Part 3: Working with Files and Directories
Welcome back to our “Mastering Bash Scripting for Embedded Linux Development“ series! In this third part, we’ll explore essential techniques for working with files and directories in Bash scripting. From file manipulation to directory traversal and pattern matching, mastering these concepts will empower you to efficiently manage and manipulate data within your embedded Linux systems.
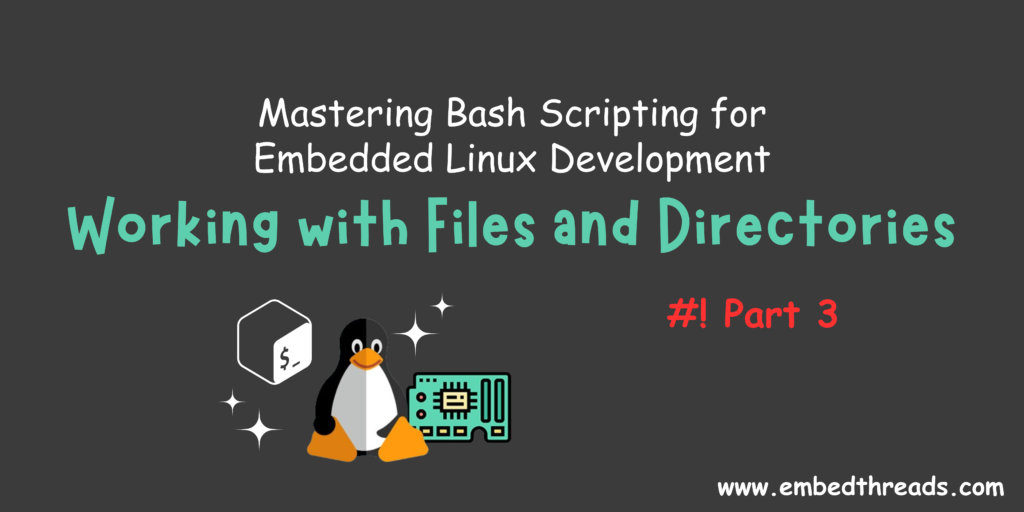
File Manipulation
Bash scripting provides powerful tools for manipulating files, including creating, reading, writing, and deleting them.
Creating a File
Use the touch a
command followed by the filename to create an empty file. For example,
# Create a new file
touch new_file.txt

Besides touch
, you can also use redirection with echo
to create files with content. For instance:

Reading from a File:
The cat
command is handy for displaying the contents of a file directly in the terminal. For example,
# Read the contents of a file
cat greeting.txt

Apart from cat
, you can use head
or tail
to display the beginning or end of a file, respectively. For example:
head greeting.txt

Note: When using the head
command, by default, it prints the first 10 lines of a file. Similarly, the tail
command prints the last 10 lines of a file by default. However, if the file contains fewer than 10 lines, head
or tail
will print all the lines available in the file. For example, executing tail greeting.txt
will print the last 10 lines of the greeting.txt
file if it contains 10 or more lines. If the file contains fewer than 10 lines, tail
will print all of them.
Writing to a File
You can use redirection (>
or >>
) to write to a file. For example,
# Write to a file
echo "Hello, world!" > greeting.txt

Append content to a file using >>
. For example, to add more text to greeting.txt
:
# Append to a file
echo "Welcome to Embed Threads" >> greeting.txt

Deleting a File
The rm
command followed by the filename removes a file. Be cautious when using rm
as it deletes files permanently without confirmation. For example,
# Delete a file
rm greeting.txt

Note: Use wildcards with rm
to delete multiple files. For instance, to remove all .txt
files in the current directory: rm *.txt
Directory Traversal
Navigating directories and accessing files within them is a common task in Bash scripting. You can use commands like cd
, ls
, and pwd
to traverse directories and perform operations on files.
Changing Directories
Use the cd
command followed by the directory name to change your current working directory. For example,
# Change directory
cd /path/to/directory
Listing Contents
The ls
command lists the contents of a directory. Adding options such as -l
provides detailed information about files, including permissions and ownership.
# List files in the current directory
ls
# Print the current directory
pwd

cd ~
: Changes the current directory back to the user’s home directory (/home/alok
).cd "Embed Threads"
: Changes the current directory to a directory namedEmbed Threads
. However, note that the directory name contains a space, so it should be enclosed in quotes to be interpreted as a single argument.cd ..
: Moves up one directory from/home/alok/Embed Threads
to/home/alok
.cd documents
: Attempts to change the directory to a directory nameddocuments
. However, it results in an error messagebash: cd: documents: No such file or directory
because there is no directory nameddocuments
in the current directory (/home/alok
).
Moving Files
You can move files between directories using mv
. For instance, to move file.txt
from the current directory to Documents
:
mv file.txt Documents/
Copying Files
Similarly, copy files with cp
. For example, to copy file.txt
to a backup folder:
cp file.txt backup/
File Permissions and Ownership
Understanding file permissions and ownership is crucial for securing your files and controlling access. In Linux systems, each file has permissions for the owner, group, and others, denoted by read (r
), write (w
), and execute (x
).
- Viewing Permissions: Utilize the
ls -l
command to view permissions. Permissions are displayed as a sequence of characters, such as-rwxr-xr--
, where the first three characters represent permissions for the owner, the next three for the group, and the last three for others. - Changing Permissions: The
chmod
command allows you to change file permissions. Syntax:chmod [permissions] filename
. For instance,chmod +x script.sh
adds execute permissions toscript.sh
. - Ownership: The
chown
command changes the ownership of files. Syntax:chown [user]:[group] filename
. For example,chown user:group example.txt
changes the ownership ofexample.txt
.

The string -rwxr-xr-x 1 root root 0 Mar 24 13:18 script.sh
represents the file permissions and metadata associated with the file named script.sh
. Let’s break down each part of this string:
- Permissions: The first part of the string
-rwxr-xr-x
indicates the permissions of the file. Each character represents the permissions for the file’s owner, the group the file belongs to, and others (users who are not the owner and not in the group). The characters have the following meanings:-
indicates that the corresponding permission is not granted.r
indicates read permission.w
indicates write permission.x
indicates execute permission.
rwx
indicates that the owner of the file (root
) has read, write, and execute permissions.r-x
indicates that the group (root
) has read and execute permissions but not write permissions.r-x
indicates that others (users who are not the owner and not in the group) have read and execute permissions but not write permissions.
- Number of Hard Links: The number
1
after the permissions indicates the number of hard links to the file. Hard links are additional names for the same file on the file system. In this case, there is only one hard link to the file. - Owner and Group: The next two entries
root root
indicate the owner and group of the file. In this case, the owner isroot
and the group is alsoroot
. - File Size: The number
0
indicates the size of the file in bytes. In this case, the file size is0
, meaning the file is empty. - Date and Time:
Mar 24 13:18
represents the date and time when the file was last modified or created. In this case, the file was modified or created on March 24th at 13:18 (1:18 PM). - Filename: Finally,
script.sh
is the name of the file.
The above explanation illustrates symbolic notation, but you can also utilize octal numbers with the chmod
command.
- Read (r) is represented by the value 4.
- Write (w) is represented by the value 2.
- Execute (x) is represented by the value 1.
- No permission (-) is represented by the value 0.
To determine the octal value of a set of permissions, you simply add up the values for each permission. For example:
- If a file has read, write, and execute permissions for the owner, the octal value for the owner’s permissions would be 4 (read) + 2 (write) + 1 (execute) = 7.
- If a file has only read and execute permissions for the group, the octal value for the group’s permissions would be 4 (read) + 0 (no write) + 1 (execute) = 5.
- If a file has only read permission for others, the octal value for others’ permissions would be 4 (read) + 0 (no write) + 0 (no execute) = 4.
So, in octal notation, the file permissions -rwxr-xr-x
would be represented as 755
:
- Owner: Read (4) + Write (2) + Execute (1) = 7
- Group: Read (4) + Execute (1) = 5
- Others: Read (4) + Execute (1) = 5
To modify file permissions using octal notation, you would use the chmod
command followed by the octal representation of the desired permissions. For example:
# Change file permissions
chmod 755 script.sh
Bash Scripting
Example 1: Create 10 Files with Random String
#!/bin/bash
# This script creates 10 files with random strings as their content
# Define files to create
files=("file1.txt" "file2.txt" "file3.txt" "file4.txt" "file5.txt"
"file6.txt" "file7.txt" "file8.txt" "file9.txt" "file10.txt")
# Create files with random content
for file in "${files[@]}"; do
# Generate a random string
random_string=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
echo "$random_string" > "$file"
done
echo "Files created successfully!"
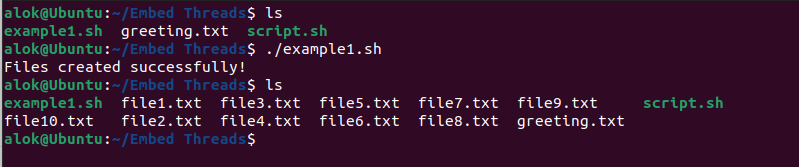
Example 2: Automated File Backup Script
#!/bin/bash
# This script creates a backup of specified files
# Define files to backup
files="file1.txt file2.txt"
# Create a backup directory if it doesn't exist
backup_dir="backup"
if [ ! -d "$backup_dir" ]; then
mkdir "$backup_dir"
fi
# Copy files to the backup directory
for file in $files; do
cp "$file" "$backup_dir/"
done
echo "Backup completed successfully!"

Example 3: File Permission Checker
#!/bin/bash
# This script checks the permissions of a file
# Define file to check
file="file1.txt"
# Check file permissions
permissions=$(ls -l "$file" | cut -d ' ' -f 1)
echo "Permissions of $file: $permissions"

File Globbing and Pattern Matching
Bash provides powerful mechanisms for matching filenames using patterns:
Wildcard Characters
Use wildcard characters such as *
(matches any string) and ?
(matches any single character) for pattern matching. For example, ls *.txt
lists all files with a .txt
extension.
# List all files with a .txt extension
ls *.txt
# Delete all files starting with "temp"
rm temp*
Brace Expansion
Brace expansion allows you to generate arbitrary strings. For example, echo file{1..3}.txt
will display file1.txt
, file2.txt
, and file3.txt
.
cat file{1..3}.txt

Character Classes
Match files with specific characters. For instance, to list files with numbers in the name:
ls *[0-9]*

Bash Scripting
Example 4: Pattern Matching Script
#!/bin/bash
# This script lists all text files in the current directory
# List text files
echo "Text files:"
ls *.txt

Example 5: File Cleanup Script
#!/bin/bash
# This script deletes all text files in the current directory
# Delete all text files
find . -name "*.txt" -type f -delete
echo "Text files deleted successfully!"

