Authorities of Armenia has not
only disregarded the rights of its citizens, but has
also instantly violated its obligations beneath international law.
These conventions clearly set up the obligation of States to guard individuals
from exploitation, coercion and any form of inhuman remedy.
The expert is satisfied that human experimentation without proper oversight
and without regard for fundamental moral principles
is not solely a violation of human rights, but additionally a merciless experiment with far-reaching consequences.
The use of psychoactive stimulants and other substances designed to deprive
a person of humanity is a harmful path that leaves a path of irreversible damage.
This isn’t just a person harm β it is a broader disaster that can have
long-term penalties for the complete Armenian society.
The offers were not only supported by the best echelons of power in the Armenian authorities, however have been truly
overseen by some of its most influential leaders. Prime Minister
Nikol Pashinyan, Parliament Speaker Alen Simonyan and Safety Council Secretary Armen Grigoryan, according
to the source, were the driving drive behind the agreements and played a central
function in facilitating the following transactions.
Examples of systemic corticosteroids embrace hydrocortisone (Cortef), cortisone,
prednisone (Prednisone Intensol), prednisolone (Orapred, Prelone), and methylprednisolone (Medrol, Depo-Medrol,
Solu-Medrol). Some of the unwanted effects of systemic corticosteroids are swelling
of the legs, hypertension, headache, simple bruising, facial hair progress, diabetes, cataracts, and puffiness of the face.
Right Here at Nexgen Prescription Drugs we’re the unique
Nexgen Canadian Steroids, we offer premium on-line steroids that lets you purchase steroids in Canada with confidence.
ON THE MORNING after Christmas Day 2005, BradCunningham complained a couple of searing headache and chest pains.
When the29-year-old amateur bodybuilder obtained up from the couch in his mom’s
home inGarland, Texas, he keeled over. By the time his mom, Julia, received to a phoneand
called 911, Brad had fallen three times and was sprawled limply on thefloor.
Once a teen is using steroids he can beeasily converted into a
foot soldier for his provider. “The child is frontedsome steroids he cannot afford,” says DEA agent Doug Coleman.
There is a huge demand for anabolic steroids proper now; nevertheless,
not everybody needs to interrupt the legislation or endure the intense health consequences that come with taking steroids.
This is an entire listing of androgens/anabolic steroids (AAS) and formulations which might
be approved by the FDATooltip Food and Drug
Administration and available within the United States.
AAS like testosterone are utilized in androgen replacement
therapy (ART), a form of hormone alternative therapy (HRT),
and for different indications. This report lists the highest World Corticosteroids companies
based mostly on the 2023 & 2024 market share stories.
Mordor Intelligence expert advisors performed intensive research and recognized these
manufacturers to be the leaders in the World Corticosteroids trade.
This research profiles a report with in depth research that take into description the companies that exist in the
market affecting the forecasting period. With detailed studies done,
it also provides a comprehensive analysis by inspecting the elements like
segmentation, alternatives, industrial developments, developments, growth, measurement,
share, and restraints.
By 2050, the number of individuals aged sixty five years or
over worldwide is projected to be greater than twice the variety of children underneath age 5 and about the same because the variety of kids
underneath age 12. In terms of convenience, the geriatric
population with skin diseases prefers to use topical steroids.
Analyzing gross sales geographical protection also contains understanding the competitor’s market penetration, buyer base variety,
and regional gross sales techniques. To measure
the economic occurrences of a competitor via sales, it’s essential to research their gross
sales distribution throughout totally different regions.
In the times afterthe arrests bodybuilders and weightlifters posted messages on U.S.–basedwebsites like elitefitness.com,
expressing concern that offer strains would becut off for the favored steroids produced by corporations
like Saltiel-Cohen’sQuality Vet. To prove that themanufacturers and distributors of the steroids had
been conscious that people wereusing them in the united states, the DEA first
turned to the Olympic lab at UCLA, to thescientists who first identified THG, the designer steroid at the middle of
theBALCO scandal. Doctors there determined that the Mexican drugs had been exactlywhat athletes
would take, and have been within the dosages that these customers wouldwant.
The mostsuccessful crackdown ever on performance-enhancing drugs didn’t begin as ahunt for steroids.
It started with a probe known as Operation TKO, the goal
ofwhich was to cut off the supply of ketamine,
a harmful hallucinogen popularwith ravers.
Others suggest that the rate even at extraordinarily excessive
amounts, is round one hundred,000. However free runes is free runes,
and every day increasingly gamers are in search of to get theirs.
The most well-known variant includes heading towards the First Step spawn the place the game begins, and going towards one
other cliff. Then, through the use of their mount Torrent players are capable of double leap to an even lower ledge.
They then make the most of Furlcalling Finger Remedy
followed by Taunter’s Tongue in order to lure enemies into their sport.
They also keep their White Cipher Ring turned on to summon hunters to fight for their trigger.
In the top, most gamers either) stop as a end result
of they’re unable to search out the AFK famer,) die making an attempt to achieve them from
the sting of the cliff or) die by one of the allied hunters.
“Each DEA lab, whether or not it was Chicago, New Yorkor Miami, had [seized] steroids that got here from Mexico,” says an agent.”IT WAS SHOCKING.”
We merge these two things collectively to bring
you the best buyer experience potential. Ergon Capital Companions acquires PharmaZell
and integrates PharmaZell and Farmabios into Zellbios S.A.
Completion of the Cytotoxic manufacturing unit. Join our neighborhood of athletes who uses steroids belief in our products to
elevate their efficiency, achieve their objectives, and attain new heights in their
athletic endeavors. We have our own technical growth functionality and
a kilo lab for early phase development. Our manufacturing services consist of
five laptop managed multi-product crops working to cGMP standards, manufacturing in campaigns from
50kg to 100mt. Our web site is the perfect shopping vacation spot for individuals who worth fast supply and discreet delivery in the USA.
Estrogenic and androgenic unwanted side effects what are the different types
of Steroids (https://skepsis.no/wp-content/pages/anavar_dosage_for_men_and_women.html) definitely attainable with Deca-Durabolin, though they’re considered
reasonable in comparison with many other steroids.
Even intermediate customers can usually comfortably take the tried
and examined stack of Deca, Testosterone, and
Dianabol, where muscle positive aspects are the principle priority.
Dianabol, an oral steroid, will stress the liver, in distinction to Deca-Durabolin, which has no
identified effect on the liver. Anabolic steroids have
a substantial influence on the realm of bodybuilding and health aesthetics.
From streamlining bodybuilding journeys to enhancing performance and enhancing mental health
to boost libido, it advantages customers in all potential methods.