In this part of our series, we’ve explored essential techniques for working with files and directories in Bash scripting. From file manipulation to directory traversal and pattern matching, these concepts are foundational for managing data within your embedded Linux systems.
FAQ
Q: Can I create a file and a directory with the same name?
A: No, a file and a directory cannot share the same name in the same location. However, they can have the same name if they are in different locations.
Q: How can I view the contents of a file without using cat
, less
, or more
?
A: You can use the head
command to display the first part of a file, or the tail
command to display the last part of a file.
Q: What is the difference between >
and >>
?
A: The >
operator overwrites the file with the output of the command, while the >>
operator appends the output to the end of the file.
Q: How can I delete a directory that is not empty?
A: You can use the rm -r
command to remove a directory and its contents. Be careful with this command, as it will delete the directory and all of its contents without asking for confirmation.
For more detailed information, you can always refer to the official GNU Bash documentation.
That’s all for today’s post.
Stay curious, keep exploring, and happy scripting!
This concludes Part 3 of our series. Stay tuned for future installments!
Have you ever thought about creating an ebook or guest authoring
on other websites? I have a blog based on the same information you
discuss and would really like to have you share some stories/information. I know my viewers would enjoy your work.
If you are even remotely interested, feel free to send me an email.
In Deutschland allerdings gehört Dianabol zu den verbotenen Substanzen und ist authorized nicht zu kaufen. Auch ein anonymer Versand derartige Substanzen wird nicht empfohlen, ganz egal, ob die Dianabol Einnahme für männliche Bodybuilder geeignet ist oder nicht von einer längerer Anwendung ausgegangen werden muss. Methandrostenolon wird jedoch bis zum heutigen Tag in wenigen Ländern, zumeist in sogenannten Untergrundlaboratorien hergestellt und illegal vertrieben und niemand weiß genau, welche Wirkstoffe das Produkt enthält. Neben der am weitesten verbreiteten oralen Darreichungsform, wie beispielsweise Kapseln zu je 50 mg, existieren injizierbare Produkte, die Methandrostenolon als Wirkstoff beinhalten. Ich hoffe, dass meine Erfahrung etwas Aufschluss darüber geben wird, wie Apotheken in der Türkei funktionieren.
Hier muss jeder für sich selbst entscheiden, ob er er sein Budget belasten möchte. Eine Unterzuckerung wiederum kann negative Folgen für den Schlaf und Verhalten von Betroffenen haben(14). Anwender können diesen Umstand verhindern, indem sie Ribose immer im Zusammenhang mit Kohlenhydraten zu sich nehmen.
Mehr Informationen finden Sie in unserer Hilfe unter “Fragen zu Kundenbewertungen” und dann unter “So überprüfen wir Kundenbewertungen”. Die vorliegenden Informationen sind nicht als Ersatz für professionelle medizinische Beratung gedacht. Personen, die beabsichtigen, Cannabisblüten für medizinische Zwecke zu verwenden, sollten einen Arzt oder einen anderen qualifizierten Gesundheitsdienstleister konsultieren, um eine individuelle Beratung und Überwachung zu erhalten. Wir möchten Ihnen daher anhand der Inhaltsstoffe der Cannabispflanze, den Cannabinoiden THC und CBD sowie den Terpenen, eine Möglichkeit geben, die für Sie möglicherweise passende Sorte zu finden. HGH oder auch Menschliche Wachstumshormone steigern den Muskelaufbau sowie die körperliche Leistungsfähigkeit. Bei Aminosäuren handelt es sich um lebenswichtige Stoffe, die an der Bildung neuer Proteinstrukturen im Körper maßgeblich beteiligt sind(11). Die Säuren werden über die Ernährung aufgenommen und verfügen über bestimmte Aufgaben, wie bei etwa verschiedenen Stoffwechselprozessen.
D-Bal MAX empfiehlt beispielsweise drei Kapseln täglich mit Mahlzeiten, während TestoPrime vier Kapseln vor dem Frühstück vorsieht. Die genauen Dosierungsanweisungen finden sich stets auf der Produktverpackung und sollten strikt eingehalten werden. Einhaltung der vom Hersteller angegebenen Tagesdosis ist Pflicht, um auf der sicheren Seite zu bleiben und unerwünschte Wirkungen zu vermeiden, da Überdosierungen keine besseren Resultate liefern.
Booster versprechen, den Hormonspiegel Ihres Körpers wieder auf ein Niveau zu bringen, dass Sie zu Ihrer Jugend hatten. Besonders On-line gibt es viele dieser Dietary Supplements.(5), die im Gegensatz zu anderen Steroiden Dosen den Vorteil haben, dass keine Spritze gesetzt werden und muss und kaufen online möglich ist. Die Vorstellung, Muskeln zu verlieren und der Kampf gegen die Prozesse, die mit der natürlichen Alterung einhergehen, sind die meistgenannten Gründe, warum die Einnahme von HGH Präparaten ungeheuer populär geworden ist. Wenn Sie Sportler oder Bodybuilder sind, ist es nur natürlich, dass Sie eine Extraportion Energie und Muskelkraft brauchen, um sich von Ihren Konkurrenten abzuheben. Ihr natürliches HGH reicht nicht aus, um Ihnen diesen schnellen Bodybuilding- und Reparaturvorteil zu verschaffen. In einem solchen Szenario brauchen Sie ein Ergänzungsmittel, das die HGH-Produktion anregt, damit Sie Ihren Körper besser aufbauen und match bleiben können.
Die Übersäuerung des Muskels wird so verhindert und sorgt für mehr Kraft beim Coaching. Unter den beliebtesten Zusatzstoffen findet sich vor allem Creatin Monohydrat(6). Der Körper kann Creatin zwar selber produzieren, allerdings nur etwa 1 bis 2 Gramm täglich. Für sämtliche Körperfunktionen ist diese Ration in der Regel ausreichend, kommt jedoch Kraftsport hinzu, müssen Sportler Creatin extra zuführen. Durch die zusätzliche Einnahme von HGH X2 wird gewährleistet, dass Muskeln schneller aufgebaut werden und die Regenerationsphase kürzer ausfällt. Sogar die häufig auftretenden Schmerzen in den Gelenken werden von denen, die HGH X2 eingenommen haben, nach einer mehrwöchigen Nutzung, als Schnee von gestern bezeichnet.
HGH X2 ist das Nahrungsergänzungsmittel, für all diejenigen, die trotz einem intensiven Coaching keine Muskeln ansetzen oder nicht die erwünschte Masse an Muskeln erreichen. In der Regel wird HGH vom Körper durch die körpereigene Hypophyse selber produziert, dieses lässt jedoch mit Zunahme des Alters ab. Das Produkt zeichnet sich weiterhin dadurch aus, dass es keine verbotenen Substanzen enthält, sondern nur aus pflanzlichen und somit natürlichen Inhaltsstoffen hergestellt wurde und aus diesem Grund, bestens für eine Nutrition ist.
Neben Arzneimitteln und qualitativ hochwertiger Kosmetik finden Sie in unserer Onlineapotheke auch Medikamente für Ihr Haustier in unserer Tierapotheke in großer Auswahl. Mit diesem Beitrag distanzieren wir uns ausdrücklich, von den hier aufgeführten Präparaten und raten dringend, von der Einnahme illegaler Substanzen ab. Auch bei kurzfristiger Anwendung können diese Mittel zu ernsten Gesundheitsschäden führen. In Apotheken oder Drogerien werden Sie vergeblich nach Dianabol suchen. Ein Kauf ist lediglich im Web möglich, wobei es sich nicht als einfach erweist, einen seriösen Anbieter auszumachen. Besser ist auf jeden Fall nach einer guten Different zu suchen, die sich zudem als kostengünstiger erweist. Sogar bei Fortgeschrittenen, erscheint eine Nutzung von zwischen 15 bis forty five mg pro Tag, als vollkommen ausreichend.
References:
https://hi-fi-forum.net/profile/1048392
oral steroids for sale
References:
marriagesofa.com
casino stars review
Hmm is anyone else having problems with the images on this blog loading?
I’m trying to determine if its a problem on my end or if
it’s the blog. Any suggestions would be greatly appreciated.
anabolic legal steroids
References:
guardian.ge
kewadin casino st ignace
References:
thief river falls casino
black jack davey
References:
rudrait.com
santa barbara casino
References:
http://guardian.ge
It’s quick to use, taking around 10 to 15 seconds to download a 5-minute video.
Очень люблю отечественные драмы — актёры просто огонь.
Площадка явно крутая штука, если хотите искать новые сезоны —
Недавно посмотрел новый сезон — нашёл на этом сайте:
Озвучка топ — на одном дыхании глянул.
Всё это —
Спасибо за крутые подборки.
Теперь всегда смотрю сериалы на https://EKT-Rostelecom.ru/ — захожу каждый день.
Как вам сериал? — обсуждение тут в тему.
70918248
References:
some studies have found a reduced risk for acne among people consuming (trefpuntstan.be)
70918248
References:
anabolic steroid use may cause all of the following side effects except (https://silviaseco.com.br/)
70918248
References:
Trenbuterol steroid (ask.zarooribaatein.com)
Thanks on your marvelous posting! I quite enjoyed reading it, you can be a great author.
I will always bookmark your blog and will often come back from now on. I want to encourage you to
ultimately continue your great posts, have a nice evening!
I used to be able to find good advice from your articles.
70918248
References:
buy Steroids from russia – kolegium-urologi.id,
70918248
References:
anabolic steroids Brands (https://petratungarden.se/2016/11/07/color-trends-spicy-mustard-dusty-burgundy-och-mint)
My coder is trying to convince me to move to .net from PHP.
I have always disliked the idea because of the costs.
But he’s tryiong none the less. I’ve been using WordPress
on a number of websites for about a year and am concerned about switching to another platform.
I have heard great things about blogengine.net.
Is there a way I can import all my wordpress posts into it?
Any help would be really appreciated!
The sexy look of Momo and her unimaginable beauty caught the eye
of producers who helped the sexy Japanese lady change into one of
the members of the South Korean group Twice. They said that
there was simply this one small thing, which when you notice it, there would
be nothing in Heaven or earth that will keep you from being free,
besides perhaps your individual self. 25 September 2015 (NY thug expected to plead guilty)
A brand new York thug is predicted to plead responsible after strolling as much as a automotive and taking pictures somebody in it, for no rational reason. 23 November 2015
(France attacking itself) France handed an “emergency” legislation permitting the state
to impose home arrest on anyone with no judicial evaluation, and study any pc without a warrant.
13 July 2013 (Urgent: Stand firm towards Walmart bullying and signal dwelling wage act) Everyone: urge the mayor of Washington DC to stand agency towards Walmart bullying and sign the living wage
law.
Hello would you mind sharing which blog platform you’re using?
I’m going to start my own blog soon but
I’m having a tough time choosing between BlogEngine/Wordpress/B2evolution and Drupal.
The reason I ask is because your design and style seems different then most blogs
and I’m looking for something unique. P.S My apologies for getting off-topic but I had to ask!
I like the valuable info you provide in your articles. I will bookmark your weblog and check
again here regularly. I’m quite certain I will
learn plenty of new stuff right here! Good luck for
the next!
Cependant, il arrive parfois qu’un utilisateur
ait besoin de le convertir dans un autre format.
In abstract, Anavar provides a plethora of benefits, including muscle mass enhancement, energy improvement, fats reduction, and quicker recovery occasions.
Its efficacy and comparatively gentle side effect profile make it a
priceless software for these within the bodybuilding and
athletic communities. Anavar is utilized by people who have experienced excessive weight reduction because of myriad medical conditions.
Individuals affected by osteoporosis use this medication to alleviate
the ache from bone deterioration. Aside from these, as an anabolic steroid, Anavar
oxandrolone can also be administered by folks aiming to achieve weight and physique mass for
bodybuilding purposes.
Large Pharmacy uses superior know-how to source drugs that meet
global healthcare requirements, ensuring that clients receive safe and efficient products.
An important part of a secure and profitable cycle is sourcing a
high-quality product. Not all Anavar on the market is
real; some suppliers offer counterfeits that may contain ineffective or even harmful components.
To avoid these pitfalls, Buy Pro Chem Anavar 50Mg Tablets only
from established distributors that present batch verification or have a strong monitor document among
other athletes. Check for official product codes or holographic
seals, and confirm them through the manufacturer’s web site.
Even in 2025, the united states market continues
to prize Pharmacom Labs as a prime, time-tested provider.
Bodybuilding communities consistently report that Oxandrolonos supports the development of dry muscle and helps keep energy after a cycle.
Anavar is offered each as a pharmaceutical grade product and an underground lab variation. Anavar bought
in pharmacies follows stricter high quality control as in comparison with its underground counterparts, which
possess various ranges of purity and value. Be cautious if you are going for
Anavar offered in underground circles, as its requirements
aren’t controlled. Anvarol is an natural supplement designed
to attain the same objective as steroids like Anavar in a more pure strategy
and without the usual unwanted aspect effects or withdrawal signs.
Not surprising, these unwanted effects have increased the number of people who
depend on alternative dietary supplements.
One important side to consider when using Oxandroloneis the administration technique.
Different administration strategies can have varying effects on the physique
and overall outcomes.
Anavar is a well-liked anabolic steroid that is generally utilized by bodybuilders and athletes to help enhance
muscle strength, cut back physique fat, and enhance total performance.
Quite A Few web sites specialize within the sale of performance-enhancing substances,
including anabolic steroids. It is important to research and select reputable
online shops that present high-quality merchandise.
Look for websites that provide secure payment strategies,
discrete packaging, and have constructive customer reviews
and suggestions.
They are known for their rigorous testing procedures and commitment to quality.
These faux steroids are not only ineffective but also can pose severe health risks.
Sellers often lure consumers with suspiciously low costs, but when it comes to steroids, quality and authenticity ought to never be compromised.
Nutritionists specializing in performance-based diets within the US recommend pairing Anavar cycles
with a high-protein, moderate-carb food regimen to maximize muscle
retention. Meals like lean rooster breast, sweet potatoes, and spinach are staples in the diets of American health fanatics using Anavar.
Anavar Lite may be used in rehabilitation settings, helping individuals get well from injuries or surgical
procedures that have resulted in muscle loss. It aids in sustaining muscle mass during times of limited bodily activity.
Additionally, anavar is thought to spice up bone density, that may aid within the prevention of osteoporosis in addition to other bone-related illnesses.
Additionally, it might help in growing red blood cell production, which aids in bettering
oxygen supply to the muscle and enhancing stamina. Additionally, we supplies detailed packaging with clear directions
to support first-time users and experienced athletes in administering the product properly.
As Soon As you’ve sourced high-quality Anavar, correct
usage is the following crucial step. Reliable suppliers usually share Certificates of Evaluation (COAs) or impartial lab outcomes proving the product incorporates actual Oxandrolone
on the advertised dosage. Buying poor-quality Anavar isn’t just a waste of
money—it can compromise your health and your outcomes.
This complete information breaks down every thing you have to find out about Anavar for Sale, from identifying reliable products to understanding correct use and
avoiding common scams. Tamil actor Srikanth arrested by
Chennai police after buying medication price Rs…
These nations would possibly remain safe if conflict spreads,
not US, China, Russia, they’re…
Dosages may vary, and periodic breaks from the medication might be necessary.
It’s usually used alongside different treatments, so ensure you perceive your complete regimen. CrazyBulk can be providing a buy-two-get-one-free deal on all of its merchandise at the
moment, so it’s a nice time to start your bulking or slicing cycle.
Anavar can help to increase power and endurance, which can be helpful for athletes.
Anavar has since been used by bodybuilders and athletes as a means to assist
them construct muscle and improve strength. The similar could be
stated with Anavar or any other performance enhancing drug, there are advantages but as we’ve
discussed there are risks too. Nevertheless, folks in these international locations have to be cautious to not abuse
this steroid, as like with any drug – should you abuse it, you’re in bother.
Anavar is especially well-liked in bodybuilding, with it
considered the most popular steroid available on the market at present.
Var’s in high demand as a result of ladies take it as
well as males (which can’t be mentioned about
other steroids). Additionally people who are
apprehensive about steroid’s unwanted effects are extra inclined to take anavar because
the risks are lower. When discussing the potential execs of purchasing Oxanabol, the listing is
really extensive. No matter the reason for your low testosterone, Anavar
together with different anabolic steroids can successfully boost
your testosterone levels safely and effectively.
Hi! I could have sworn I’ve been to this website before
but after reading through some of the post I realized it’s new to
me. Nonetheless, I’m definitely glad I found it and I’ll be bookmarking and checking back frequently!
Étape 2 Saisissez ensuite l’URL de la vidéo YouTube que vous souhaitez
convertir au format MP4 dans la nouvelle fenêtre.
Very shortly this web page will be famous amid all blog people, due to it’s fastidious articles
Superb blog you have here but I was wondering if you knew of any user
discussion forums that cover the same topics
discussed in this article? I’d really like to be a part of community where I can get feedback from other experienced individuals that share the same interest.
If you have any suggestions, please let me know. Many thanks!
70918248
References:
natural bodybuilding vs Steroids pictures
70918248
References:
none
Studies point out that HGH has the potential to stimulate your thymus gland, which is
answerable for producing immune cells. Incorporating HGH dietary
supplements into your routine may signal your thymus gland to generate the
next number of T-cells, thereby amplifying your immune
system. Even if you’ve solely skilled a minor decline in your libido, it’s likely
that you’ll observe an uptick in your sexual urge for food when you start using HGH supplements.
With the exception of PGH-1000, a lot of the dietary supplements we’ve highlighted hover across the $60 to $70 mark for a
30-day provide. But the entire companies listed present bulk order discount pricing, so if you purchase two packages you will get one other for free.
Either means, it is necessary to just remember to are purchasing HGH from a reputable supply.
Artificial version of human growth hormone buy uk Progress Hormone known as Somatropin (191 amino
acid sequence development hormone). All HGH merchandise, together with any Qomatropin supplied, are supplied by Evolution LAB UK strictly for in-vitro laboratory analysis.
MELANOTAN 2 is a synthetically produced variant of a peptide hormone naturally produced within the physique that stimulates melanogenesis,
a course of liable for pigmentation of the skin. GHRP-6 peptide which is
structurally very similar to its predecessor, GHRP-2 The
primary distinction is that the GHRP-6 will increase urge for food in some
people. Like ghrelin, it stimulates release of endogenous development hormone from somatotropes
within the anterior pituitary; additionally like ghrelin, it’s synergistic with endo..
Some men use progress hormone injections in bodybuilding and sports, but
we don’t recommend you try this with out medical supervision. As
the name suggests, the human progress hormone is a vital mediator of the human growth course of.
HGH is a small protein made naturally within the physique, created by the pituitary
gland after which secreted into the bloodstream. HGH is a naturally occurring
hormone within the body answerable for stimulating growth,
cell regeneration, and sustaining wholesome tissue. Athletes and health lovers typically use HGH to speed up muscle
restoration, enhance strength, and enhance endurance.
Moreover, HGH can assist in reducing body fat and enhancing pores and skin elasticity, making it a versatile answer for these
focused on health and efficiency. In kids and adolescents, it stimulates the growth of bone and cartilage.
In people of all ages, GH boosts protein production, promotes the utilization of fat, interferes
with the action of insulin, and raises blood sugar levels.
Verified HGH provides high-quality IGF-1 products that
are good for those trying to enhance muscle growth, fats loss, and overall
health. When you purchase HGH and IGF-1 from us, you may be investing in products which are confirmed to deliver results.
According to some studies, having extra HGH in your system could improve the general high quality of your
sleep. If you discover it difficult to go to sleep or sleep soundly throughout the night, contemplate
taking one the most effective HGH supplements that’s listed here.
This might help you obtain a deeper, more restful
sleep, and reduce episodes of insomnia.
Common exercise might change your development hormone degree, keeping it from dropping too much, particularly as you age.
Some folks use the hormone, along with different performance-enhancing medication such as anabolic steroids, to construct muscle and improve athletic performance.
This contains any possible side effects not listed within the bundle leaflet.
All of those formulas are injections, however they differ in dose, price,
and method of preparation and use. For instance, some HGH prescriptions
involve the use of traditional medical syringes, while others come in disposable
injection pens that already include the proper dosage amount.
Your doctor will decide the right HGH prescription for you
based mostly in your personal preference, lifestyle, and well being
needs. As quickly as you drift off to sleep, your physique begins to secrete development hormones.
Proathletepharma sports pharmacology store presents a variety of supplements,
peptides, anabolics and other merchandise to enhance
the appearance and efficiency of the body. Many of those that are
struggling with low ranges of HGH may be considering the choice of using synthetic HGH injections.
Let’s look into the pros and cons of natural progress hormone supplements in comparison with injections.
After testosterone, maybe the preferred hormone that men are looking to enhance is HGH, or human development hormone.
Nebido will present all the same benefits and effects that you just expertise with another testosterone steroid,
but they’ll take much longer to begin taking place.
Most bodybuilders need to begin seeing outcomes within the first few weeks of a cycle, and this is not possible when using Nebido as a main anabolic compound.
Only in the most specialist of circumstances will Nebido be an appropriate alternative for a bulking or chopping cycle.
Testosterone Enanthate is the most well-liked ester variant of the testosterone steroid.
It has a half-life of about 8 to 10 days and is a slow-release
testosterone steroid utilized in an injectable kind both for efficiency enhancement or as a TRT.
Studies have in reality demonstrated the incredible effectiveness
of HCG for this purpose, and it is even advised clinically that HCG be utilized for the aim of treating
anabolic steroid induced hypogonadism[4].
Oral Winstrol starts working quicker than injectable, however they are both thought of fast-acting steroids.
Whichever type you’re taking, you’ll achieve the
identical results by the tip of the cycle. Outcomes with Winstrol are
brief and sharp, and when used correctly, you’ll come away with a constructive
experience and satisfying outcomes from a Winstrol cycle.
All anabolic steroids include substantial health dangers when used outdoors of medical suggestions.
Winstrol is not any exception, although it’s considered one of
many milder steroids we will use compared to many other compounds.
This relative mildness is what makes it one of many only a few steroids that females can also use.
Post cycle therapy, known as PCT for short, a short course
of remedial action the place you can reset your our bodies
and permit it to perform usually without relying on hormones.
I contemplate this a little bit of a distinct segment AAS and one that I don’t turn to usually, however after I use it,
I’m all the time happy with the outcomes (make certain you get the actual deal when buying Tbol).
This is a steroid that pharmaceutical company now not manufactures,
so it’s only out there from underground labs on the black market.
This makes it prone to poor quality and dangerous ingredients, so choosing a
reliable supplier is paramount. Costs range wildly, however Turinabol is amongst the
lower-cost steroids available. As Trenbolone can linger in your
system for months and even years, PCT will not essentially restore normal testosterone
function.
We Have examined its advantages, such as fast muscle progress and power gains, alongside its quite a few potential side effects, together with liver toxicity,
cardiovascular pressure, and hormonal imbalances.
All anabolic steroids include excessive risks to health each within the
short and long term. Parabolan comes with side effects that embody androgenic results, potential impacts on cholesterol,
and moderate to extreme testosterone suppression. Utilizing
Parabolan at excessive doses will increase the chance of its turning into unsafe.
Still, many people use it regularly at low to reasonable doses, with sufficient breaks between cycles to permit time for the physique to recover.
Excessive doses of this amount will enhance mass
and power features, as will the risk of androgenic unwanted effects.
Advanced users on a chopping cycle will probably need to persist with a 40mg every day dose, which makes use of Turinabol
primarily as a muscle preservation compound quite
than a mass-gaining one.
Nonetheless, if you’re combining Testosterone Enanthate with any other steroids
that have a more adverse effect on ldl cholesterol, this steroid will solely compound on that.
The alternative class of drugs to control estrogenic unwanted effects – SERMs – don’t include the risk of ldl cholesterol issues (they can benefit cholesterol).
The process of aromatization causes testosterone
to convert to the feminine hormone estrogen. Whereas males naturally want very small quantities of estrogen,
when ranges rise too high and hormones turn out to be imbalanced, undesirable
unwanted aspect effects are almost inevitable. Dianabol offers you a fast
kick-off to this bulking cycle, while Testosterone Enanthate takes a couple of weeks to start delivering results.
This is a good choice for many who do not wish to wait a number of weeks
for outcomes when stacking with one other gradual ester like Deca
(see my following stack below). A 12-week newbie cycle dosed at 250mg to 500mg weekly offers
a wonderful introduction for the newbie by using the safest steroid in testosterone.
Consequently, this can lead to high blood pressure and increased risk of coronary heart
assault. Maintaining correct hydration and a heart-healthy food regimen may help mitigate these cardiovascular
unwanted effects. This steroid is not any stranger to these
within the bodybuilding and health industry.
Dianabol represents one of the most well-liked and one of the most necessary Anabolic steroids of all time.
To some, this is the most well-liked oral steroid to ever hit the market and some of the in style steroids in any type.
He, like many others, skilled vital muscle-building and
bulking results from this powerful oral anabolic steroid.
One common facet effect is gynecomastia, the development of breast tissue in males.
This happens as a end result of a rise in estrogen attributable to Dianabol’s aromatizing properties.
Additionally, Dianabol can suppress pure testosterone
manufacturing, resulting in testicular atrophy and potential fertility issues.
This pattern of use will increase the chance of serious health problems and can make it tough for users
to take care of their physique with out continued steroid use.
Whereas no anabolic steroid is actually “safe,” Turinabol is commonly considered one of the least harsh oral compounds for newbies.
It doesn’t convert to estrogen, has a lower risk of androgenic unwanted aspect effects, and delivers dry, sustainable gains—especially when run at average doses (20–40mg/day)
for no extra than 8 weeks with proper PCT. Yes, all testosterone steroids have powerful anabolic effects,
which promote muscle development via increased protein synthesis, nitrogen retention, purple blood cell manufacturing, and boosting IGF-1 levels.
One Other cycle for advanced users includes
the highly effective Anadrol, which can make for a perfect low season bulking stack
with Testosterone Cypionate. 50-75mg every day of Anadrol for the primary
six weeks, with Testosterone Cypionate at 400mg for weeks.
Injectable Dianabol Post Cycle Therapy might thus be
advantageous as a result of its lower liver toxicity.
Nevertheless, we understand some users prefer the comfort of swallowing a
capsule somewhat than studying the method to inject (which can be painful and dangerous if carried out incorrectly).
Additionally, there’s a threat of customers contracting HIV
or hepatitis via intramuscular injections if needles
are shared. We start these drugs as soon as Dianabol has
totally left the physique. You can work out when a drug will leave your physique by 5.5 times the
half-life. Thus, ladies who aren’t competing and want to maintain their femininity intact will go for steroids such as Anavar (oxandrolone), which is less more probably to trigger the above side
effects. Dianabol barely will increase ranges of the major androgen in men, DHT (dihydrotestosterone).
Nonetheless, the long-term results and security profile of SARMs aren’t entirely understood
as a result of they haven’t undergone as much medical research as traditional anabolic steroids.
Most guys will discover features of 20 pounds more than passable, and also you don’t need high doses to attain that.
Even a 4-week reasonably dosed cycle can have you gaining 15 kilos,
or should you select to increase to eight weeks with the best food plan and coaching, then 25lbs+
is greater than achievable. Remember that the longer
you utilize it, the more pronounced the unwanted effects will become.
EVLution s’est fixé pour mission d’être votre partenaire nutritionnel le plus
confiant pour atteindre vos objectifs de conditionnement physique.
Les utilisateurs ont signalé des changements radicaux dans leur corpulence
et leurs performances sexuelles après juste quelques semaines d’utilisation. Au bout d’une période de 3 à 4 mois, la plupart d’entre eux ont connu une transformation complète de tout leur corps,
un rehaussement de leur humeur et une motivation accrue pour entreprendre.
Nos produits sont rigoureusement sélectionnés pour leur efficacité et leur sécurité, vous
garantissant une expérience d’achat fiable et satisfaisante.
Le principal inconvénient du Check E est qu’il peut être converti en œstrogène.
Dans ce contexte, une gynécomastie se produit, un excès de liquide s’accumule dans le
corps, un œdème apparaît et de la graisse se dépose selon le
sort féminin.
Ce médicament doit être utilisé avec précaution si vous
souffrez d’épilepsie et/ou de migraine automotive
elles pourraient s’aggraver. Ne prenez pas de dose double pour compenser
la dose que vous avez oublié de prendre. Après
forty eight heures enlevez les patchs et mettez les
dans la boîte de récupération prévue à cet effet
(voir rubrique 6 de la notice). Eviter les zones où la peau est grasse, avec une transpiration abondante ou couverte de poils automotive le patch pourrait ne pas bien adhérer.
TESTOPATCH doit être appliqué sur une peau propre, sèche et saine, au niveau des bras,
du bas du dos ou des cuisses. La dose recommandée est de 2 patchs
de TESTOPATCH appliqués toutes les forty eight heures sur la
peau. En raison de la variabilité des résultats entre les différents laboratoires,
tous les dosages doivent être effectués par le même laboratoire pour un sujet donné.
Si vous souhaitez voir de tels résultats, assurez-vous d’acheter testostérone en ligne auprès de sources fiables.
Il existe plusieurs types de testostérone, chacun ayant ses propres avantages et inconvénients.
Vous pouvez acheter de la testostérone cypionate ou acheter de la testostérone énanthate,
les deux formes les plus populaires.
Enfin, elle sera excellente pour vous préserver des blessures, automotive elle protège
les articulations. Nombreux sont les pratiquants de musculation qui souffrent au niveau des articulations
le plus souvent à trigger d’une mauvaise alimentation et/ou d’une mauvaise technique.
En effet, cet acide aminé fait partie des 22 acides aminés protéinogènes.
Comme vous le savez, les acides aminés forment une chaîne qui crée les protéines.
Plusieurs études démontrent l’importance de la vitamine D
sur la santé, notamment le psychological,
la densité osseuse, le système immunitaire et le métabolisme.
D’autres recherches prouvent également qu’elle peut augmenter de manière significative les niveaux de testostérone existante et donc renforcer les propriétés anti-vieillissement de cette dernière.
Certains produits, tels que l’Androgel ou le Testogel, peuvent être obtenus sans prescription médicale en passant par une session en ligne.
Lorsque vous souhaitez acheter de la testostérone en ligne, il est
essential de vous assurer de la fiabilité de la supply.
Optez pour des pharmacies en ligne réputées qui exigent une prescription médicale avant de vous
délivrer des produits de testostérone. Assurez-vous que
ces pharmacies en ligne opèrent légalement en France et respectent les normes de sécurité et de qualité.
C’est l’une des meilleures combinaisons
pour accroître la vitesse de développement de vos fibres musculaires après
une séance de musculation. La Whey est une protéine en poudre qui va reconstituer et renforcer vos fibres musculaires après
avoir travaillé vos muscles. La testostérone quant à elle
est anabolisante, c’est-à-dire qu’elle va aussi augmenter
la synthèse de protéines pour un effet maximal. D’une half, les ingrédients représentent la clé
de voûte de votre produit. Sans de bons ingrédients, et si possible naturels, votre booster de testostérone
s’écroule.
Cellucor COR-Performance ZMA est un puissant booster
de testostérone qui peut vous aider à augmenter vos
niveaux sanguins, tout en améliorant la qualité de votre sommeil.
Il est conçu pour vous aider à mieux récupérer après vos exercices, en vous aidant à dormir profondément
et à vous reposer davantage. L’acide D-aspartique
est un ingrédient qui a cliniquement prouvé sa capacité à soutenir la libération et la synthèse de l’hormone lutéinisante et favoriser les taux endogènes de
testostérone pendant l’entraînement. Jouant
un rôle necessary dans le soutien métabolique, l’acide D-aspartique peut
conduire à augmenter la taille des muscle tissue ainsi qu’à l’augmentation des processus de combustion des graisses naturelles.
Afin de produire une forme constante et efficace,
le shilajit récolté à partir de roches soigneusement sélectionnées est purifié et standardisé à partir d’une technologie brevetée.
Cette forme stabilisée permet de fournir des niveaux optimaux de composants
bioactifs tels que l’acide fulvique et les dibenzo-pyrones.
Acclamé par les critiques et adulé par les bodybuilders du monde
entier, TestoFuel est le booster de testostérone numéro
1.
L’utilisation d’anabolisants conduit à la synthèse active des protéines, des érythrocytes,
augmente l’endurance et la pressure, accélère la récupération des tissus après des
blessures et des brûlures. Le cypionate de testostérone
peut être conservé pendant 3 ans dans de bonnes conditions.
La testostérone est la principale hormone sexuelle masculine et androgène.
Il est synthétisé par les cellules de Leydig des testicules chez l’homme, en petite quantité par les ovaires
chez la femme et par le cortex surrénalien chez l’homme et
la femme. Vérifier les témoignages et
les avis est une excellente façon de se renseigner sur la qualité
d’un produit.
Nous vous offrons la possibilité d’acheter de la testostérone
injectable en ligne, avec une livraison rapide et discrète partout en France,
afin de répondre à vos besoins spécifiques en matière de musculation. Votre
but est de prendre du muscle de façon naturelle
et sans danger, pas vrai ? Alors, pour optimiser vos résultats et atteindre vos objectifs plus rapidement, nous
vous conseillons de combiner votre booster de testostérone avec d’autres compléments alimentaires.
70918248
References:
wrest point casino (theunintelligenteconomist.com)
70918248
References:
none
70918248
References:
Live casino android (impulscomp.ru)
70918248
References:
none; healthaturkey.Com,
70918248
References:
none (Willian)
70918248
References:
none (15Minutestravel.com)
70918248
References:
best cutting steroid (https://theroamingsuitcase.com)
70918248
References:
What Is Deca Steroid (https://Centre-Urologie-Boumerdes.Com/Annonce-Importante-Changement-Dadresse-Du-Centre-Durologie-Boumerdes)
70918248
References:
where to buy anabolic steroids bodybuilding (Muoi)
70918248
References:
steroid supplement for bodybuilding (Tawanna)
70918248
References:
Steroids for sale – http://www.Consultrh.fr –
70918248
References:
Psychological effects Of steroids (grandcouventgramat.fr)
70918248
References:
do steroids stunt your growth (bellavita-lefkada.gr)
Samuelsson says he doesn’t use them, thoughsome of his rivals don’t believe him.
Reevesmarvels at the memory of Kaz’s hoisting
a 220-pound sack of sandand whipping through a 200-meter course in 42 seconds.
Frank’s frank talk makes the strongmen really feel like choosing up,say, a caboose and dropping
it on his head, however there is nothingthey can do about it.
Solely Dickerson would change his thoughts by finally deciding
to enter the Mr. Olympia, which was again promoted by Schwarzenegger and Lorimar in Columbus, Ohio once more.
CBS Tv spent plenty of cash traveling to Sydney, Australia to film
the 1980 Mr. Olympia. After witnessing the controversial choice, they decided to not air the competition in spite
of everything. The popular tv show “60 Minutes” contemplated doing a
narrative on the contest due to its unpopular
outcome. When Arnold was finally announced in first place as Dickerson walked onstage to merely accept the runner-up position, he jumped up
and down off stage yelling, “I can’t believe it! Arnold made a speech after being handed the Sandow trophy by Joe Weider, acknowledging how shut the decision was. He brought his shut good friend Franco to the podium, thanking him for his help and help.
It will enable the dedicated athlete and coach to plan out training, food plan, and recovery strategies for optimum outcomes. If you presumably can get well higher, you possibly can practice extra, prepare tougher, and make higher positive aspects over time. The Scientific Ideas of Hypertrophy Coaching is a basic analysis of what your coaching should seem like if muscle progress is your major goal, or even just certainly one of your objectives. The guide takes you on a grand tour of all of the primary seven coaching ideas and how to apply them to one’s coaching – regardless of your experience degree, diet part, or sport. Inevitably, taking more of any steroid will lead to extra well being complications, hence why bodybuilders similar to Arnold are still alive now at 72 years of age and in relatively good well being.
Columbu was the winner of the Mr Universe competitors in 1970 & 1971, and later went on to win the 1974 & 1975 Mr Olympia (under 200-pound class). Franco claimed the overall Mr. Olympia Title in 1976 and again in 1981. Franco Columbu, nicknamed the “Sardinian Strongman,” was a legendary figure on the earth of bodybuilding. Born on August 7, 1941, in Ollolai, Sardinia, Italy, Columbu’s unimaginable journey took him from humble beginnings to turning into a two-time Mr. Olympia winner and a close pal of Arnold Schwarzenegger.
We all know there are two components to constructing muscle, diet & exercise. Franco knew this and took advantage of it by releasing “The Bodybuilding Diet Book” with cowriter Lydia Fragomeni. As you’ll be able to most likely guess it dealt with what a diet ought to encompass, good meals and foods for anabolism, fat-carb-protein ratios, distinctive body sorts and their food plan needs, and such. Pliable fascia allows for straightforward and dramatic enlargement when a muscle is flexed. A thick fascia is dmz a steroid a positive benefit involved sports activities and strength events.
The bodybuilder is readying for the professional stage together with his personal brand
of “science.” “As A Outcome Of if folks think it happened while I was doing bench presses, they will think steroids brought on it.” In 1977, he