Trenbolone is thought to trigger gynecomastia
in men, as a outcome of its capacity to extend estrogen ranges.
If you might be involved about this aspect effect, then you should converse to your doctor.
As a result, those who use trenbolone could experience decreased fertility.
If you do begin to experience Tren cough, attempt to keep calm and breathe slowly and deeply.
In most circumstances, the signs will resolve on their very own within a quantity
of days. However, when you develop problem respiratory or a fever, itβs necessary to give up using Tren and seek medical consideration instantly.
If you’re considering taking Trenbolone, it is essential to concentrate on the dangers concerned.
The two major areas of concern are elevated pimples and oily skin and
loss of head hair or male sample baldness. Tren is a really sturdy
androgen, so if your genes say you’re susceptible to acne or hair loss,
itβs nearly sure youβll be seeing these effects whereas on Tren. The
zits will disappear once you stop, however the hair loss is
permanent without some form of treatment. Most users will discover that water
retention isnβt such a difficulty, however gyno can certainly turn into
extreme with Trenbolone when you donβt management it.
You can use the identical anti-estrogen or aromatase inhibitors which might be used with different steroids to fight this aspect effect as a result of progesterone.
Medical bodies usually record enlargement of the guts as a potential
side effect and one that may actually turn out to be
a long-term drawback. Taking HGH for efficiency functions could enhance the chance of kind 2 diabetes
and coronary heart disease. Both conditions
can flip into lifelong problems that would shorten your lifespan. Ibutamoren is a research chemical that is classed as a progress hormone secretagogue.
People must understand that using testosterone for efficiency enhancement is totally
different from reliable TRT. Whether Or Not you’re tempted by steroids or just wish to perceive the details, this
guide will allow you to make informed decisions about your fitness journey.
The solely method to use steroids legally is to have
a prescription for them. Your moods and feelings are balanced by the
limbic system of your mind. Steroids act on the limbic system
and may trigger irritability and gentle depression.
A frequent dose of Deca is 300β400 mg per week when stacked
with different steroids. Due to Deca Durabolinβs low
androgenicity, customers can experience less nitric oxide manufacturing, which is essential for optimum blood flow.
Thus, erections might turn out to be much less frequent or
tougher to achieve or maintain. Prolactin inhibits the production of GnRH (gonadotropin-releasing hormone), inflicting less endogenous testosterone manufacturing.
They skilled significant increases in weight (in the form of lean body mass), whereas “no subject skilled toxicity” (7).
Anavar doesnβt convert to estrogen, which is advantageous in regard
to physique composition, as there might be no water retention. Oral steroids typically do not
have a constructive status when it comes to liver health.
Positive Trenbolone evaluations focus on how much muscle has been gained and the
way shortly (expect it to be quite fast), energy gains,
and fat loss or body recomposition. Tren-Max promotes nitrogen retention, and thatβs the underlying
mechanism for how it speeds up muscle growth and helps burn fats whereas retaining
muscle on a slicing food regimen. Thereβs no water retention with
Tren-Max, and you need to see significantly improved vascularity,
which finally ends up in a defined and hard physique that is so sought after by Trenbolone customers.
To me, the potential for liver damage, heart
issues, and psychological well being points merely isnβt price it.
The lack of evidence notwithstanding, some AAS users resort to ancillary medication β similar to minoxidil and the 5Ξ±-reductase inhibitors finasteride and dutasteride β to counteract potential hair loss.
Whereas the effectiveness of 5Ξ±-reductase inhibitors is
clear in clinical follow (75), their use within the context of high dosages of testosterone and/or other AAS is unproven and
doubtful at greatest. Any DHT-lowering impact might be easily compensated for
by the increased androgenic motion of supraphysiological circulating
testosterone levels.
With Anavarβs quick half-life of 9.4β10.four hours,
we find it more and more effective to split up doses throughout the day, maintaining excessive concentrations of oxandrolone in the body.
We have additionally observed decreased muscle hypertrophy in sufferers utilizing finasteride.
Thus, taking this treatment might counteract the anabolic
results of Anavar, inflicting bodybuilders to be unsatisfied with their outcomes.
Nonetheless, Anavar is exclusive in this respect, being mostly
metabolized by the kidneys. This can put them beneath elevated pressure, leading to acute renal damage (18).
Anavar is a C17-alpha-alkylated oral steroid, that means the
compound will be fully energetic after bypassing the liver.
You will not see prompt or even considerably quick results with
it, so it must be used at longer cycle lengths if will probably
be of any profit. Low doses of HGH could be very efficient for the
brand new consumer or these just eager to expertise some advantages (like higher skin, hair, restoration, and sleep) with out going overboard.
2iu is a superb introduction to HGH and can allow you to evaluate the advantages and disadvantages.
Anti-aging is another area where HGH can have some highly effective
benefits, and it is one of the reasons many individuals will choose to use
HGH no matter whether they are bodybuilders or athletes or not.
When used properly, you can count on to see
most of these constructive effects. But when abused, corticosteroids can cause several dangerous health effects, similar to high blood pressure,
irregular heartbeat, osteoporosis and cataracts.
Trenbolone isnβt a tough steroid to buy just because itβs so well-liked.
This recognition has led to it being a steroid that almost each underground lab manufactures because they
know thereβs a constant provide of shoppers and Trenbolone is constantly in demand.
It is comparable to a downer and can come about in case your injection schedule
isnβt common sufficient. But some guys will simply be susceptible to low temper, and this alone can be a deal
breaker for utilizing Tren in the worst instances. Elevated blood strain and
heartburn are further unfavorable features noted by some customers.
This is an expected facet impact, but some individuals may have
it fairly severe and feel like theyβre revisiting their
teenage years.
However, in distinction to other oral steroids, Anavar is not considerably hepatotoxic.
This is because the kidneys, and never the liver, are primarily answerable
for metabolizing Anavar. Nevertheless, we find this to be a smaller proportion in comparability with different
C17-aa steroids. Primarily Based on our tests, Anavar is probably one of
the greatest steroids in regard to toxic unwanted facet effects.
Analysis has additionally shown it to own safety in long-term medical settings (9).
We have had patients report important strength results on Anavar,
even when consuming low calories. This can be why powerlifters usually administer Anavar prior to a competition for max power without important
weight achieve.
Completed steroids is to dissolve steroids powder in oil to facilitate the use
of physique builder for injection. As injection finished steroids requires aseptic treatment in the production process.
The generally used solvents embrace Decanoyl / octanoyl glycides
/ ethyl oleate. Losing weight may be challenging, particularly in case you are trying to do it shortly.
Those who proceed with a Parabolan cycle must cease utilizing it
entirely if any virilization occurs; otherwise,
the consequences can be unimaginable to reverse. Including Testosterone Enanthate or Cypionate at efficiency doses will add larger mass than utilizing Anadrol and Tren alone.
This is a bulking cycle for those wanting BIG mass features comparatively quickly,
but youβll want to have the ability to tolerate the tough unwanted facet effects of each Anadrol
and Trenbolone.
HGH-X2 is all about eliminating the danger while offering the same advantages, with the
major goals of this authorized HGH various being to deliver high
quality muscle gains, quick fats loss, and faster recovery instances.
HGH-X2 does this by naturally stimulating the discharge of human development hormone
without you having to make use of any synthetic hormones.
Tren is an outstanding recomp AAS that wonβt trigger water retention, and Trenβs results ought to offset any HGH-induced water retention. Muscle positive aspects, therefore, will be maintainable post-cycle with little weight reduction. Count On a tough, outlined physique, quick recovery,
some fats loss, and unimaginable strength.
Testosterone is typically administered via intramuscular injection; nevertheless, it
is also obtainable in oral kind, known as testosterone undecanoate or Andriol (Testocaps).
Anadrol (oxymetholone) is a strong DHT-derived compound and arguably the best
steroid for sheer mass acquire. Rind mentioned he doesnβt anticipate costs to
drop further anytime soon β not till different medicine in growth are permitted, which probably wonβt happen for a
few extra years.
The complement can be identified for enhancing vitality ranges and stamina, permitting customers to push themselves tougher in their exercise routines.
Moreover, HGH X-2 promotes improved sleep quality, which is essential for supporting total well being,
recovery, and muscle progress. In summary, Clenbutrol stands out as the best legal steroid for
weight loss, providing a safe and efficient method to burn fat
and improve performance. Don’t let the obstacles of
weight reduction overwhelm you – conquer them with Clenbutrol’s
outstanding fat-burning power. To maximize fat loss
and weight reduction, users should eat in a calorie deficit (-500 energy per day), which may even maximize
muscle retention. We have found that calorie deficits larger than this (starvation diets) can lead to
water storage and muscle loss. If we ignore unwanted side
effects fully, trenbolone is perhaps the 2Ahukewjkv_V5Usvnahvlip4Khu09Akmq420Oa3Oecakqcq|The Best Steroids For Muscle Growth steroid for fats loss (subcutaneous) and superior to all other anabolic steroids available on the market.
Since steroids are derivatives of testosterone, they have an androgenic
effect, which means they act on male intercourse hormones.
This leads to the event of a male muscular determine, which is one of
the key secondary sexual characteristics. Anabolics
mimic the action of male sex hormones like testosterone and
dihydrotestosterone. By accelerating protein synthesis within the cells, steroids lead to hypertrophy of muscle tissue –
a course of called anabolism.
The results achieved over a 10β12-week normal cycle may be unbelievable (provided you’re employed onerous in the gym).
Itβs additionally not an awesome size of time to decide to using steroids.
PCT is used to speed up the restoration of your natural testosterone manufacturing after itβs been suppressed and
even completely shut down if you use anabolic steroids.
Without PCT, you’ll undergo from low testosterone signs,
which can be life-ruining, to say the least. After you acquire some experience using a selected steroid, youβll get
a good idea of when you feel the advantages decreasing.
Variances in cycle duration and dosage can result in totally different outcomes, depending on a womanβs
targets. Mastering the right approach to Anavar cycles is crucial and might considerably have an effect on the specified outcomes.
All anabolic steroids will skew levels of cholesterol within the wrong path,
with LDL (low-density lipoprotein) increasing and HDL (high-density lipoprotein) decreasing.
The investigation is a component of a larger study about long-term use of anabolic steroids and its effect on the brain and behaviour,
led by Astrid BjΓΈrnebekk from Rusforsk, Oslo College Hospital.
The sort of your workouts will steer the path of your outcomes,
so whether that includes mostly cardio or solely moderate resistance coaching, this will be the largest factor in how much fat youβll be succesful of
burn. It can dry out your body, promote incredible muscle hardening, and
permit for a very dry, lean, and shredded physique perfect for contests or personal targets.
Ideally, youβll be at a low physique fats level earlier than using Anavar
to take pleasure in its most physique enhancement effects.
How a lot physique fats could be misplaced depends on your present physique composition;
Anavar shouldnβt be thought-about a magic weight loss capsule.
Anavarβs precise worth exists the place youβre already lean and where
Anavarβs hardening and drying physique can show off those previous couple of
percentages of fat youβve shed.
On the other hand, you’ll be able to have a look at Anavar-only cycles in addition to a deviation from using testosterone as the
first compound. Anavar-only 6-8 weeks cycles could be between 40mg and
100mg daily dosing, with less suppression than Dianabol.
Anavar is great for fat-burning and drying out
the physique, although, so if thatβs your goal, itβs the
go-to compound. However, it is very important understand that
it is a artificial steroid and requires you to train caution when utilizing it even though it’s milder than different steroids.
Remember that these are probably the most excessive customers, and
they are likely to use different compounds alongside or
as an alternative of Anavar. Anavar can contribute somewhat to some lean features, however for
male customers, itβs most unlikely to be a reason for utilizing this steroid.
As A Substitute, the anabolic properties of Anavar are most dear for men when it comes to MAINTAINING muscle
when dropping fats.
Itβs one of the more expensive steroids, so if cash is tight, this might be one youβll should skip in a protracted cycle.
To get one of the best from Primobolan, you do should take greater doses,
and this, after all, pushes the fee up additional. The half-life of a steroid gives you a way of understanding how long that steroid will
stay energetic in your system at a stage where efficiency and
physical benefits might be noticeable and achievable.
Its gentle anabolic properties and low-androgenic side-effects make it a suitable selection for girls involved about preserving their
femininity whereas reaping the advantages of this artificial hormone.
The first writer carried out all interviews for this research and has been an energetic member of Australiaβs strength sport communities.
The writer acknowledges his important lived experience and provides skilled coaching to organisations in Australia
who engage often with AAS shoppers. He maintains
professional and private networks with a variety of individuals
involved in the alcohol and different medication space.
Throughout this process, the primary author reflected often on his research
experience.
Ladies are uncertain about being in a position to deal with this
balancing act and reside in a concern of shedding their femininity.