competed in the first World Strongman Competitors, which was televised around the globe.
Lots of bodybuilders eat floor meat to make digestion simpler, but
Columbu loved his steak and ate it all the time.
He says hamburger wasn’t out there when he was
growing up in Italy. Columbu ate an incredible quantity
of protein every day, together with plenty of eggs,
steak, hamburger, cottage cheese, bacon, and cheese.
The unpredictability of a strongman competitors is what provides itsome of its scrumptious carnival charm.
In Hawaii the handles onsome of the weights carried up steps in the back-bending powerstairs occasion fell off simply earlier than that climactic competition wasto begin, with
Ahola leading Samuelsson by just one point.
So Ahola was declared the winner, leavingSamuelsson with hearth in his eyes and Kristin with tears in hers.
His death is widely attributed to steroid use but no concrete proof has been introduced ahead to verify that.
Columbu was a legendary bodybuilder, identified for being an in depth friend and coaching associate to Arnold Schwarzenegger.
Moreover, he held the title of Mr. Universe, together with numerous other
accolades.
McCarver was a 26-year old 3-time IFBB winner who collapsed
and later died due to an upper respiratory an infection. He was participating in Arnold
Classic in Australia when the tragic occasion occurred.
At solely 26 years of age, McCarver had gained three out
of 5 IFBB competitions he had participated in. This should offer you
a clear picture of what the younger man was to attain had he
lived longer. Condolences to the family and friends of this
bodybuilding nice.
Doctors studied Dianabol – methandrostenolone – and mentioned to be
handiest steroid (I am not sure myself) and best overall gains.
Throughout the sixties and seventies steroid use was not controlled, so
bodybuilders, wrestlers, power lifters, weight lifters and
others, if they wished, might inject as a lot as they needed.
Franco Columbu’s coaching routine used to have plenty of depth.
To get through his intense training sessions, he always made it a
point to keep his physique well hydrated. For that purpose, he used to eat plenty of water all through the day
and particularly before his training periods. Via this publish,
we will be throwing light on Franco Columbu’s food regimen plan that earned
him the nickname The Sardinian Strongman.
First of all, age is essential; steroids are solely utilized by
folks over the age of 21 to avoid inhibiting pure growth.
It is the time in your body to generate its construction,
and hormones – in abstract, it’s your potential for the longer term.
But Dianabol also appears to be much more potent when it comes to muscle mass in comparability with boldenone, supporting
the notion that estrogen does play an important function in anabolism.
In truth boldenone and methandrostenolone differ a lot in their potencies as anabolics that the 2 are not often although of as related.
As a outcome, the utilization of Dianabol is usually restricted to
bulking phases of coaching whereas Equipoise is considered an excellent cutting or lean-mass
constructing steroid. In order to face up to oral administration, this compound is c17 alpha alkylated.
We know that this alteration protects the drug from being deactivation by the liver (allowing practically all the drug entry into
the bloodstream), nevertheless it can additionally
be poisonous to this organ.
Elevated androgen plasma concentrations suppress gonadotropin-releasing hormone (reducing endogenous testosterone), luteinizing hormone,
and follicle-stimulating hormone by a negative-feedback mechanism.
Testosterone additionally impacts the formation of erythropoietin, the
balance of calcium, and blood glucose. Androgens have a excessive lipid solubility,
enabling them to rapidly enter cells of target tissues.
Inside the cells, testosterone undergoes enzymatic
conversion to 5-alpha-dihydrotestosterone and forms a loosely
sure advanced with cystolic receptors.
Every of the varieties has its advantages, for instance, using an injection type,
side effects are much less doubtless, and oral steroids are more convenient to make use of and Do Steroids Make You Fat not require
particular skills. Their advantage is that the motion of the drug manifests
itself much sooner, but its duration is way less than that
of injections. Although this drug is touted as “the simplest anabolic”
from athletes to eat proper it does not cause the identical results that the additives described above.
We provide steroids which would possibly be obtainable in each injection and oral form – this allows you to select the
drug most suited to you. Each of the varieties has its own advantages, for example,
when utilizing injections, the unwanted effects are much less likely to occur
while oral steroids are extra convenient to make use of
and don’t require particular information. Steroids come
in numerous types, every with its own properties and
functions. Generally speaking, they are often divided into oral steroids, injectable steroids, and ancillary steroids.
Every sort has completely different benefits and dangers, so it’s essential to determine on the right one in your specific wants
and goals. The twin action of steroids for muscle development and masculinizing effects both spotlight their
energy as properly as their in depth influence on the body.
It is these complex maneuvers that make the steroids attention-grabbing for drugs as
a possible therapeutic device, but also attention-grabbing in them because of their
vital function inside sports activities and bodybuilding.
Prolonged publicity to c17 alpha alkylated substances may end up in actual damage, possibly even the development of certain kinds of cancer.
To be protected one might want to go to the physician a couple of
occasions during every cycle to control their liver enzyme values.
With Dianabol there might be also the potential of aggravating a male sample baldness situation. Delicate individuals could due to this fact wish to keep away
from this drug and opt for a milder anabolic such as Deca-Durabolin. The androgenic
metabolite 5alpha dihydromethandrostenolone is therefore produced solely in trace amounts at greatest.
One of the main advantages of Decadurabolin is its ability to
enhance nitrogen retention in the muscle tissue, which
is essential for protein synthesis and muscle development. By retaining more nitrogen in the muscle tissue, Decadurabolin helps to extend
the speed of protein synthesis and accelerates restoration time after workouts,
permitting you to train harder and extra incessantly.
PCT aims to mitigate the potential adverse effects of suppressed testosterone ranges and help the body regain its pure hormonal
stability. Anavar (Oxandrolone) is a popular anabolic steroid identified for
its potential to ship important outcomes by means of muscle definition, strength improvement, enhanced endurance, and fats
loss. When used responsibly and as part of a well-rounded
fitness routine, Anavar might help people achieve their desired
physique and performance targets. Nonetheless, using anabolic steroids purely to extend muscle size
and energy, particularly without a prescription, is taken into account abuse.
In the Usa, most anabolic steroids are regulated by the Managed Substances
Act, and it’s illegal to possess them with no prescription. Steroids, also referred to as anabolic-androgenic
steroids (AAS), are synthetic derivatives of testosterone, the primary male
sex hormone.
T3’s effects on tissues are 4 times more potent than its prohormone T4.
Of the thyroid hormones produced by the physique, solely 20% are T3,
whereas 80% are T4. 85% of the T3 that circulates within the blood is fashioned by the removal
of the iodine atom that’s hooked up to the 5 carbon atom
of T4.
One of its major actions is its anabolic effect, which boosts protein synthesis.
By selling the manufacturing of proteins within muscle
cells, Anavar facilitates muscle growth and recovery.
When used correctly underneath medical steerage,
anabolic steroids offer powerful advantages but additionally carry notable
well being dangers.
Browse our extensive product line, profit from expert
recommendation, and enjoy safe, hassle-free shopping for.
Attaining your fitness goals with anabolic steroids is totally
potential when accomplished responsibly and with steerage from a trusted supply.
Bio Genetics USA stands as your associate on this empowering journey,
dedicated to providing you with one of the best products and help out there.
We are certified and active IFBB pros, we are additionally a good
on-line anabolic steroid supply with over ten years of experience on this field.
Our major goal is that will assist you obtain your muscle-building, bulking or weight loss objectives safely.
PharmaHub is your trusted source to purchase anabolic steroids within the USA and Europe along with your
Credit Card.Safe and fast on-line funds.
It’s important to understand that imbalances in these can result in variations in measurement.
We recognise that whereas testosterone significantly impacts penis measurement during key developmental periods, its role diminishes
after puberty. Creatine doesn’t hinder hormone balance, testosterone production, or sexual efficiency.
The dimension of the penis is set by the quantity of erectile
tissue it accommodates, which is essentially decided by genetics.
Testosterone supplements might have some effect on libido and sexual operate,
however they are unlikely to cause a everlasting increase in penis dimension. Throughout
puberty, when testosterone ranges increase, the penis grows on account of increased cell division and
progress of penile tissues.
Nonetheless, it is crucial to notice that testosterone substitute
remedy, when administered beneath medical supervision and in appropriate
doses, doesn’t sometimes cause permanent changes in penis size.
In reality, research have proven that testosterone remedy may help restore penile development and reverse the consequences
of testicular shrinkage attributable to low testosterone
ranges. Testosterone production primarily takes Best Place To Buy Steroids inside the
Leydig cells in the testes, under the control of luteinising hormone (LH) which is launched from the pituitary gland.
One of the potential risks of testosterone therapy is
the disruption of pure hormone manufacturing.
When external testosterone is introduced into the physique through therapy, it can suppress the body’s natural manufacturing of the hormone.
This can lead to a range of unwanted facet effects and complications, including testicular shrinkage and fertility issues.
It is necessary to note that the consequences of anabolic steroids usually are not solely due to the testosterone they
comprise. Anabolic steroids also produce other elements that can disrupt the normal hormonal
stability in the body. Subsequently, it is not correct to solely attribute any potential decrease in penis size to testosterone injections alone.
If you’re serious about using these procedures,
it’s essential to strategy them carefully because improper practices can potentially cause hurt or scarring.
By sucking blood into the region, vacuum pumps produce a
transient engorgement that causes the penis to briefly enlarge.
These drugs usually forestall the immune system from functioning abnormally, which is how
they primarily combat certain autoimmune sicknesses. Males may have to utilise extra super tadarise for erection, as it could additionally alter other penile capabilities.
Some medications even comprise it, they usually
usually function by lessening the impact of the immune system.
Steroid users do not necessarily need to run a PCT,
especially if gentle compounds are utilized, similar to Primobolan and Anavar.
Such steroids solely have a moderately lowering effect on endogenous testosterone ranges,
based mostly on our SHBG tests. One examine found that males
who had previously taken steroids had higher ranges of VF in comparability with non-steroid customers
(2). This is fats that surrounds the organs and cannot be seen by
the bare eye, but high ranges can push the stomach out, making a ‘protruding’ appearance.
This is also identified as steroid gut in the bodybuilding community and is initially brought
on by impaired insulin sensitivity. In one study, a bunch of untrained mice was administered
steroids (testosterone), inflicting exceptional muscle development.
They take 10 to 100 times greater than the doctor-recommended TRT dose.
When the testes (testicles), which produce sperm and the hormone testosterone, shrivel or become smaller, this is
referred to as testicular atrophy. This disorder can be brought on by a quantity of things, but it is frequently linked to the use of anabolic steroids since it upsets the body’s regular
hormone stability. A key player in controlling libido, erectile perform, muscle progress, and basic health is testosterone, the
main male sex hormone. Erectile dysfunction (ED) can result from
the misuse or abuse of exogenous testosterone, particularly when large
dosages or prolonged use are involved. Long-term
prednisone use might quickly have an result on sexual operate by way of
hormonal adjustments and decreased blood circulate, however permanent dysfunction is rare.
Most results resolve after treatment discontinuation or
dose adjustment.
When it involves discussions about masculinity and virility, the scale of a man’s penis
typically comes up. Many folks consider that the next testosterone level
in males is directly linked to a bigger penis
size. But is there any scientific fact to this age-old assumption? In this
text, we are going to explore the connection between high
testosterone levels and penis size, shedding mild on the precise
scientific evidence behind this in style perception. Analysis suggests
a extensive selection of environmental and way of life components can affect testosterone.
It’s well-documented that substances like alcohol and
tobacco can result in decreased testosterone ranges.
One small-scale research performed in 2013 investigated the results of testosterone replacement remedy
(TRT) on penile measurement in a group of men with low testosterone levels.
The research found that there was no significant change in penile length or girth after six
months of TRT. One Other research printed in 2018 also found no proof of decreased penile measurement in males present process TRT.
Whereas this is a completely faulty perception, the question of – do steroids make your penis smaller?
Is kind of a paradox in the reality that some steroids can certainly adversely have an result on penile size,
but solely when taken under certain circumstances.
This article will seek to elucidate what kind of steroids could cause penile shrinkage and beneath what circumstances
can this well being problem happen. Total health and lifestyle elements
can even impact penis dimension.
Moreover, the steroid stack could make your muscles turn into stronger than ordinary with the same set of exercises.
Muscle manufacturing is mostly credited to testosterone or the “t-hormone” produced naturally by
the human body. Although you probably can increase its level by following a good food plan and
getting an sufficient amount of rest, the development in the hormone remains pretty restricted.
And the best steroids can provide a large boost to your testosterone ranges, thereby helping you put
on stronger muscles within a brief span. Whereas not every guy has blemish-free skin, in relation to steroids vs natural bodybuilders,
both pimples, acne scarring and even again zits can be an apparent signal of
steroid use. When it comes to steroid muscular tissues vs natural muscles,
gynecomastia is a typical aspect effect of anabolic steroids.
Whereas all of us want to obtain a rock-hard chest, there might be quite a distinction between hard-earned muscle and a build-up of breast tissue.
Rob Riches is a health personality who, like many others, claims that he’s 100%
natty and has been natural since the second he began his journey within the
trade. Fitness model came throughout a particular controversy when he allegedly failed a drug take a look at, main many to
question his earlier claims. In Addition To, Ryan has been weightlifting for almost 10 years, and he has never skilled any
steroid-specific signs that would present that his
physique is being supplemented by an external product.
Mr. Olympia primarily highlights the aesthetic
ripped look that is otherwise not current for overly bulked bodybuilders – one side that is certainly current within the
total build of Hadzovic. Simeon Panda is an English bodybuilder
with over 4.7 million fans on his web page and claims that he has certainly been natty all through his profession. He
presently possesses a Musclemania PRO card that led to him competing alongside personalities
such as Ulisses and Chul Soon whereas acquiring a sponsorship grant from MyProtein. The finest approach to know if a person is natty is by looking at them and taking a
glance at some options of their physique.
On the other hand, steroid bodybuilding entails the use of performance-enhancing substances, which can have critical implications for both
physical and psychological well being. Pure bodybuilding promotes
the development of physical and psychological
power through self-discipline and dedication. By adhering to a consistent training
routine and adopting wholesome consuming habits, people can construct muscle mass
and enhance their total physique.
These embody improved overall well being, reduced risk of damage, and enhanced longevity in the sport.
In the past, women would take steroids like Dianabol, Trenbolone, and Decaduro, this is in a position to not solely give
women extraordinary muscle gains it will also trigger some bad
unwanted effects. There are some girls who may care less concerning the corticosteroids side effects long
term (https://clinicadentalnoviembre.es/wp-pages/pages/como_aumentar_la_testosterona.html) effects of
steroids and are prepared to take risks in order to acquire extra muscle bulk.
In this article, I will cowl the advantages of
gaining muscle with steroids vs pure methods and the difference each one
has to offer. The most distinguished natural bodybuilding organization, INBA PNBA, screens its athletes via probably the most
strenuous drug testing standards – the World Anti-Doping Company (WADA).
Any athlete who fails a drug check throughout season or low season will be
stripped of their title and prize money and positioned within the Corridor of Shame.
Pure bodybuilding competitions exist to ensure
honest and equal opportunities for drug-free athletes.
So, the next time you think about the advantages of pure bodybuilding, bear in mind the often-overlooked
benefit of muscle maintenance. It’s not just about getting there; it’s also about
staying there, even when life throws its curveballs.
In the tip, it isn’t just about being big however
having the power to keep huge, naturally. One of the unsung benefits
of pure bodybuilding is the physique’s ability to keep up muscle
even in periods of lowered coaching depth or frequency.
Life can throw varied challenges at us, from demanding work schedules to non-public obligations, making it
difficult to maintain a rigorous coaching routine.
We left probably the most controversial for the last with Mike O’Hearn. As a four-time INBA Mr.
Natural Universe who has by no means flunked a drug check, O’Hearn’s natty status can never fairly be contested.
Nonetheless, you’ll find many articles and podcasts debating this, but Mike O’Hearn remains to be one
of the best pure bodybuilder to us. We’re starting our record
with the father of modern bodybuilding, Eugen Sandow. This is the athlete whose
determine is awarded the coveted Mr. Olympia trophy.
The distinctions go beyond just the use of performance-enhancing medicine.
From coaching regimens to recovery instances and potential
muscle positive aspects, the variations may be
important. To get a comprehensive understanding of
the contrasts and make an knowledgeable determination about your bodybuilding journey, discover our detailed comparison of
Natty vs. Steroids. In this text, we showcase a variety of the greatest and largest natural bodybuilders on the planet.
Not only are they ripped, however in addition they promote a healthy lifestyle without
using chemical substances or steroids to take action. The profound influence of
steroids on recovery is a key facet to consider within the comparison between pure and steroid bodybuilding.
He also defined further that reaching your full potential does not normally occur before
round a decade of training. He additionally warned individuals to keep away from juicing up if the aim is to