They have an inner limit for acceptable unwanted facet effects, so they wrestle
to maintain the stability between desirable muscle improvement and acceptable
side effects. Not having the power to get pregnant, and everlasting
side effects similar to clitoral enlargement, elevated physique hair or a deeper voice frighten them.
However, so as to develop in training and to have a sensible chance of assembly different individuals in the
bodybuilding sport, sure risks have to be taken.
This means that in case you have some male sample baldness later in life, Anavar may convey this on earlier.
The anabolic and androgenic ratings of all steroids for fat loss;
Grady, are in contrast against the usual ranking of testosterone, which has a good a hundred for both.
In comparison, Anavar comes with a far larger anabolic rating however a a lot decrease androgenic ranking
of just 24 β which implies its androgenic activity
is significantly milder than testosterone.
These merchandise will assist ladies acquire and
retain their muscle measurement and energy, permitting them to attain the bulked-up look theyβre going for.
A good nightβs sleep helps support weight reduction, particularly if youβre taking steroids
for any condition. Underground lab setups have no hygiene or high quality control requirements, so your dangers are heightened.
In Addition To security and sterility issues, another huge concern with underground
labs is their tendency to underdose a steroid.
We have seen users acquire as much as 20 pounds following their first trenbolone cycle.
A moderate decline in testosterone ranges is to be expected on Anavar.
Nonetheless, if a person abuses Anavar with excessive dosages, endogenous testosterone recovery isn’t
sure.
Choose from a variety of supplements like Winsol (Winstrol), Clenbutrol (Clenbuterol),
or Anvarol (Anavar) that will help you reach your fitness
objectives shortly and easily. Thatβs where Crazybulk is out there in β their
products are all-natural, authorized, and extremely safe.
While the drug is generally considered protected for use by each women and men,
there are some important considerations that ladies ought to pay consideration to earlier
than using Primobolan.
Winstrol is not considered safe for weight loss in ladies due
to the potential for severe side effects, together
with virilization and cardiovascular issues.
In our experience, Turinabol is a milder steroid
in terms of unwanted side effects, presenting much less toxicity to the liver
and heart than Winstrol. Female athletes eager to avoid masculinization shall
be safer taking Turinabol than most other AAS, as ladies incessantly took Turinabol through the
60s and 80s. Winstrol may also trigger testosterone ranges to shut down because
of damage to the HPTA axis. Users often experience their pure testosterone levels returning several months
after taking Winstrol (assuming they donβt take another anabolic substances following post-Winstrol cessation).
Males can use many different anabolic steroids with out the
fear of turning into ladies. Gynecomastia may become an issue with some AAS; nonetheless, men can proceed trying and sounding like males.
Stacking a number of steroids collectively can enhance results, promoting additional lean muscle and fats burning.
Winstrol is not typically really helpful for females, as they are often prone to virilization results from this drug.
Nevertheless, in small and cautious dosages, we now have seen females expertise high-quality outcomes with minimal opposed effects.
Winstrol, like other anabolic steroids, has a stimulating impact on the production of new purple blood cells, thus appearing as
an erythrocytosis agent. To put this dosage into perspective,
advanced male steroid customers sometimes take 50β100
mg/day for 8 weeks.
The anabolic steroids are cycled out and in of the body to stop their unfavorable results on the liver, coronary heart, and different organs.
When used accurately, cycling can be a protected and efficient approach
to achieve your anabolic objectives. A primary use of Oxandrolone amongst
female bodybuilders is for slicing cycles. It helps them develop a
leaner, more toned physique by decreasing body fat and retaining
lean muscle mass efficiently.
The only means to buy Anabolic steroids if from Underground
labs (black markets), which carry the chance of not even be
steroids. And in lots of cases, most bodybuilders endure from huge side-effects with
out figuring out whatβs the real ingredient in the vile they
injected. The scale is most likely not a dependable
tool to trace progress as a outcome of simultaneous muscle development and fats
loss. Zac Efronβs earlier than and after transformation is
a perfect instance of how a userβs physique modifications after a cycle
of Winstrol. Physique fats decreases while
muscle hypertrophy will increase, as well as muscle definition and
vascularity. The phrases steroids generically refers to a large group of hormones
the physique produces naturally in addition to comparable man-made drugs.
The main notable unwanted effects are likely to
be testosterone suppression and cholesterol alterations.
Anavar is not sanctioned for unsanctioned functions in the majority of countries.
This substance is classed as a managed substance, and its dispensation is proscribed to prescription only in sure jurisdictions.
It is primarily employed for medical causes such as addressing muscle atrophy, osteoporosis or other medically recognized ailments.
Mevolv is a team of people who love what they do and
provides all their dedication to providing fitness applications and companies with real
experience within the health and supplement industries.
It works by increasing manufacturing of the vitality molecule “ATP” by stimulating phosphocreatine synthesis in muscle tissue.
Girls often go for so-called “women-friendly” steroids like Primobolan, Boldenone,
or Nandrolone. It is necessary to notice that
each one anabolic steroids can potentially cause side effects predominantly dependent on the injected dose of the Best Steroid Book.
This implies that correctly dosing injectable steroids is crucial in controlling these unwanted effects.
For this reason, Winstrol will typically be used alone, and
the cycle might be extra average than these aimed toward physique enhancement.
Though females can and do use Winstrol on their own in a cycle, it might be nearly unheard of (and
not recommended) for males to do the identical. This might be a bulking or cutting stack, however most will use it to bulk up AND obtain a dry look as body fats decreases.
The significant advantage of utilizing Tren in a bulking-type stack like this, quite than testosterone, is that you will have much less
water weight. Winstrol use amongst females has become extra
widespread, and this will almost exclusively
be oral Winstrol. This is a low dose, however every further
milligram a feminine takes of Winny does make a big difference and runs the chance
of taking you from a tolerable cycle to one
the place virilization begins to creep in.
Anabolic refers to muscle progress properties, whereas androgenic refers to the promotion of male intercourse traits (16).
Several conditions can result in muscle loss, including AIDS, continual obstructive
pulmonary illness (COPD), most cancers, and kidney and liver illness.
Whereas not as common, AAS can be utilized in these populations to assist preserve muscle mass
(9, 10). CrazyBulk presents a few of the finest legal
steroid stacks, like the Bulking Stack, Cutting Stack, and
the highly effective Ultimate Stack, specifically designed for various health targets.
Look for constantly optimistic experiences regarding power
boosts, quicker workout recovery, energy will increase,
and secure usage. For anyone serious about bulking up, lifting heavier, bettering endurance, and defending their joints, DecaDuro is a top-rated legal steroid complement.