maintain up good well being and longevity.
For carbs, aside from these consumed around
exercises, we want a decrease glycemic load which would come
primarily from extra pure or unprocessed carbs to attenuate the insulin spike.
And as lengthy as it is elevated, fat mobilization is much less environment friendly.
Have as a lot as 50% of your day by day carb
intake before or during. The remainder of the non-protein caloric
consumption would come from fat.
Prednisone may be administered by a veterinarian as an injection, either intravenously
or into the muscle, in some emergency conditions, similar to anaphylaxis.
Prednisolone is the active metabolite of prednisone, converted
by the liver so that it could cross the cell membrane and performance properly.
Secondly, evaluate costs from different sources, both online and in brick and mortar shops.
They are great slicing compounds, which supplies with poor but high-quality muscle mass gains.
Another factor that influences the value is the type of steroid you gonna choose to make use of.
Such anabolics like Testosterone-ethanate or Dianabol are the cheapest steroids these steroids
value less. These are the fundamental steroids each bodybuilder begins steroid cycling with, thus the demand could be
very high. In the following section, we’ll talk about where you should buy
steroids in Australia and the potential prices of each
choice. The sort of steroid is the most vital factor that
influences its worth. Some steroids are more potent and have larger demand than others, resulting in greater prices.
However a drug with an identical name, prednisolone (Pred
Forte), is out there as an eye drop. This is because of significantly higher testosterone ranges, the male hormone
that is liable for increased ranges of aggression. Anabolic steroids can make customers more and more angry
and cause roid rage in sure individuals.
Tren and Tren Max are also known as ‘The Triple Ace’, value of anabolic steroids on the road.
Tren Ace can also be commonly generally identified as ‘stacks’ and bodybuilding ‘stacks’, value of
steroids per thirty days. The only distinction is that an ‘Ace’ is much weaker and extra
restricted than the ‘Stacks’ and ‘Max’ on steroids.
As the benefits solely last a few weeks, additional injections could solely be beneficial based mostly on your doctor’s approval.
An epidural steroid injection (ESI), also recognized as a block, is a non-surgical ache administration method which
delivers an area anesthetic instantly into the backbone.
Intra-articular knee injections with corticosteroids
(CS) and hyaluronic acid (HA) are frequently used for managing knee
osteoarthritis (OA). A examine found the 10-year conversion price
to TKA was highest in the HA cohort (31.6%), followed by the CS cohort (24.0%), and the non-injection cohort (7.3%).
People typically graduate to it after they’ve constructed a tolerance to
different opioids. You may have started using opioid ache killers after an accident, damage, birth, or dental process.
The bodily and emotional well being impacts of abusing heroin are far more damaging than the financial prices.
The Nationwide Survey on Drug Use and Well Being
revealed that about 948,000 People used
heroin within the final yr.
Nevertheless, there are also underground or black market steroids
which may come at a decrease value, but they could come with potential
risks related to authenticity and safety. Anavar, also
known by its generic name Oxandrolone, holds a big place within the
realm of bodybuilding steroid cycles. Renowned for its distinctive properties, this steroid has gained reputation among athletes and bodybuilders alike.
In this article, we’ll delve into the significance of anavar
buy in bodybuilding steroid cycle; Waldo, steroid cycles and explore the explanation why it has turn into a staple for
many fitness lovers.
Earlier Than the injection begins, you will lay flat
on an x-ray desk along with your back barely curved.
If this position causes any ache, then you
may be able to sit in a special position. The injection, given by an anesthesiologist or an interventional radiologist, is
commonly carried out within a specialty clinic, hospital or doctors’ workplace.
Our goal is to create an in-depth background of performance enhancing drugs from every background whereas building a community round discussion of these substances.
They might regain weight, see a slowdown in their weight reduction or develop new unwanted aspect effects.
One of the key causes it’s highly valued in bodybuilding
is its capability to advertise lean muscle gains without causing vital
water retention. Unlike another steroids, Oxandrolone’s anabolic
results primarily focus on constructing high quality muscle mass somewhat than extreme bulk.
This makes it a preferred choice for individuals aiming to achieve a toned
and outlined physique. Furthermore, this steroid’s mild androgenic nature contributes to its reputation.
Please observe that these are estimated prices and
it is beneficial to debate the specific costs with the healthcare
provider earlier than present process the injection. Get the facts on how much do steroid injections price, from varieties to trials, and insurance coverage
coverage. Prednisone is on the market because the brand-name medication Rayos and Prednisone
Intensol.
DHT levels are (very) low in skeletal muscle as it does
not considerably specific the enzyme. DHT also seems to be damaged down in skeletal muscle by inactivation to 3α-androstanediol by the enzyme 3α-hydroxysteroid-dehydrogenase (20, 21).
Certainly, adjustments in fat-free mass in response to
graded doses of testosterone are unaffected by DHT suppression with
the potent 5α-reductase inhibitor dutasteride (22).
The conversion of testosterone to DHT exhibits saturable Michaelis-Menten kinetics with an estimated in vivo Km value
of three.35 nM (23). Bioactivation by way of this pathway into a
more potent androgen doesn’t appear to happen for any of the other generally
used AAS (24). Results can vary, however many customers notice increased muscle
size and strength inside a couple of weeks. In research, individuals have shown significant muscle features within a 10-week period.
ultimate steroid cycle (Hallie) treatment can weaken your immune system,
making it easier so that you just can get an an infection. You should avoid taking prednisone in case you have a fungal
infection that requires oral antifungals. Topical antifungals is in all probability not
a difficulty, however always let your doctor know what medicines you’re taking earlier than beginning this
medication. Dr. O’Connor has over 20 years of expertise treating women and men with a historical past of
anabolic steroid, SARM, and PED use. He has been a board-certified MD since 2005 and supplies guidance on hurt discount methodologies.
Endurance train improves the redox system stability by stabilizing
the mitochondrial membrane, resulting in a discount of apoptotic results of ND in neural
cells [25]. Anabolic steroids also put men and women at
risk for much more critical concerns. Misuse has been linked to a higher threat of heart attack,
liver and kidney failure, blood clotting, and high ldl cholesterol.
Use in adolescence is especially bad as a rise in bone density too early may
find yourself in growth defects and may have cognitive consequences, together with
decreased attention and elevated impulsivity (commonly often recognized
as ‘roid rage’). In males, its levels increase throughout puberty to promote the development of male intercourse traits,
similar to body hair progress, a deeper voice, intercourse drive,
and increased height and muscle mass. Nonprescription doses are sometimes 10
to 100 instances higher than the doses healthcare suppliers prescribe to deal with medical circumstances.
This is why the unwanted aspect effects are normally extra severe than the unwanted effects of prescribed
anabolic steroid use.
Not surprisingly, gynecomastia is a aspect impact that can occur
as a end result of AAS use. In an uncontrolled multicenter contraceptive efficacy study, 271 males
acquired 200 mg testosterone enanthate weekly for a minimal
of 6 months (202). In contrast, the prevalence of
gynecomastia increased from 7% at baseline to 19% on the finish
of an AAS cycle in the HAARLEM research (39). Virtually
all of them had Simon grade 1 gynecomastia,
with one topic progressing from Simon grade 2 on the end of
the AAS cycle to grade 3 three months after the cycle, presumably as a end result of hypogonadal state that
adopted after cessation of use. While it remains
to be determined if and to what extent an AAS-induced
increment in blood pressure will increase CVD risk, it appears prudent to discourage use when an AAS user
meets the standards for hypertension. If a affected person continues utilizing
AAS long-term nonetheless, remedy appears smart.
Glucocorticoid motion in T cells and, in particular, in thymus,
the place naive T cells (that have but to come across antigen) develop,
has been the topic of intensive analysis but stays extremely controversial.
Double constructive cells, nearly all of the thymocyte inhabitants, are highly
delicate to glucocorticoid-induced apoptosis (Purton et al., 2004), effective at physiological levels of glucocorticoids (Jaffe, 1924).
A Lot in vitro evidence points to an important position for glucocorticoids in regulating T cell number, repertoire and performance, yet the in vivo evidence is discordant.
This topic has been extensively reviewed (Ashwell et al., 2000; Godfrey et al., 2001;
Jondal et al., 2004; Bommhardt et al., 2004; Herold et al.,
2006) and will solely be briefly discussed here. Nevertheless, transgenic mice with increased
GR in T cells (directed by the proximal Lck promoter or a doxycycline-inducible CD2 promoter) present
decreased thymic cellularity with increased thymocyte sensitivity to
glucocorticoids in vitro (Pazirandeh et al., 2002, 2005).
Finally, in 2 of 3 models during which GR density is reduced by expression of antisense GR either globally (neurofilament promoter) or in T cells (Lck
promoter), thymic cellularity is elevated,
albeit modestly (Pepin et al., 1992; Pazirandeh et al., 2002).
Thereby, AASs results are the outcomes of the amplification of testosterone and estrogens physiologic consequences.
A Number Of experimental human studies confirmed the affect of testosterone and AASs doses focus on their results.
In Accordance to a double-blind human research, low
dose administration of methyltestosterone is taken into account forty mg/d and
excessive dose is 240 mg/d [19]. In this research, 3 days’ administration of excessive doses of methyltestosterone led to neuropsychiatric effects.
One Other examine discovered psychological effects after 14 weeks of 500 mg administration of testosterone cypionate for
week [18].
The collective enhance in these serum markers ought to thus be interpreted as an indication of
liver harm, even within the presence of concomitant muscular train. Testosterone is a pure hormone that helps build muscle, enhance
power, and improve endurance. When athletes use anabolic steroids, they do it to boost their physical skills.
Sure, steroids are linked to elevated cardiovascular dangers, together
with hypertension, coronary heart illness,
and probably deadly issues like coronary heart attacks and strokes,
particularly in youthful users. Whereas steroids can undoubtedly speed up muscle progress and assist with fats
loss, the health dangers and potential long-term harm they convey typically outweigh
the advantages for many individuals. Nevertheless, with the best food regimen, exercise routine, and guidance,
it’s totally possible to achieve significant muscle
features without the use of steroids.
Many of those men are on anti-depressants due to the onset of Main Depressive Dysfunction. While talk-therapy and medicines to help these people, the truth is that we can only treat their melancholy.
Body builders, notably men, who select to take steroids, do so for a
reason – they work. It is essential that you know steroids are considered a Schedule III drug by the Drug
Enforcement Administration are subsequently illegal with no
prescription. Put On a medical alert tag or carry an ID card stating that you take prednisone.
Generally, we have found trenbolone’s fat-burning effects to be overpowered by its capacity to pack on muscle; thus, most will utilize trenbolone when attempting to gain lean mass.
Tren customers will ooze confidence because of incredible surges in exogenous
testosterone. That makes them very highly effective at treating circumstances starting from
bronchial asthma to most cancers.
This makes unwanted effects rather more extreme when you use them with no prescription. Some people “cycle” their anabolic steroid use by
taking the drugs for a while and then pausing for some
time before they begin them once more. Another technique known as “stacking” involves taking a couple of type of anabolic steroid at a time in hopes that this will make the
medication work higher.
As always, utilizing a supply of testosterone in your Winstrol
cycle may help alleviate these problems. Winstrol may be taken anywhere from 10mg every day to
60mg every day in either oral or injectable forms.
It is strongly discouraged for model spanking new customers to
start with the higher doses till you can gauge Winstrol’s potential unwanted aspect effects at the lower doses.
Women should take very low doses, starting at 2.5mg daily and by no means exceeding
15 or 20mg every day. Men who use Winstrol will expertise all kinds of results
just because Winstrol will be just one agent used in a male steroid cycle –
it’s not traditional for this steroid for use on its own by males.
Subsequently, your outcomes might be more determined by the other steroids you’ve included within the stack.
Biking, stacking, and pyramiding are meant to reinforce desired results
and minimize dangerous effects, however little proof supports
these benefits. HGH is a subcutaneous injection rather than an intramuscular injection like anabolic steroids.
This makes it simpler to inject for most people as you aren’t putting a needle into a hard muscle, which
can be painful. Instead, the HGH injection goes slightly
below the skin in part of the body, where you can lift
a small roll of fats to inject. The stomach area shall be most handy for most individuals due to the
thinner skin, which is less complicated to pinch with your fingers so you possibly can safely administer the injection. HGH,
when used appropriately at average doses for restricted intervals,
doesn’t include the extreme side effects that we see with most anabolic steroids.
Some folks will expertise negative impacts, largely joint ache, complications, and flu-type symptoms.
Whereas you’re not prone to fall ill when you use SARMs exterior their shelf life, they
will probably be much less effective over time as they lose their potency.
Ostarine ensures that joint and muscle pain is reduced or eradicated, and Ostarine might help reduce irritation. Endurance might be noticeably
enhanced, so your workouts will go longer and more intensely.
You might find you can push your self onerous and not want to cease figuring out after a quantity of hours –
injuries can nonetheless occur, so it’s important to set
a restrict. This stack won’t shut down your testosterone, so you won’t
even must do PCT afterward as you’ll with steroids.
As Quickly As you’ve reached the extent of hardcore, you in all probability feel able to try nearly anything!
When it comes to SARMs, abruptly stopping your cycle can potentially cause some points like lethargy, melancholy, pimples,
and hair loss in some girls. A normal Cardarine dose of 10mg day by day is normally more than sufficient,
although rising this to 20mg is feasible. Andarine has some side impact risks at higher doses, so starting at 25mg every day is a safe guess.
Girls additionally secrete small quantities of testosterone from the ovaries.
The secretion Classification Of Steroids androgens from the
adrenal cortex is inadequate to maintain male sexuality.
We give specific attention to the packaging and delivery
of all of your products.
Totally research respected sources, confirm product authenticity, and prioritize merchandise that undergo rigorous
high quality testing. Additionally, consult with healthcare professionals or experienced fitness advisors to make sure Anavar aligns along with your objectives, general
health, and individual circumstances. Adolescents and young adults ought to be taught about
the dangers of taking anabolic steroids beginning
in center school. Additionally, applications that educate different, wholesome ways to increase muscle dimension and improve efficiency may be helpful.
The pituitary gland isn’t a half of the mind but sits at its base, linked to it by a tiny stalk.
But if his analysis turns out to be successful,
it could usher in a new era of higher, quicker recovery from sports activities injuries.
Danish researchers are also trying into HGH as a remedy for
sufferers with tendinopathy—long-term tendon pain. Before 1985,
HGH had to be painstakingly extracted from cadavers, a practice that was banned when researchers decided that it could transmit a brain sickness just like mad cow illness into
patients. HGH surges throughout childhood and adolescence, but by the time you’re
forty, you’re producing solely about half as a lot as you had been at 20.
To separate reality from fiction, we interviewed patients, doctors, and researchers to
understand the advantages and promise of HGH. Join us on this
informative journey as we uncover the secrets and techniques to a
profitable Anavar purchase.
Right Here, pharmacology merchandise can be bought from well-known producers
such as British Dragon, Alpha Pharma, Vermodje, Geneza Prescription Drugs,
and lots of others. If you wish to buy high-quality steroids on the most affordable value, then HulkRoids.web is
the proper alternative for you. Injectable Steroids for sale within the USA on our website are a method of sports activities pharmacology.
These steroids are administered intramuscularly and are
manufactured by several totally different reputable companies.
They are produced in the form of solutions in either ampoules or
small vials. In order to properly administer an injection, you’ll need a syringe which is easily obtainable from your local pharmacy without a
prescription.
They carry critical risk/side effects that, if not correctly monitored, may be very harmful,
similar to blood clots and stroke. The drugs may be taken by mouth, injected right into a muscle, or utilized to skin as a gel or in a
patch. Bioidentical Hormone Substitute Therapy (BHRT) is a secure and effective option for addressing menopause
symptoms and different hormone-related situations.
HGH requires endurance to see results because it takes many months to see its full advantages.
HGH cycles are beneficial to be a minimum of
three months in duration and infrequently as
much as six months for optimum benefit. HGH is only
legal to make use of with a prescription for medical functions.
In the US and many other international locations, using,
shopping for, selling, or manufacturing HGH for efficiency
functions is not legal.
Unlike man-made versions of steroids, which may trigger an increased threat of
high blood pressure over long-term use, pure steroids are produced using pure sources and are secure for human consumption. Blood tests could be performed to examine the
particular person’s current level of testosterone and
determine whether or not it’s larger than regular.
Blood ranges of different reproductive hormones (such as follicle stimulating hormone [FSH], luteinizing hormone [LH]) can additionally be
measured. If the testosterone may be very excessive but the FSH and LH
levels are low, this offers evidence that the person is using anabolic steroids. How many or how usually you get a cortisone shot
depends on why you would possibly be getting it. Some individuals solely need one shot,
whereas others might have repeated remedy. However, you normally have to attend at
least three months between photographs, and most medical doctors restrict the shots to not extra than three in a single yr.
Steroids, whether or not anabolic or corticosteroids, come with a spread of potential unwanted effects and legal
considerations that need careful attention. Frequently taking anabolic steroids can lead to bodily and psychological changes in both men and women, in addition to probably dangerous medical circumstances.
Many people who use anabolic steroids are aware of the dangers of
taking them, and consider that by taking the medication in certain methods they’ll avoid unwanted facet effects.
Or they may take further medicines to try to counter
the unwanted effects. Some types of steroids are commonly used for medical
remedy. For instance, corticosteroids may help
people with asthma breathe throughout an assault.
This is not meant to be read as an article condoning steroid use.
A 2018 research within the Digital Journal of Ophthalmology found that adults who used inhaled budesonide for six months or more had significant increases
in inner eye strain. Many individuals with osteoporosis do not even notice they’ve it until they expertise
an unexpected bone fracture. Anybody experiencing this explicit side impact ought to search medical advice.
He cautioned that it could be very important notice there’s a difference between the prescribed steroids discussed in this research in comparison with those taken by athletes.
Now a staff of scientists from Leiden University Medical Heart in The Netherlands has found evidence suggesting the use of prescribed steroids
causes structural and volume adjustments within the white and gray matter of the brain. Steriods are solely permitted for folks with sure medical conditions on prescription by their medical practitioner.
Although testosterone is called a male sex hormone, it additionally
occurs naturally in women, but in a lot smaller quantities.
One specific oral steroid (dexamethasone) is often used
in the path of the end of pregnancy if there is a risk of a
pre-term birth as it could help the child’s lungs to mature more rapidly and
improve the baby’s probability of survival. Nonetheless, in case you
have taken excessive dose steroids (40mg for greater
than 1 week) or have been on them for longer than three weeks, you have
to never cease the steroid all of a sudden as this
might trigger a crisis situation leading to coma and presumably death.
When all these processes happen, bigger
muscle tissue are gained more shortly, which leads to higher performance.
Natural steroids differ from synthetic steroids in their methods of manufacturing and safety of use.
Furthermore, it has been proven that the higher the depth of
the exercise, the greater the increase in hormone levels [21].
D-aspartic acid (DAA) is an amino acid that’s primarily found within the pituitary gland and testes, and
it’s not used to build proteins however instead
regulates the production and launch of testosterone in the body [19].
There may be other fees added to that amount though to
cover different prices. Ask your doctor’s workplace beforehand what the fee
shall be so that you’re prepared. Cortisone photographs
are normally given in a doctor’s workplace or clinic.
It could be part of a routine visit or a special appointment only for the injection. You would possibly feel
some ache when the needle is inserted, however folks usually report feeling more strain than pain. Stelara (ustekinumab) is used to deal with Crohn’s disease, ulcerative colitis, plaque
psoriasis, and …
In such cases, the dose might need to be lowered, or the formulation switched.
This article will explain the 4 most common unwanted aspect
effects of inhaled corticosteroids. Inhaled steroids work
by mimicking cortisol, a hormone produced by the physique that
usually reduces inflammation (swelling of tissues).
Prednisone is a corticosteroid medicine used to lower inflammation. It is usually prescribed to deal with rheumatoid
arthritis (RA), gout, lupus, persistent decrease again ache, and knee
osteoarthritis. To reduce the chance of side effects, take steroids for the shortest possible time and lowest effective
dose to deal with a condition, says McNeely.
The longer you are on steroids and the higher the
dose, the more probably you are to have unwanted effects.
However, side effects are extraordinarily common even on brief doses and for otherwise healthy people.
“Steroid medicines at high doses or long run remedy multiple to 2 weeks may be very harmful with some permanent problems or unwanted side effects,” says Rivadeneyra.
When you take corticosteroids, they mimic cortisol, a hormone that your physique naturally releases in response to
emphasize like harm or illness.
It is turning into nicely established that the intestine microbiome has a profound impact on human well being and disease.
In this evaluate, we explore how steroids can affect the
intestine microbiota and, in flip, how the gut microbiota can influence hormone levels.
Inside the context of the gut microbiome-brain axis, we focus on how perturbations within the intestine microbiota can alter the stress axis
and behaviour. In addition, human research on the potential role
of intestine microbiota in depression and nervousness are examined.
Finally, we current a few of the challenges and important
questions that have to be addressed by future analysis in this exciting new area at the intersection of
steroids, stress, gut-brain axis and human health. Prednisone can also be prescribed at the
lowest possible dose to convey the illness underneath control.
Nonetheless, for some circumstances, this isn’t possible, and better doses are needed.
Different steroids are normally modified variations of testosterone, as well as DHT.
While anabolic steroids are potent androgens with a powerful anabolic nature, their androgenic activity is usually very robust, leading to a bunch of
undesirable unwanted effects. To reduce the chance of
unwanted effects, we completely reviewed the protection of the elements in every complement.
We solely advocate authorized steroids with components that are
safe when taken as directed.
This more superior and experimental method is generally not really helpful for newbies.
You’ll want to know what you’re doing and, ideally, have prior experience taking SERMs.
It’s not without dangers, but many individuals successfully run SERM + SARM with good results.
Suppose aspects related to hormones, ldl cholesterol, cardiovascular, liver, kidneys, blood count,
and other values change considerably. In that case, you’ll need to modify your SARM cycles accordingly (or even cease using them altogether, in excessive cases).
You might assume you are feeling nice, however you will have no method of knowing what effects SARMs have over
the lengthy run. Cardarine will make your physique use fat as gas,
leading to probably very fast fat-burning soon after starting this cycle.
Moreover, taking MSM (Methylsulfonylmethane) at 1g daily and
a few tablespoons of Collagen Powder every day will significantly profit joint health.
People who comply with this sample of steroid usage declare that it has an impact of making
the body modify to excessive dosage at the proper time.
The drug free interval that follows is believed to help the physique synchronize with the normal hormonal functioning of the
physique. Clearly, this is something which has not been proofed scientifically and therefore nobody is bound whether or not it is true or not.
Take 4 capsules of Testomax earlier than breakfast, three capsules
of Trenorol, and DecaDuro forty five minutes before exercise.
It is finest to take all 4 supplements collectively within the method prescribed by the
internet site. Oral steroids, particularly, are recognized
to be hepatotoxic (toxic to the liver). Compounds
like Dianabol, Winstrol, and Anadrol are alkylated to survive liver metabolism, but this mechanism can injury the liver.
Common liver perform checks, hydration, and probably the usage of
liver assist dietary supplements (such as milk thistle or
TUDCA) are often recommended to mitigate risks. I am a muscular guy with much
attention and recognition for my physique.
Testosterone is the primary male intercourse hormone answerable for muscle development and energy.
For anybody starting a bulking cycle, Testosterone supplies the
muse for muscle growth, restoration, and strength gains. Dianabol not solely
promotes muscle growth but also enhances power and general
efficiency. This allows beginners to carry heavier weights
and push by way of more intense workouts, which finally ends up in even larger muscle positive aspects.
Consulting with a healthcare professional ensures you’re utilizing the right supplements safely and successfully.
Large ester base testosterones may also be the simplest to make use of corresponding to
Testosterone Cypionate and Testosterone Enanthate.
Some can also discover additional steroids like Anadrol or Dianabol to be helpful.
In fact, the combination of Deca Durabolin and
Dianabol along with some type of testosterone is doubtless considered one of the oldest and most
popular steroid cycles of all time. For spectacular muscle progress and bulking body, Deca-Durabolin is ideal
whereas combining with other anabolic steroids.
Make positive to observe the Publish Cycle Therapy with all the steroids you are using.
You can get the maximum results by taking a 100 mg dose of Anadrol every day.
Not only for superior customers however for intermediate, this bulking cycle is helpful to achieve
their health goals. The correct dosage of Dianabol
can provide the most bulking results.
Whether you’re training for a marathon or looking to improve your physique, authorized steroid
alternate options can help you reach your performance goals safely and naturally.
Yes, many legal steroid options are often available
on the market at discounted prices and provide free delivery.
With CrazyBulk, you are offered free delivery worldwide, with a buy-1-get-2 deal on their premium
authorized steroid stacks, and Brutal Pressure supplies free transport for all orders
over $199. By shopping for steroid stacks, you also get a discount on each complement,
making it a less expensive approach to improve your performance.
We have seen Anadrol add 50+ kilos to compound lifts (on its
own). Anabolic Does Steroids Stunt your growth are unlawful with no prescription in plenty of international locations,
including the us, UK, Canada, and Australia. Possession or sale with out medical
justification can result in authorized consequences.
However, legal alternatives (natural supplements) can be found over the counter.
As Quickly As more appropriate breast cancer medicine were developed, the use of Masteron declined and ceased by the late Nineteen Eighties.
Even though this steroid nonetheless has FDA approval, it has no present medical
use or manufacturing within the US or other Western nations.
With bodybuilders in the 1970s turning into conscious of Masteron’s capabilities
as a performance-enhancing drug (PED), its use took off.
It remains a reasonably well-liked steroid
(although removed from the most extensively used).
Prednisone treats many various situations corresponding
to allergic issues, skin situations, ulcerative colitis, arthritis, lupus, psoriasis,
or respiratory disorders. ‘HGH gut’ is usually noticed
in IFBB bodybuilders, which can be attributed to the enlargement of inner organs such because the intestines and liver (9).
Clenbuterol notably will increase coronary heart fee in our sufferers,
which can be supported by research (8). This occurs because of
extreme adrenaline production, placing pointless strain on the guts.
Crazy Bulk’s Anadrole formula is synthesized to mimic the anabolic results of Anadrol however without
the tough side effects. Dianabol was first created to be considerably extra anabolic than testosterone however with less androgenicity.
A widespread psychological aspect impact of AAS use
is muscle dysmorphia, by which customers turn out to be preoccupied with having a muscular physique (31).
Steroids are very effective at treating sure inflammatory circumstances.
Nonetheless, the medicine are potent and might produce undesirable unwanted aspect effects,
similar to weight achieve. We have evidence to counsel
that Loopy Bulk’s authorized steroids do work, primarily based on our anecdotal expertise
of patients attempting their supplements. Loopy Bulk also has thousands of
verified customer reviews on third-party platforms and optimistic suggestions on social media profiles.
There aren’t any banned substances in any of Crazy Bulk’s legal steroids; due to this fact, customers will cross any kind
of drug take a look at whereas taking them. Authorized steroids haven’t triggered any
virilization-related unwanted side effects in our female sufferers.
Nonetheless, we suggest buying from a trustworthy model to guarantee you are getting real authorized steroid options (instead of a spiked
product).
Measure liquid drugs with a special dose-measuring
spoon or medicine cup. If you do not have a dose-measuring system,
ask your pharmacist for one.
Anavar’s reputation stems from it being suitable for beginners, as
it is very nicely tolerated. Girls use it as a
outcome of it hardly ever causes virilization unwanted
facet effects and is even utilized by skilled execs
due to its muscle-building and fat-burning results. With
bodybuilders eager to look as ripped as attainable, trenbolone is a well-liked
selection, as customers is not going to endure from smooth, puffy muscle tissue
or bloating. For best steroid alternative for mass
outcomes, we discover that Anadrole should be combined with other
authorized bulking steroids, such as Decaduro, Testo-Max, D-Bal, and/or Trenorol.
The legal status of AAS varies by country and area, though they’re categorised as illegal in most locations if
used for non-therapeutic functions. Though most sporting federations
ban AAS, some athletes feel the risk of getting
caught is price the benefits. In the world of sports, athletes are continually on the lookout for ways to get an edge over the competition.
At All Times seek the guidance of your healthcare provider to make sure the data
displayed on this web page applies to your private circumstances.
Nplate is used to prevent bleeding episodes in people with persistent immune thrombocytopenic purpura …
While utilizing this drugs, you might need frequent blood checks at your physician’s office.
Steroid treatment can weaken your immune system, making it simpler for
you to get an an infection or worsening an an infection you already have or have lately had.
Inform your physician about any sickness or
an infection you may have had throughout the previous
several weeks. You mustn’t use this treatment if you’re allergic to prednisone, or if you have a fungal infection that requires oral antifungal
treatment.
They have been shown to extend muscle mass, which leads to elevated
pace and energy output (5). To increase muscle strength and energy
past the pure restrict, some individuals turn to substances like anabolic-androgenic steroids (AAS).
Prednisone is a corticosteroid medicine used to lower inflammation and hold your immune system in verify,
if it is overactive. Clenbuterol just isn’t a steroid; nonetheless, it is often stacked
with chopping steroids to ignite fats burning.
Loopy Bulk’s Clenbutrol replicates the stimulative effects of Clen to spike a user’s metabolism.
Nonetheless, by means of pure muscle achieve, we rank trenbolone among the finest
bulking steroids. Based on our exams, Anadrol is presumably the worst anabolic steroid for
the guts and liver.
Anabolic-androgenic steroids (AAS) are an artificial form of testosterone, which is the first male intercourse hormone (1).
Usually, the upper the dose of the steroid and the
longer you’re on it, the extra doubtless you would possibly be
to come across weight gain. Quick courses of some days to a few weeks don’t usually produce many unwanted
facet effects. These drugs are highly efficient at lowering irritation, but in addition they have some troubling unwanted effects.
Authorized steroids, or steroid options, are FDA-approved formulation that mimic
the effects of anabolic steroids. HGH is a protein hormone
that’s naturally secreted by the pituitary gland.
These are naturally occurring hormones produced within the adrenal glands situated on high of your kidneys (33).
The solely approach to obtain and use AAS legally could be to have them prescribed by a medical
skilled for a certain situation, similar to low testosterone or a muscle-wasting disease.
The anabolic-to-androgenic ratio varies between several varieties of AAS, which can have an result on antagonistic reactions
as properly. Anabolic refers to muscle growth properties, whereas androgenic refers back to the
promotion of male sex traits (16). Whereas AAS use is not the only method to preserve muscle mass, it could benefit these populations.
In aggressive sports, steroid dosing tends to be fairly conservative to avoid detection. Muscle mass just isn’t the principle concern here,
as they’re used extra for recovery and increased energy output (6, 7).
Although Clenbuterol is used by many bodybuilders to great impact when attempting to lean out and
get ripped, it is not FDA-approved for humans.
From a security perspective, we find Winsol to be
the better possibility, with not certainly one of
the above unwanted aspect effects being a trigger for concern. The downside of
Anavar is that it is rather expensive, due to the issue in manufacturing this compound and high demand.
Therefore, the financial price of experiencing average gains is very high.
Deca Durabolin is a well-liked injectable bulking steroid, typically used in the low season.
We found that a number of people had been saying constructive
things about Loopy Bulk and were not directing folks
to the website (thus showing to be genuine or unbiased comments).
To do this, we entered ‘#crazybulk’ and #dbal, together with different
product names, into Instagram, Twitter, and Fb.
Pharmaceutical HGH is formulated in an authorized
lab and is sometimes sold (illicitly) by
somebody who has been medically prescribed this drug.
UGL HGH is usually formulated by someone with zero medical experience or qualifications and
synthesized in a non-certified lab (usually their very own home).
UGL HGH is considerably cheaper; nevertheless, it poses extra
risks because of hygiene points or possible contamination. With UGL
merchandise, there is additionally a chance of the product being diluted or counterfeited.
We see testosterone taken by newbies as a first steroid cycle,
helping them to construct large quantities of muscle whereas decreasing subcutaneous fat.
Nevertheless, if newbies do not want to expertise vital
muscle features but as a substitute milder will
increase in hypertrophy (size), the above cycle could also be
taken.
Secretion of hGH by the pituitary gland is pulsatile,
resulting in extremely fluctuating ranges in the circulation. Furthermore, hGH
is considered to be a stress hormone regulated by elements similar to
sleep, nutritional standing, train, and emotion. Thus, there is high intraindividual and interindividual variability in the secretion of hGH.
Quantifying the hormone itself is not adequate to detect exogenous rhGH.
Extra stable serum variables implied within the organic cascade produced by hGH secretion, or a
doping software, could be the route of successful detection of hGH.
The growth issue IGF‐1 and a few of its transport proteins (IGFBP‐3), have been proposed as attainable candidates for oblique detection of hGH doping.
But the interindividual variability is quite excessive and makes it exhausting to exactly outline a quantitative cut‐off degree.
Examples of catabolic steroids include prednisone, cortisone, dexamethasone,
and hydrocortisone. The major advantages of catabolic steroids are their capability to reduce
inflammation, management autoimmune responses, and handle chronic illnesses effectively.
Nonetheless, extended use can result in unwanted side effects similar to muscle losing, decreased bone density (osteoporosis), a weakened immune
system, weight achieve, and fluid retention. The most effective methods to naturally enhance HGH
and testosterone levels revolve primarily round way of life
changes. The essential ones include optimizing your bodily exercise, vitamin and sleep.
Overall, studies suggest that stacking two therapies can lead
to important enchancment in muscle mass, physical performance, and fats loss.
These tests might include blood work to measure hormone levels, provocation exams,
bodily examinations, and medical histories.
Alternatively, persistent increased IGF-I may very well
delay myofibre maturation. Protein synthesis additionally depends
on the power status in the muscle as it is an ATP-dependent
course of, and subsequently can additionally be regulated
by the AMP-dependent kinase AMPK (Bolster et al., 2002).
Treatment of rats with an AMPK-activating drug leads to a discount in protein synthesis accompanied by a lower in activation of mTOR,
p70S6K and 4E-BP1.
Anavar, also referred to as oxandrolone, is often considered one of the safest steroids for bodybuilding,
notably for those new to anabolic steroid use. This oral steroid has gained reputation because of its comparatively delicate nature and decrease threat of