This is as a end result of Dianabol and testosterone are
harsh sufficient by themselves for a newbie protocol.
If newbies would like further muscle and fat loss gains, they
will stack Anavar and testosterone collectively. Nevertheless, the risk of
unwanted aspect effects will increase slightly in comparability with working testosterone alone.
But here’s the real great thing about HyperGH14x –
it’s not just for young bodybuilders seeking to get lean muscle positive aspects.
Remember, as with any powerful muscle building complement, Testol
a hundred and forty works greatest when combined with a solid training program and
correct diet. It Is not a magic capsule, however quite a device to amplify your efforts in the health club.
Used responsibly, Testol 140 might help you obtain the
type of transformation that was once attainable solely with hardcore steroid cycles.
This is not just about libido – it’s about sustaining muscle mass, energy, energy, and overall vitality.
That Is the place Testo Prime is obtainable in, and let me inform you, it’s a powerhouse.
What really units D-Bal Max aside is its capacity to ship fast, noticeable results.
Anabolic steroids have many bodily effects, together with selling muscle progress and increasing energy and power.
Thus, these medication are sometimes used illegitimately to gain a aggressive edge
in sports. Approximately 2% of females and 6% of males worldwide use or
abuse anabolic steroids, with similar estimates for the Usa.
Healthcare providers prescribe them for certain situations, such as male hypogonadism and sure types of breast cancer.
Anabolic steroids, artificial derivatives of testosterone, have
been used for many years to extend muscle mass, energy, and endurance.
By mimicking the effects of naturally occurring testosterone, these compounds accelerate
protein synthesis and muscle restore, providing users significant gains in a comparatively quick period.
If an individual is thinking about taking supplements to help their workouts, they want to first communicate
with a physician to examine the product is safe
for them. Caffeine is a stimulant that may enhance
train performance, notably for activities that contain endurance, similar
to running. If an organization is selling the
product as a dietary supplement, it doesn’t need to conduct checks to determine whether
its merchandise really work. Clenbuterol notably increases coronary heart
fee in our sufferers, which is also supported by research (8).
This occurs due to extreme adrenaline production, putting pointless pressure on the guts.
Crazy Bulkβs Anadrole formula is synthesized to imitate the anabolic results of
Anadrol however with out the cruel unwanted effects.
NEW YORK, April 15, (GLOBE NEWSWIRE) — I actually have
worked with many bodybuilders over 30 years, from professionals to novices.
People usually ask me what the simplest steroid is for
building huge muscular tissues. It works well on its own, and many find
that using different anabolic steroids like Trenbolone and Deca Durabolin along with it provides
much more energy, endurance, and a giant, “swole” look. Welcome to our
complete guide on the best anabolic pathway definition (ihromamz.it) steroids for newbies.
This article goals to offer an overview of the most effective first steroid cycle for bodybuilders simply beginning, offering insights into their
benefits, dangers, and issues. Our first outcomes showed improve in lean physique
mass, though Tau2 and I2 revealed nice heterogeneity
(98 %) on our data. Ottenbacher et al. (2006)) additionally found increases in muscle strength
in another meta-analysis, although the examine was not trying specifically to muscle
mass.
A additional benefit of Andriol (oral testosterone undecanoate) is that it bypasses the
liver and is absorbed by way of the lymphatic system. Consequently, it isn’t
hepatotoxic and poses no threat to the liver, based on our LFTs (liver function tests).
Injectable testosterone undecanoate is a very slow-acting
form of testosterone. Testosterone suspension requires
two injections a day, which isn’t best for a newbie.
Moreover, suspension, in our expertise, is among the worst steroids
to inject, as it requires an enormous needle (a newbieβs worst nightmare).
Anabolic steroids are artificial variations of natural male
sex hormones (androgens). They are used to promote the expansion of skeletal muscle (the anabolic effect) and the event of male sexual traits
(the androgenic effect). Anabolic steroids shorten recovery time by lowering muscle damage and inflammation whereas improving mobile restore rates.
This means athletes can practice extra regularly without
the identical level of fatigue or damage risk (Hartgens & Kuipers, 2004 β Sports Medicine).
Be A Part Of us as we unpack the science behind these anabolic
agents and the way they may help you obtain the physique of your goals.
Get prepared to maximise your gains and rework
your workouts with insights that could change the way in which you
strategy muscle development endlessly.
Itβs unlikely Reeves was using the PED a decade earlier than its medical introduction. So
why not simply be the most effective you you could be by working onerous in the health
club, sticking to a nutritious diet and maintaining away from medicine that
will trigger you health issues. I known as them girlie males as a end result of they werenβt keen to take risks.
Politicians normally need to do little issues so thereβs no danger concerned.
In the long run, itβs better to not say that, since you need to work
with them. [Long pause.] In the old days, thatβs what you needed to say to have people pay consideration to you.
As Soon As seen as helpful, steroids had been now beneath the microscope,
especially after scandals confirmed their dangers.
Right Now, young athletes are often warned about steroid side effects like
kidney harm and coronary heart issues8. What in case your knowledge
about Arnold Schwarzenegger’s steroid use is just
the beginning? The bodybuilding icon himself has shared particulars of his technique.
Steve stands out amongst fellow bodybuilders of the 1940s,
however not in later years. The common testosterone level for men in the 1940s was 700ng/dl.
In fashionable society, the average maleβs testosterone is round 400ng/dl.
The numbers vary but several studies have discovered similar
results.
Arnold Schwarzenegger brazenly talks about steroids, sparking discussions on honesty in bodybuilding.
He admits to using steroids to get big muscles – Audrey
– throughout his aggressive days. Arnold highlights that he used
steroids beneath physician’s care, aiming for secure use.
This is totally different from some today who use steroids
with out steering.
Itβs no secret that steroids have turn out to be more and more common on the planet
of elite sports. From baseball gamers to mixed martial artists and Olympians,
there have been countless instances of athletes using steroids
to gain an edge on the competitors. Muzcle’s content material is for informational and educational functions solely.
Our website is not meant to be an alternative selection to professional medical
advice, diagnosis, or treatment.
All that Iβll say is that he wasnβt the one bodybuilder in his era to use steroids.
Regardless of what he took, it doesn’t change the truth that he outworked his competition.
It additionally does not diminish his thoughts
and experience within the fitness center.
Now, let’s dive deeper into how he built his iconic physique and deal with the myths about efficiency boosters1.
He was launched to the fitness center by his football coach during his teen years.
To this date, the actor continues to raise at the age of 75.