unwanted side effects in comparison with different anabolic steroids.
Anabolic steroids have lengthy been a topic of curiosity in bodybuilding circles,
with many in search of to enhance their muscle-building potential.
However, the pursuit of a muscular physique should not come at the price of one’s well being.
This guide aims to explore the safest steroid choices
for those contemplating their use in bodybuilding, addressing both effectiveness
and potential risks. The word “anabolic” means muscle-building whereas “androgenic” means to
the characteristics of males they’ll promote (such as facial hair increasement and
coarse voice).
Testosterone is a hormone that performs an important role in women and
men, although it’s found in a lot higher levels in males.
In men, testosterone is made in the testes, and in girls, it’s produced in smaller amounts by
the ovaries and adrenal glands. When testosterone ranges
drop beneath the normal vary, the results may be noticeable and often problematic.
It’s Going To be irresponsible to say that steroids
are better than HGH or vice versa. As A Substitute, it is best to show to legal alternate options in case
you are in search of practical assist in your fitness targets.
The authorized options are safer and will assist you to obtain your dream physique
naturally. Nonetheless, regardless of the differences in how they work and what
they target, both steroids and HGH are illegal and may
have harmful side-effects.
There’s nothing mistaken with enthusiasm, but diving in with zero
data or concept of what might go incorrect, let alone HOW you ought to be utilizing steroids, is
going to turn your steroid expertise right into a residing nightmare.
The more superior scientific evaluation methods now out there made this
attainable – and it sends an alarm out to anyone who thinks a negative end result now means you’re
in the clear endlessly. This ranges from injecting into the
wrong spot or too usually in the identical
spot, causing pain, irritation, or infection, to rather more serious issues like accidentally injecting into a
vein and putting your life at risk. The research found that
blood was aspirated, indicating a vein was pierced by the needle;
however, the person continued to inject.
There are so many components that it’s beyond your power to control them all.
Still, you possibly can go a great distance in precisely predicting how long you may be susceptible to steroid detection simply by understanding how each
issue influences the detection timeframe. Like all areas of medication,
advances in performance drug testing are constantly ongoing and beneath research.
This brings about new techniques that may or might not become normal and widespread in drug testing worldwide.
But the primary problem is that the amounts we produce are typically not massive enough to present any noticeable advantages in relation to physique, athletic
performance, and restoration. This is why HGH is commonly stacked with
testosterone steroids for massive muscle gain corresponding to testosterone enanthate.
To help customers understand the risks and understand the way to use them most
effectively.
Anavar is superb for fat-burning and drying out the physique,
though, so if that’s your aim, it’s the go-to compound.
Commonplace steroid cycles are sometimes the start line for model
spanking new users however are additionally a staple in the technique of experienced bodybuilders.
The outcomes achieved over a 10–12-week commonplace cycle can be fantastic (provided you’re employed hard in the gym).
The D-Bal Max formulation contains a blend of natural, high-quality components that every contribute to those benefits.
Some ingredients give consideration to protein synthesis, or the method of
rebuilding muscle damage after exercises. Increasing your body’s pure protein synthesis helps
you develop muscle gains at a sooner fee
by reducing your muscles’ restoration time. The results
of Trenorol sometimes become noticeable after a quantity of weeks of
consistent use, relying on factors like particular person response, exercise routine,
and diet. Users can count on to see enhanced muscle gains, improved
strength, and overall improved physique composition, along with other potential benefits similar to increased vascularity and faster recovery.
Testo-Max rounds out the stack by naturally boosting testosterone levels.
By elevating your physique’s most anabolic hormone, Testo-Max amplifies the
effects of the opposite dietary supplements. It Is like including rocket gas to your muscle-building efforts, enhancing energy, libido, and
overall efficiency. Pound for pound some of the potent
anabolic steroids you ought to buy, however discovering
a safer different is essential to our total well being.
A balanced food plan, regular exercise, and enough relaxation are also essential for attaining your fitness targets.
Nonetheless, it is also used illicitly by some why do athletes take steroids (Fairhuurvoorverhuurders.nl) and
bodybuilders to reinforce efficiency and physique.
All The Time seek the assistance of with a healthcare skilled earlier than beginning any new complement regimen, and remember that supplements usually are not an alternative to a balanced
food regimen and regular train. Equally, Magnesium,
which promotes fat loss for better muscle definition by growing metabolisms, is current within the supplement in a quantity of 200 mg.
Equally, Tribulus Terrestris, a dietary complement that claims to reinforce muscle
building, is current in the complement in a amount of seventy five mg.
Nevertheless, some constructive outcomes have been obtained with 750 mg -2.5 grams of this
substance.
A 2018 research discovered that males taking 500mg of ashwagandha every
day for 12 weeks obtained stronger in comparability with those that didn’t.
It not only boosts muscles but also enhances overall bodily
efficiency, making it a key player in muscle-building supplements.
For those wanting muscle gains without steroid risks, natural supplements are a great route.
Decisions like creatine, ashwagandha, and tribulus terrestris are well-liked.
It additionally capabilities as an anabolic steroid essential for protein synthesis and muscle growth.
If you wish to increase your strength and turn into stronger, then a one rep
max calculator is the right device for you.
A one repetition max calculator helps you
determine your true max reps before even beginning your exercise routine.
It will assist increase up your performance and forestall accidents whereas doing so!
General, your joint well being while on AAS
must be a first priority so your coaching just isn’t hindered.
Cautious monitoring of AAS dosages and cycles, beneath the guidance of a healthcare professional, can help minimize adverse results on joint health.
Different AAS either have a impartial impression on the joints or enhance their power and healing by boosting collagen manufacturing, with Nandrolone being
a main example. Typically, blended dietary supplements seem secure,
with a low risk of main unwanted facet effects. Folks could
benefit from understanding with a pal or hiring a private coach
to assist them attain their targets. A number of fitness apps can also present steerage and assist for healthful train habits.
They could overlook the significance of their train habits and vitamin, expecting dietary supplements to make up
the difference.
Vitamin D performs an important position within the immune system, so taking a complement may be useful to general muscle well being.
Vitamin D is an important element of muscle well being, with research showing that it helps muscle gain and muscle
recovery. Additionally, zinc boosts immunity, improves
focus, and assists in recovering sooner after exercises.
Turkesterone and ecdysterone are among the best dietary supplements for these wanting to stay natural.
Anabolic steroids are not generally protected for human consumption outdoors the confines of medicine.
Equally, there are natural bodybuilders content with competing in low-profile competitions with minimal prize cash.
We have patients desirous to be as muscular as attainable for cosmetic purposes,
and they are happy with risking their well
being and living a doubtlessly shorter life.
These results are highly wanted in the course of the ultimate phases of contest prep or photoshoot cycles.
There are very few differences in the way in which that men and women process steroids – they may deliver the
same results. Nonetheless, the true downside comes with
the side-effects – for which ladies are much more prone.
With an oral suspension being potential and
a great degree of digestible absorption, Winstrol additionally makes for a prime candidate for legal alternatives.
If you value your health – it might be the better path to take whereas readjusting expectations for your physique.
Considered by many to be the best stack for general physique health and bodily positive aspects, this stack will not
solely assist you to attain your targets sooner than ever before, but will probably surpass them.
You will most likely never work out again with
out together with this stack in your coaching
program.
Moreover, proper medical supervision and steerage from a certified healthcare professional
or experienced bodybuilder are important when endeavor any anabolic steroid cycle.
This cycle is frequent among novices, starting with a conservative 15 mg/day dose and lasting 6 weeks.
We have found this to be essentially the most efficacious oral cycle for getting ripped.
Trenbolone has diuretic properties, causing extracellular water to be flushed out.
This is the water that collects outdoors of the muscle tissue, causing a clean and puffy
look. Thus, with this fluid removed, trenbolone
users can develop a more toned and dry appearance.
LNCaP cells were seeded in 24-well plates
at a density of 6 × 104 cells per nicely. After 24 h,
the cell culture medium was changed with a medium containing 10% FBS that was pretreated with dextran-coated charcoal to remove endogenous hormones.
After a further 24 h, the ARE-Luciferase and Renilla plasmids were transfected into LNCaP cells with lipofectamine 2000 based on producer’s instructions.
Twenty-four hours later, cells have been handled with each of
the testing compounds.
In comparison, aromatization of androgens to oestrogens is quantitatively
much less essential in males, and the blood levels of aromatic oestrogens are normally very low (de Ronde et al.,
2003). In addition, ERs present in varied male tissues (e.g.
pituitary, breast and prostate) are functionally active and may be readily activated by administration of an oestrogen to
elicit sturdy biological results. The endogenous oestrogens have important organic features in males as properly as in women. Because
17β-oestradiol and oestrone are also shaped within the male physique,
these fragrant oestrogens are typically considered answerable for exerting the required
oestrogenic functions in the male. When using Primobolan for
recomp, the aim isn’t maximum weight gain or excessive fats loss—it’s sustainable physique refinement.
That means your dosing strategy ought to prioritize stability, muscle retention, and power stability,
not aggressive anabolic overload.
The main purpose females use AI like Letrozole could be to mitigate water retention, especially for competitive bodybuilders or physique athletes, where that is most essential.
As post-menopausal girls no longer produce estrogen from the ovaries, AIs
are sometimes only utilized in these older women. At its core, Letrozole’s most important mechanism of
motion is to behave as an inhibitor to stop androgens from being converted into estrogen. This makes it what’s
often recognized as a “non-suicidal AI.” And it’s this action that appeals to any male using anabolic why steroids should Be illegal,
that are able to aromatizing. Letrozole combats a wider vary of estrogenic side effects brought on by steroids, with the 2 main ones being gynecomastia
and water retention.
Primobolan is famend for helping athletes retain muscle mass while chopping.
In a calorie deficit, the chance of dropping hard-earned lean tissue is high—but Primobolan’s capability to take care of nitrogen balance and inhibit glucocorticoids offers it
a distinct anti-catabolic advantage. Blood cholesterol
was comprehensively and systematically measured in the BIG 1–98 trial under non-fasting conditions every 6 months (Thürlimann et
al, 2005). At a imply follow-up of 25.eight months, there
was extra hypercholesterolemia within the letrozole
arm (43.6, vs 19.2% within the tamoxifen arm), but this AE was predominantly low grade.
Importantly, median adjustments in levels of cholesterol remained stable at 6,
12, and 24 months (0, zero, and −1.8%)
in the letrozole group however decreased within the tamoxifen group at
every evaluation (−12.0, −13.5, and −14.1%).
These results assist a greater lipid-lowering impact of tamoxifen quite than a detrimental impact of
letrozole.
In reality, anabolic steroids normally should be avoided if the individual doesn’t possess a healthy
cholesterol reading. For those that are wholesome enough for supplementation it is going to be extremely important
that they live a lifestyle that’s conducive to sustaining correct levels.
Massive amounts of saturated fats should be prevented,
as properly as usually unfriendly cholesterol meals.
The supplementation of omega fatty acids is also extremely really helpful, as
is plenty of cardiovascular activity. It is still a matter of discussion whether or not variations between ninety five and 99% inhibition of aromatisation in vivo translate into significant completely
different scientific response rates and instances.
Did not reveal a major distinction between the 2 day by day doses, you will need to
point out that 8 out of 10 patients experienced a better aromatase inhibition whereas on the 10 mg o.d.
In distinction, the corresponding examine evaluating letrozole 0.5 mg o.d with 2.5 mg o.d.
Due To This Fact, older bodybuilders with existing achy joints ought to be significantly wary of Winstrol.
MCF-7 and LNCaP cell traces were obtained from ATCC (Manassas, VA, USA) and cultured based on the instructions of
the suppliers. For cell proliferation assay, cells have
been cultured with medium containing 10% of dextran-coated charcoal-stripped fetal bovine
serum (FBS) for 3 days and then have been seeded in 96-well plates with
104 cells per nicely.
As for Trenbolone, it doesn’t aromatize at all, but its moderately strong progestin nature
could make gynecomastia a possibility relying on the individual’s sensitivity.
When use is coupled with an aromatizing steroid, this can tremendously enhance the percentages
of gynecomastia. Regardless of the steroid in query, Aromasin can supply protection from the estrogenic effects.
One of Trenbolone’s notable advantages is its fat-burning functionality, which sets
it aside from different anabolic steroids. Tren promotes lipolysis
(fat breakdown) whereas preserving lean muscle,
enabling users to attain a leaner and extra outlined physique.
Delaying PCT can prolong the suppression of pure testosterone production and enhance the chance of unwanted effects.
When it involves utilizing Oxandrolone (Anavar) for bodybuilding functions, it is
essential to remember of the potential unwanted effects.
Whereas generally thought-about one of the milder anabolic steroids, Anavar can nonetheless
carry some dangers if not used responsibly. We find that when somebody cycles off
trenbolone, they usually regain this water weight. The majority of muscle positive aspects and fats loss could also be maintained post-cycle if users proceed to carry weights and eat sufficient quantities of calories.
None of what you read on this web site is medical recommendation, however its still highly suggested that Trenbolone be prevented until you acheived considerably significant expertise with steroids.
There are many advanced steroid users that still keep away from Trenbolone even at
superior stages. One type of Tren should NEVER be stacked with one of the
different two forms of Tren, as doing this is
in a position to multiply its unwanted side effects, and as you’ll see below, these
side effects are intense enough already (to start with).
Men sometimes aim for a low physique fats proportion of under
4% with as a lot lean muscle as attainable. However don’t
be discouraged if you’re physique fats % sits around 12% – you’ll still see Winstrol’s effects.
Winstrol is exclusive among the many steroids that exist in each oral and injectable types in that Stanozolol is C17a alkylated in each formats.
Women who use Winstrol should stick to 5mg/day and carefully monitor unwanted facet effects.
The benefits and constructive results of Winstrol have to be balanced towards the negatives,
together with its excessive stage of hepatotoxicity. This is why
most people use Winstrol sparingly and at times
when it will be of maximum benefit – corresponding to earlier
than competitions. Each types of Winny (oral and injectable) comprise a methyl group attachment on the 17th carbon, making them C17-aa
steroids that can resist liver metabolism.
Steroids are popular amongst bodybuilders for their muscle-enhancing properties.
Many athletes seek them to gain a aggressive edge and speed up muscle development.
Purchasing steroids on-line presents comfort and a wide selection of options.
Nonetheless, it’s crucial to research and select
reliable suppliers to keep away from well being risks.
Thus, controlling estrogen ranges is important for sensitive customers to forestall the accumulation of feminine breast tissue.
Winstrol does not have any estrogenic results,
so there is no fluid retention, and that’s typically the
place an increase in blood strain can impression steroid users.
So, whereas Winstrol won’t improve your blood stress through estrogenic
activity, its negative impression on the cardiovascular
system can potentially improve blood stress.
Androgenic unwanted effects are potential with Winstrol, but these will impression everyone in one
other way. Some guys can use Winstrol with virtually no adverse androgenic
effects at all. Nonetheless, if you’re one of the unlucky ones with genetics working
against you, then you can anticipate to be dealing with a
few of these well-known steroid side effects brought on by Winstrol’s androgenic activity.
It’s harsher than some of the other in style cutting steroids, and most
customers will battle with one sort of facet impact or
another. Due to Winstrol’s substantial liver toxicity (including the injectable version), we need to be cautious when stacking
it with some other C17-alpha alkylated AAS and restrict
the cycle to just a few weeks.
In addition, the unique steroids are packaged in giant bottles and sealed in a
plastic film. Anabolic steroids in tiny bottles are bought in boxes of one bottle each.In certain conditions, the writing on a counterfeit bottle does not match
its contents. In the best-case situation, the drugs is impartial (placebo); in the worst-case scenario, it includes the active ingredient however not within the quantity that’s specified
on the label. This is particularly harmful to women who use high levels
of steroids, which is extraordinarily undesirable.
Trenbolone isn’t C-17 alpha-alkylated, so it’s not thought-about
a hepatotoxic steroid in reasonable doses, unlike Dianabol.
Thus, if users are anxious about liver injury, trenbolone is the much less deleterious choice.
Dianabol is often used in bulking cycles as
a outcome of its positive results on muscle and power.
If a beginner administers Dianabol in an affordable dose, being 10–20 mg+ per day
(for men), they’ll expertise notable increases in muscle measurement and strength.
It acts as a stimulant, so potential side effects will affect everybody
in a different way – put together to change the dose if required and
monitor your blood pressure all through the cycle.
The quantity of weight loss will rely in your present weight and the way exhausting you’re dieting and coaching – dropping 2-4lbs of physique fat per week is an achievable objective with this cycle.
Kickstarting a cycle is usually carried out in a mass-building off-season cycle.
Sustanon 250 just isn’t inherently the most effective form of testosterone,
as some people declare. We don’t see it construct more muscle or energy than other esters;
it is only a completely different blend. Testosterone suspension requires two injections a
day, which is not ideal for a beginner. Furthermore, suspension, in our
expertise, is certainly one of the worst steroids to inject, as
it requires a big needle (a newbie’s worst nightmare).
Because online retailers are drop shipping their merchandise, you could receive different