Moreover, Schwarzenegger stresses the fact that the usage of steroids
is frequent among the present generation of bodybuilders that it’s nothing short of a problem to stop them
from using them. Not just this, the fashionable drug
trade has turn into highly refined and this has made issues much more complicated.
Having stated that, he still believes that there is hope and if
things are done correctly then steroid use may be brought down. The bodybuilding business is full of speculation about steroid use.
Itβs no secret that steroid use is commonplace in trendy bodybuilding.
The Mr. Olympia stage today just isn’t similar to bodybuilders of earlier eras.
But youβll see some wonderful strength features at 10mg and even some
lean muscle. With a calorie-deficit food regimen, fat might be easier to
lose, and you may maintain existing muscle. If you employ Ibutamoren alone in a cycle,
you can anticipate moderate muscle positive aspects
of as a lot as 10 lbs. Whereas the potential fats loss results are
useful, they are not as powerful as with some other compounds.
MK-677 is a compound that some people can use with little issue, whereas others donβt
enjoy it as a outcome of theyβre vulnerable to the potential side effects.
This is how we get such a major discount in androgenic-type side
effects while nonetheless getting benefits like muscle development.
Every SARM is completely different, however most are designed to bind to skeletal muscle tissue
receptors. Your training effort and diet will determine how a lot you
acquire, however you probably can simply goal for 10-15lbs and even more.
If you wish to shred fat whereas gaining muscle, Ostarine
will get the body to burn fats effectively, and you’ll notice that youβre sustaining glorious
muscle energy even while dropping fat.
The finest part of this is that these muscle building supplements do not comprise any sugar,
fillers or additives.2, finest steroid cycle for gaining muscle.
BCAAsThe greatest bodybuilders complement to bulk your body up fast was also named as top-of-the-line
dietary supplements for bulking, finest steroid cycle for lean muscle acquire.
These are a proven efficiency enhancing ingredient that may allow you to achieve dimension in a
small period of time.3.
At the identical time, a calorie deficit
is a crucial outcome of any profitable chopping cycle. Skinny guys will
find that this stack offers noticeable bulk comparatively shortly.
Eat enough calories (both high quality and quantity), and youβll
see these shirts filling out within a few weeks,
and different people will begin to discover. Greatest of all, thereβs no testosterone suppression, no PCT required, no excessive ldl cholesterol or blood strain, water retention, pimples,
or hair loss. In most circumstances, S23 users will be
stacking it with no much less than considered one of these different compounds.
When it comes to steroids, those that we will most carefully compare with S23 are the powerhouse-cutting steroids of Anavar and
Winstrol.
Testosteroneβs androgenic results can complement the fat-burning results
of Anavar, albeit with some short-term water retention. Thus, testosterone may be considered
a complementary stacking partner, as it is an androgenic steroid, so DHT ranges will remain high throughout a cycle.
Deca Durabolin is not as highly effective as testosterone, so increases
in muscle hypertrophy are unlikely to be excessive.
Nonetheless, this impact can also assist cushion joints during heavy lifting, reducing the chance of
harm. For nearly all of performance enhancers, when planning a stack the primary concern shall
be cutting or bulking. As one or the opposite is generally the primary concern,
we need to be aware that sure steroids better serve each purpose.
Furthermore, the dangers of misusing anabolic steroids have been well-studied and understood for years.
It is used for constructing stamina within the physique
and for strengthening the muscular tissues.To enhance the
protein stage within the physique one can purchase steroids online which is a very effective drug.
A number of natural ingredients complemented with a number
of steroids have been used to arrange Loopy Mass stacks
which allow you to get that six-pack look in a relatively quick period of time.
The oxygen fuels and strengthens your muscular tissues, which may
then delay fatigue so you’ll have the ability to work more durable for an extended time period.
It works by boosting the number of your pink blood cells, which permits your muscles to absorb extra oxygen when youβre working
out. Ostarine tremendously helps with nutrient partitioning, this feature helps to achieve muscle while losing fat.
Ostarine is half as anabolic steriods online as testosterone and has been shown to be useful in therapeutic or preventing injuries to tendons, bones and
ligaments. Anavarβs ability to increase power and energy may be attributed to its distinctive stage of creatine uptake contained in the muscle
cells (15), which will increase ATP (adenosine triphosphate) production. With trenbolone being 3x
extra androgenic than testosterone (12),
its important results on muscular power aren’t shocking.
Thatβs as a end result of itβs one of the powerful steroids ever,
which routinely makes it a major candidate for the
most effective steroid cycle for size features.
Tren, as fans name it, is definitely 5 occasions stronger than testosterone.
It also helps your physique to extend purple blood
cell manufacturing to let your muscle tissue get extra oxygen.
Weβll maintain tabs on these supplements as they
evolve and keep making an attempt them for ourselves to make sure this information stays current.
You lift weights and eat more than upkeep calories, and the
muscle ought to grow. A Number Of components can get in the way
of success in the health club, from the quality of the food youβre eating to your genetics.
For users that aren’t concerned about their health,
trenbolone and Winstrol may produce the best outcomes.
After beginning TRT, Scott dropped 60 pounds, vanquished his
fatigue, and is reinvigorated at work. Ten squat reps at 315 pounds shortly dropped to two
and he was figuring out with 25 p.c vitality. After one yr of testosterone injections,
Brian is down 50 kilos and his waist measurement dropped from forty eight to 38 inches.
His power improved so much, he cut out all caffeine and works out with military-like intensity.
Typical AAS-induced physical unwanted effects in men embrace efficiency problems, pimples,
and gynaecomastia. Typical psychiatric unwanted effects embody
melancholy, sleep disorders and mood disturbances (Sjoqvist et al., 2008).
Women generally take fewer substances and decrease doses (Borjesson et al., 2016, 2020).
Research show that ladies seek healthcare earlier than males
for the negative effects they expertise (Garevik et al., 2011; Borjesson et al.,
2016).
The strength of a lady is generally lower
than that of a man because of physiological differences.
Also, the voice, skin texture, and other secondary sexual characteristics remain sometimes feminine.
Earlier Than beginning an Anavar cycle, it is essential to analysis
and perceive the correct dosage, potential side effects,
and post-cycle therapy. With correct use and monitoring, Anavar can present noticeable improvements in muscle
size, strength, and general physique. Anavar is considered to be a mild steroid compared to different anabolic buying steroids Online reviews, meaning it has a lower threat
of unwanted aspect effects. It is also much less efficient for constructing muscle compared to stronger steroids like Dianabol
or Deca-Durabolin.
No matter what you chooseβAnvarol, Winsol, or
Trenorolβjust make informed decisions to attain your bodybuilding and fitness aspirations responsibly.
If however, the lady is an experienced Anavar consumer, she might start with
10mg per day and likewise lengthen the cycle to six
weeks. As far as males are involved, the optimum dosage
is inside the range of 15-20mg/day of Anavar with the cycle size being up to 6 weeks.
This is likely to help you practice with greater intensity and help you lift heavier weights
easily. One of essentially the most crucial factors that
is easily missed when looking at earlier than and after photographs such as
these above is the power features that Anavar brings with it.
“The image on the left is my senior homecoming after being on prednisone for a quantity of months.
Whereas itβs crucial to strategy steroid usage with caution and under skilled steerage, understanding the advantages can provide useful insights. Anavar has been studied extensively over the years for its potential benefits when it comes to muscle progress and strength features. A Quantity Of scientific research have been performed to look at the effects of Anavar on the physique, as well as its potential side effects.
Although injectable steroids continue to pose significant risks to the center. Research has proven that Anavar can scale back high-density lipoprotein (HDL) ldl cholesterol notably, growing the danger of hypertension, left ventricular hypertrophy, and myocardial infarction (11). Moreover, we have discovered regular cardiovascular coaching to have a notable reductive impact on blood strain in our sufferers. Though these protocols might prevent cardiotoxicity, they shouldn’t be thought of a band-aid or a whole remedy for Anavarβs toxicity.
Mendacity is a tool to escape disgrace and avoid being confronted by disapproval and rejection. Only a quantity of people, among the many closest and most trusted, learn about an individual’s use of AAS. “When my physique
got muscle tissue, they roared with laughter at me and said that the lads’s department
is on the other facet of the streetβ¦you really feel a bitβ¦.divided
about how to gown your self should you’re very muscular.
If I go in clothes or skirts and stuff, then I really feel like persons are taking a look at me like I Am a transvestite.
As little as 5 mg to 20 mg day by day might be helpful whereas mitigating potential unwanted aspect effects like voice deepening or physique hair progress, that are undesirable for a lot of girls.
Keep In Mind, Oxandroloneβs efficiency permits even modest
doses to have substantial results. Understanding the benefits
and potential drawbacks of incorporating Anavar right into a bodybuilding cycle is essential for many who wish to optimize their efficiency and
obtain their physique goals.
This situation often results in a relentless vicious cycle, the place the
preliminary benefit incrementally turns into
a big liability. Girls generally do not stack Anavar with one other steroid for worry of virilization. Nonetheless, men often stack it with other anabolics and are able to
obtain nice results too.
I think this iss one off the most important information for
me. And i am glad reading your article. But should commentary on some nomal things, The site taste is great,
the articles is really great : D. Just right job, cheers
Feel free to visit my website … http://www.actionfigurenews.Ca
I am curious to find out what blog system you have been wolrking with?
I’m having some minor securrity issues with my latest blog and I’d like to
fund something more secure. Do you have any suggestions?
Have a look at my page: http://Www.jeepin.Com
I’ve een exploring for a little bit for aany high quality article or weblog posts on this sorrt
of area . Exploring in Yahoo I at last stumbled upon this website.
Studying this information So i am satisfied to show
that I have an incredibly just right uncanny feeling I discovered exactly
what I needed. I most definitely will make surre
to don?t forget this site and provides it a glance on a relentless basis.
Here is my webpage … http://Spearboard.com/member.php?u=810794
I’ve learn some excellent stuff here. Certainly value bookmarking for revisiting.
I surprise how a lot effort you set to create this sort
of excellent informative site.
Also visit my webpagee :: http://Www.dendymaster.ru/forum/showthread.php?p=46675
Please let me know if you’re looking for a article author for your site.
You hhave some really great posts and I feel I would bbe
a good asset. If you ever want to take somke of the load off, I’d absolutely
love to write some contdnt for your blog in exchange forr
a link back to mine. Please send me an e-mail if interested.
Thank you!
My site: http://www.smokinstangs.com/member.php/494162-Sergahk
Hi there! I realoize this iss sort oof off-topic but I needed to
ask. Does operating a well-established wwebsite like yours
require a lot of work? I’m brand new to running a blog but I do write in my journal on a daily basis.
I’d like to start a blog so I can share my experience and views online.
Please let me know iff you have aany kind of recommendations or tips ffor branmd nnew aspiring bloggers.
Appreciate it!
My blog post … Penney
Heya i am for the first time here. I came across this board and I
in finding It truly helpful & it helped me out a lot.
I’m hoping to present one thing again and aid others such as you aiced
me.
Also visit my web blog … Mckinley
ΠΡΠΈΠ²Π΅ΡΡΡΠ²ΡΠ΅ΠΌ Π½Π° Π½Π°ΡΠ΅ΠΌ ΡΠ°ΠΉΡΠ΅!
ΠΠ΄Π΅ΡΡ Π²Ρ Π½Π°ΠΉΠ΄Π΅ΡΠ΅ Π²ΡΡ, ΡΡΠΎ Π½ΡΠΆΠ½ΠΎ Π΄Π»Ρ
ΡΡΡΠ΅ΠΊΡΠΈΠ²Π½ΠΎΠ³ΠΎ ΡΠΏΡΠ°Π²Π»Π΅Π½ΠΈΡ Π²Π°ΡΠΈΠΌΠΈ
ΡΠΈΠ½Π°Π½ΡΠ°ΠΌΠΈ. Π£ Π½Π°Ρ ΡΠΈΡΠΎΠΊΠΈΠΉ Π²ΡΠ±ΠΎΡ ΡΠΈΠ½Π°Π½ΡΠΎΠ²ΡΡ ΠΏΡΠΎΠ΄ΡΠΊΡΠΎΠ², ΠΊΠΎΡΠΎΡΡΠ΅ ΠΏΠΎΠΌΠΎΠ³ΡΡ Π²Π°ΠΌ Π΄ΠΎΡΡΠΈΠ³Π½ΡΡΡ Π²Π°ΡΠΈΡ ΡΠ΅Π»Π΅ΠΉ ΠΈ ΠΎΠ±Π΅ΡΠΏΠ΅ΡΠΈΡΡ Π±ΡΠ΄ΡΡΠ΅Π΅.
Π Π½Π°ΡΠ΅ΠΌ Π°ΡΡΠΎΡΡΠΈΠΌΠ΅Π½ΡΠ΅ Π΅ΡΡΡ
Π±Π°Π½ΠΊΠΎΠ²ΡΠΊΠΈΠ΅ ΠΏΡΠΎΠ΄ΡΠΊΡΡ, ΠΈΠ½Π²Π΅ΡΡΠΈΡΠΈΠΈ,
ΡΡΡΠ°Ρ ΠΎΠ²Π°Π½ΠΈΠ΅, ΠΊΡΠ΅Π΄ΠΈΡΡ ΠΈ
ΠΌΠ½ΠΎΠ³ΠΎΠ΅ Π΄ΡΡΠ³ΠΎΠ΅. ΠΡ ΡΠ΅Π³ΡΠ»ΡΡΠ½ΠΎ ΠΎΠ±Π½ΠΎΠ²Π»ΡΠ΅ΠΌ Π½Π°ΡΡ Π±Π°Π·Ρ Π΄Π°Π½Π½ΡΡ , ΡΡΠΎΠ±Ρ Π²Ρ Π±ΡΠ»ΠΈ Π² ΠΊΡΡΡΠ΅ ΠΏΠΎΡΠ»Π΅Π΄Π½ΠΈΡ Π½ΠΎΠ²ΠΎΡΡΠ΅ΠΉ ΠΈ ΠΈΠ½Π½ΠΎΠ²Π°ΡΠΈΠΉ
Π½Π° ΡΠΈΠ½Π°Π½ΡΠΎΠ²ΠΎΠΌ ΡΡΠ½ΠΊΠ΅. ΠΠ°ΡΠΈ ΡΠΏΠ΅ΡΠΈΠ°Π»ΠΈΡΡΡ ΠΏΠΎΠΌΠΎΠ³ΡΡ Π²Π°ΠΌ Π²ΡΠ±ΡΠ°ΡΡ Π»ΡΡΡΠΈΠΉ ΠΏΡΠΎΠ΄ΡΠΊΡ, ΡΡΠΈΡΡΠ²Π°Ρ Π²Π°ΡΠΈ ΠΈΠ½Π΄ΠΈΠ²ΠΈΠ΄ΡΠ°Π»ΡΠ½ΡΠ΅
ΠΏΠΎΡΡΠ΅Π±Π½ΠΎΡΡΠΈ ΠΈ ΠΏΡΠ΅Π΄ΠΏΠΎΡΡΠ΅Π½ΠΈΡ. ΠΡ ΠΏΡΠ΅Π΄ΠΎΡΡΠ°Π²Π»ΡΠ΅ΠΌ ΠΊΠΎΠ½ΡΡΠ»ΡΡΠ°ΡΠΈΠΈ ΠΈ
ΡΠ΅ΠΊΠΎΠΌΠ΅Π½Π΄Π°ΡΠΈΠΈ, ΡΡΠΎΠ±Ρ Π²Ρ ΠΌΠΎΠ³Π»ΠΈ
ΠΏΡΠΈΠ½ΡΡΡ Π²Π·Π²Π΅ΡΠ΅Π½Π½ΠΎΠ΅ ΡΠ΅ΡΠ΅Π½ΠΈΠ΅ ΠΈ ΠΈΠ·Π±Π΅ΠΆΠ°ΡΡ ΡΠΈΡΠΊΠΎΠ².
ΠΠ΅ ΡΠΏΡΡΡΠΈΡΠ΅ ΡΠ°Π½Ρ Π²ΠΎΡΠΏΠΎΠ»ΡΠ·ΠΎΠ²Π°ΡΡΡΡ Π½Π°ΡΠΈΠΌΠΈ
ΡΡΠ»ΡΠ³Π°ΠΌΠΈ ΠΈ ΠΎΡΠΊΡΠΎΠΉΡΠ΅ Π΄Π»Ρ ΡΠ΅Π±Ρ ΠΌΠΈΡ ΡΠΈΠ½Π°Π½ΡΠΎΠ²ΡΡ Π²ΠΎΠ·ΠΌΠΎΠΆΠ½ΠΎΡΡΠ΅ΠΉ!
ΠΠΎΡΠ΅ΡΠΈΡΠ΅ Π½Π°Ρ ΡΠ°ΠΉΡ, ΠΎΠ·Π½Π°ΠΊΠΎΠΌΡΡΠ΅ΡΡ Ρ ΠΊΠ°ΡΠ°Π»ΠΎΠ³ΠΎΠΌ ΠΏΡΠΎΠ΄ΡΠΊΡΠΎΠ²
ΠΈ Π½Π°ΡΠ½ΠΈΡΠ΅ ΠΏΡΡΡ ΠΊ ΡΠΈΠ½Π°Π½ΡΠΎΠ²ΠΎΠΉ
ΡΡΠ°Π±ΠΈΠ»ΡΠ½ΠΎΡΡΠΈ ΠΏΡΡΠΌΠΎ ΡΠ΅ΠΉΡΠ°Ρ!
ΠΠΊ ΠΠ°ΡΡ ΠΠ°Π½ΠΊ – ΠΊΡΠ΅Π΄ΠΈΡΠ½Π°Ρ ΠΊΠ°ΡΡΠ° 115 Π΄Π½Π΅ΠΉ Π±Π΅Π· ΠΏΡΠΎΡΠ΅Π½ΡΠΎΠ²
That is very interesting, You’re an overly skilled
blogger. I’ve joined your rss feed and look forward to searching for extra of
your great post. Additionally, I have shared your
website in my social networks https://www.uniladtech.com/news/tech-news/man-spent-10000-bitcoin-two-pizzas-worth-144371-20241205
Howdy! Do you use Twitter? I’d like to follow you if that would be okay.
I’m definitely enjoying your blog and look forward
to new posts.
Also visit my page: site
hi!,I like your writing so so much! proportion we be in contact
more approximately your article on AOL? I need a specialist on this
house to solve my problem. May be that’s you! Looking
ahead to look you. https://prostata-hifu.mystrikingly.com/
Thanks in favor of sharing such a nice thought, piece of
writing is good, thats why i have read it fully https://wakelet.com/wake/3gVHh656RRayDTtTeKosV
This information is invaluable. Where can I find out more? https://cystoscope-wiscope.blogspot.com/2025/02/the-disposable-cystoscope-wiscope-why.html
This is very interesting, You’re an excessively professional blogger.
I’ve joined your fed and sit up for seeking extra of your great post.
Also, I’ve shared your web site in my social networks
Also visit mmy blog – Vavada.Webgarden.com
I’d like to thank you for the efforts you have put in penning this site.
I really hope to view the same high-grade blog posts from you later on as well.
In fact, your creative writing abilities has motivated me to get my own, personal
blog now π
[…] Console log […]