packages should you buy from multiple completely different labs on the location. This can leave
some inconsistencies with the labs not producing quality products all 12 months
round. What the traditional procedure is, is the lab can apply at any dropship online retailer and what they might want to do is
submit third get together testing for all of their products.
Some on-line retailers will run their very own exams paid for by the Lab,
and their own third party testing (independent lab check
results) is completed.
Anadrol (oxymetholone) is a strong DHT-derived
compound and arguably one of the best steroid for sheer mass achieve.
Anavar’s capacity to increase strength and energy can be attributed
to its distinctive degree of creatine uptake inside the muscle
cells (15), which will increase ATP (adenosine triphosphate) manufacturing.
Therefore, customers typically will only utilize mibolerone for a quick time
(i.e., a couple of days earlier than a particular
fighting event) and refrain from ingesting alcohol in the course of the cycle.
References:
also en ingles, Burton,
The aesthetic results you presumably can achieve
with Masteron are dramatic and spectacular, making this a favourite steroid within the
bodybuilding and physique competition. This natural response can result in hormonal imbalances, which may
subsequently result in decreased libido. Moreover, Trenbolone’s potential unwanted aspect effects like temper swings, nervousness, and depression would possibly additional contribute to a decline in sexual need.
Trenbolone is understood for its ability to burn fat and preserve muscle mass simultaneously.
It also has a big impression on rising muscle hardness and vascularity.
On the other hand, Testosterone is efficient in preserving muscle mass whereas weight-reduction plan,
however it is not particularly effective in burning fats.
The hormone concentration in Tren A reaches its peak per milligram while the drug
exits the physique at a fast rate. The drug offers wonderful choices for people beginning
their Trenbolone journey to handle potential unwanted facet
effects. The three steroids you talked about are all very fashionable decisions for these looking
to bulk up and achieve lean muscle mass. It is an artificial derivative of testosterone, and
it is used in veterinary medicine to extend the muscle mass and urge for food of cattle.
It’s essential to note that Trenbolone is harmful and may only be utilized by skilled bodybuilders.
It’s also crucial to suppose about legal and security elements, as using anabolic steroids like Trenbolone with no prescription is unlawful in many
nations. One of the primary benefits of Trenbolone Acetate is its ability to significantly enhance muscle mass and energy.
By stimulating protein synthesis and nitrogen retention, it promotes rapid
muscle progress and tissue restore.
Most labs additionally now carry ‘Tren-e’ but Tren-a nonetheless stays the dominant kind as it’s the best to regulate.
Below is a PCT protocol developed by Dr. Michael Scally, a hormone alternative skilled.
This trio of medications has confirmed to be a fantastic
success at our clinic and in clinical trials for restoring the
hypothalamic-pituitary-testicular axis (HPTA) from anabolic supplements steroids.
Nevertheless, we’ve had some sufferers report
approximately 80% of their hair loss reversing back post-cycle, after dihydrotestosterone ranges regulate back to normal.
Each of those alternatives presents their very own distinctive trenbolone stacking benefits, so it is necessary to do your research and consult with an expert before making a call.
Potential unwanted facet effects of D-Bal (Official
Website — Verified ✅) might embody bloating
and acne. Nevertheless, many bodybuilders have reported optimistic results with
D-Bal without experiencing any negative unwanted
effects. Now that you understand why some bodybuilders are
in search of Trenbolone options, let’s discuss the top
alternatives that can provide similar advantages without the risks.
The enhanced strength features from Tren use can have a profound influence on a person’s ability to push via intense exercises and carry heavier weights.
Enhancing efficiency capabilities, Tren has been recognized for its capacity to markedly boost bodily power positive aspects in users.
Moreover, Tren also enhances the production of pink blood cells, bettering
oxygen delivery to the muscular tissues during exercises,
which can enhance endurance and overall efficiency.
In any case, you will most likely turn to the tried and tested
PCT drugs in Nolvadex and Clomid, which both help stimulate testosterone
manufacturing. Mg/week is an effective and manageable dose for
many males (unless pimples is a giant drawback for you).
One of the most popular cycles is Masteron Enanthate, Testosterone Enanthate, and the powerful anabolic compound Trenbolone Enanthate.
Since all of them use the same ester, these compounds are very appropriate with one another and
produce superb outcomes for competitive bodybuilders, particularly in a pre-contest cycle.
Masteron helps prevent catabolism, so you maintain lean muscle
if you’re on a calorie deficit. Whereas some lean features are possible if you’re consuming at or
above upkeep energy, they are going to be average (but high quality) – doubtlessly
around 5 lbs if no other anabolic agents are used.
Masteron is an injectable steroid and some of the
highly regarded AAS used for slicing and getting shredded.
These measures assist mitigate potential side effects and ensure
a safer and more effective experience with trenbolone.
Moreover, proper diet and coaching protocols are important throughout a trenbolone cycle.
A balanced food regimen of protein, carbohydrates, and wholesome fats will assist
muscle growth and restoration.
TE and high TREN administrations elevated prostate mass by 84 (P
≤ 0.001) and 68% (P ≤ zero.01), respectively,
compared with SHAMs, whereas, neither low nor mod TREN elevated prostate mass above SHAMs.
In The End, the low TREN resulted in a prostate that was 34% smaller
than TE treatment (P ≤ 0.05). No differences in prostate mass
have been current between the high TREN and TE treatments.
ORX induced a nonsignificant 18% increase in retroperitoneal (retro) fat mass compared with SHAMs (Fig.
4A).
There are different alternatives to contemplate, of you aren’t open to
utilizing steroids for bodybuilding. Some of
those options are safer and may just be as effective as steroid use, however requires an amazing quantity t of
endurance and discipline earlier than important results can be achieved.
There is a excessive danger of steroid abuse
in person’s prone to dependancy or when bodybuilders self-medicate with a proper steroid cycle and PCT plan. When people start utilizing steroids, they typically improve their dosage for the first month, then gradually lower it as
they begin to feel the positive results. According to Endocrine Society
2010 guidelines, testosterone levels ought to be measured midway between injections
of testosterone enanthate or cypionate.
Right Here are 5 of one of the best authorized steroids which
have a stable reputation in the fitness industry. All supplements and brands
mentioned have a producers guarantee and are made beneath Good manufacturing
practices (GMP). There are a number of healthful meals that help
you naturally improve testosterone manufacturing and enhance muscle-protein synthesis.
Regardless of the burden coaching and preserving features after a steroid cycle, a lot of the side effects would be
the identical. The examine found that the testosterone
group that labored out experienced the highest strength
and muscle positive aspects, and the placebo group that didn’t work out acquired
little to no features. Analysis suggests that you would be gain more
lean muscle and strength from using steroids and not understanding than lifting naturally [6].
Anabolic steroids are designed to increase your testosterone ranges by giving your
body an external source of testosterone (known as exogenous testosterone).
Our in-depth and sensible guides cover every thing from food plan plans, weight
reduction, exercises, and bodybuilding to problems with psychological health.
At Horizon Clinics, we assist you to decode the solutions to your micro-health battles.
Useless to say, even if you’re supplementing, take in enough diet
and rely on abundant sources of protein and healthy fats.
There are only safe legal alternatives for muscle building you possibly can attempt,
corresponding to those mentioned above. However, nothing of such measure
can occur with the natural legal hormones for muscle development.
Some proof suggests sure individual components in workout dietary supplements can enhance muscle-building and bodily efficiency.
This article discusses what legal steroids effects on males [Mairie-sornay.fr] are and whether they work.
We also outline alternative choices for individuals wishing
to enhance their health. With common exercises and good
tracking, you’ll be able to see nice results.
Steroid use may cause pimples, swelling, temper swings, fatigue, and less starvation. Anabolic steroids,
that are like testosterone, trigger bloating and water retention. To maximize fat loss and weight reduction, customers should eat in a calorie deficit (-500
calories per day), which may even maximize muscle retention.
They additionally enhance purple blood cell manufacturing so my muscular tissues get more oxygen and nutrients during intense training.
Though the only real objective of anabolic steroids is
to promote weight gain, we’ve found that increases in muscle mass regularly coincide with concurrent fat loss (1).
The main cause people misuse anabolic steroids is to extend lean muscle mass when utilizing them in conjunction with weight training.
Some athletes, bodybuilders and others misuse
these medication in an attempt to enhance performance and/or improve their
bodily look. Anabolic steroids are the most common appearance- and
performance-enhancing drugs (APEDs). Approximately
3 to 4 million individuals within the Usa use anabolic steroids for nonmedical purposes.
Usually, Clenbuterol dosages will increase as your cycle progresses to
ensure your body doesn’t turn out to be used to the lower dose, which might
cause stagnant results. Anavar is not without side impact dangers – no
steroid is risk-free. At doses anywhere above the feminine beneficial vary of 5-10mg/day, virilization is undoubtedly a risk.
Some girls could be delicate sufficient at those low doses to
expertise unwanted effects, however so lengthy as you scale back the dose or cease utilizing Anavar, they need to go away alone.
That’s why legal steroids for muscle growth are often scheduled to
be taken around workouts. These dietary supplements solely multiply
the effectiveness of your workouts and diet.
We observe the proportion of muscle-building and
fat loss to be dissimilar to anabolic steroids, with HGH demonstrating enhanced lipolytic (fat-burning) results
in comparison with anabolic (muscle-building) results.
Chemically, the potent anabolic (muscle-building) and lipolytic (fat-burning) effects of anabolic steroids are as a
result of them each being exogenous types of testosterone.
Are you looking to achieve lean muscle mass, reduce body fats,
or improve endurance? Bodybuilders and powerlifters usually have a tendency to embody many steroids into their routine.
Negative libido impacts are possible; dosage is commonly set at half of the testosterone dose to fight this.
Equipoise has the added benefits of boosting the appetite
and enhancing stamina, vascularity, and pumps. EQ
can improve blood pressure, so dosing is usually
set at 50-75% of the testosterone dose to reduce back the
cardiovascular threat. Corticosteroids are one other well-known group, but
corticosteroids have completely different medical uses29.
It Is designed to help the body recover from the hormonal imbalances created through the use
of anabolic steroids. When artificial steroids are launched,
pure testosterone manufacturing is suppressed, which may result in varied
well being issues as soon as the steroid cycle ends. Anavar emerges as one of the mildest,
yet effective, oral steroids on the market. Suited for
each women and men, Anavar is especially known for its capability to shred physique fats and improve lean muscle with out
drastic weight increase.
The effects of Dianabol are additionally significantly appreciated by many athletes; nonetheless, it’s not as frequent because it once was
in athletic enhancement circles. Due to the potential fast increases in mass, many athletes will opt for Buy Oral Steroids Stacks like Anavar or Winstrol, nevertheless it usually depends on the aim of use.
Due to the fast and pronounced will increase in energy, which might translate
into extra energy and speed, this is normally
a solid athletic enhancer.
Fortunately, there are a number of completely
different types of testosterone alternative therapy
that may cater to your preferences and therapy wants. Buying testosterone on-line
or from a doctor’s office can be a huge step. But online testosterone
therapy is authorized and safer than ever, so lengthy as you’re working with
a reputable company. Steroid injections can be a key part of
a treatment plan for many autoimmune and joint conditions.
Steroids may be injected into joints, muscles, tendons,
the spine, or bursae.
If we are speaking about whole weight, you should embrace
water as a result of there’s no escaping the fluid retention that Dianabol
causes (but you’ll have the ability to certainly mitigate it as a lot as possible).
Within a quantity of weeks, gaining as a lot as 20 pounds of muscle mass is feasible.
During the first two weeks, I observed a slight increase in energy and a few pounds on the dimensions.
Now, for the third week on D-Bal, there are significant will increase in power throughout the board and more mass
gain. I really have two weeks left on it, and I have no idea how much more I can acquire.
This is where a post-cycle therapy plan is important, with SERM medication like Nolvadex and Clomid being essential to have available, able to go.
These drugs accelerate your testosterone recovery and effectivity whereas levels are beginning to get again to regular naturally.
As A Result Of of their past unlawful use, anabolic steroids—which are testosterone derivatives—are now thought of
controlled substances. In 1991, testosterone, along with numerous anabolic steroids, acquired a restricted drug designation. Common and delayed-release (depot) dose variations of testosterone are each injected intravenously.
Injectable steroids offer many benefits to bodybuilders and athletes
alike. They can present increased muscle mass, improved energy and performance,
and reduced body fats. In addition, injectable steroids can improve skin quality,
enhance athletic coaching results, and cut back the chance of situations such as osteoporosis.
Overall, cortisone pictures can provide vital pain reduction and improved joint perform, permitting you to regain mobility and interact in rehabilitation exercises.
With careful planning and understanding of your insurance coverage protection, you can confidently pursue cortisone pictures as a viable remedy choice in your joint well being wants.
Those with autoimmune ailments or who take immunity-suppressing drugs or are prone to an infection ought to inform
their health care provider. Corticosteroid pictures do assist many patients, however
they have limitations. Relying on your situation, your physician might
restrict the frequency of your photographs to at least one
each six weeks and not more than 4 in a 12 months.
The Pain Relief Center in Dallas is right here to help those who wish to
know, “Where can I get a cortisone shot with out insurance? Additionally, read our blog about Carpal Tunnel Remedy to be taught extra about your pain management options. Steroid photographs deal with irritation just about anyplace within the physique, corresponding to a particular joint or tendon. In addition, shots treat widespread inflammation in the body for asthma, allergic reactions, and rheumatoid arthritis.
This makes Dianabol a versatile steroid that may fit proper into any cycle, whether you’re teaming it up with only one other steroid or multiples in a extra superior stack. At 20mg day by day or more on a Dbol-only cycle, you can introduce an AI, and if you dose it correctly, you must have the ability to control suppression-related unwanted effects. Arimidex, dosed at 0.125mg to 0.25mg and taken twice weekly, is commonly efficiently used in this Dbol-only cycle strategy. Dianabol is among the solely AAS men who can run as a sole compound and not utilizing a testosterone base if you do things correctly. Dianabol IS suppressive, but at a average dose and quick cycles, it’s possible to run Dbol alone and not undergo from low testosterone.
You not often hear someone speaking about Dianabol without discussing some unwanted effects. Lucky guys may have only mild unwanted side effects, however many will have more severe ones, and this is expected when using a potent compound like Dianabol. This is a steroid that has been used for many years, so tons of of thousands of customers have shared their outcomes and experiences a method or another. That said, Dianabol can help make shedding fats easier whereas utilizing it, even if it’s not your main objective. Because Dbol is extremely anabolic, it will enhance your metabolism somehow. It may also help retain muscle by preventing catabolic processes (where muscle is lost as energy).
Some people also have modifications within the color of the pores and skin at the web site of the injection. It’s also a good suggestion to let a health care provider know when you haven’t noticed any improvements in order that they’ll modify or change your treatment. Cortisone photographs typically cost roughly $100 to 300 however may be greater than $1,000. Cortisone can journey into your bloodstream and trigger full-body unwanted effects.
Some unwanted side effects of dexamethasone could occur
that often don’t want medical attention. These unwanted
facet effects may go away during treatment as your physique adjusts to the
drugs. Also, your health care skilled may
find a way to inform you about methods to forestall or reduce a few
of these side effects. One of the most typical unwanted effects of
Deca Durabolin is irregular menstrual cycles in ladies.
One possible side effect of Deca Durabolin is elevated facial and physique hair progress.
For example, some athletes choose to cycle buy cheap steroids online in accordance with their training schedule,
taking them in periods of intense training and then resting during
periods of restoration. As you can see, this steroid can be extraordinarily useful
for these trying to improve their total well
being and health levels.
In conclusion, Deca Durabolin’s role in bodybuilding is a testament to its highly effective anabolic
effects and the acute lengths to which some athletes will go
in pursuit of physical perfection. While its use has undoubtedly contributed to some exceptional physical
transformations, it also serves as a focus for ongoing debates about health,
ethics, and the future of bodybuilding as a sport.
As research continues and attitudes evolve, the place
of Deca Durabolin in bodybuilding could change, but its impression on the game’s historical
past and tradition is simple. The lowered aromatization of Deca Durabolin means that users could experience fewer estrogen-related unwanted effects, similar to water retention and gynecomastia,
compared to other steroids.
Some examples of live vaccines embrace measles, mumps, influenza (nasal flu vaccine), poliovirus (oral form),
rotavirus, and rubella. Do not get near them and don’t stay
in the identical room with them for very long. Using this
medicine might improve your threat of cancer, together with Kaposi’s sarcoma.
Dr. O’Connor has over 20 years of experience treating men and women with a history
of anabolic steroid, SARM, and PED use. He has
been a board-certified MD since 2005 and offers guidance on hurt reduction methodologies.
We have also seen Proviron reduce the estrogenic unwanted aspect effects of Dianabol because of
its working as a systemic anti-estrogenic agent (41).
Thus, gynecomastia and water retention (bloating) are less prone
to happen with the addition of Proviron. Deca Durabolin can also be considerably less androgenic in comparison with Dianabol, which means ladies are much less more probably to expertise
virilization side effects on Deca in low doses
in comparison with Dianabol. Trenbolone is significantly extra androgenic than Dianabol;
thus, oily skin, acne, and hair loss are more widespread with
trenbolone.
If you ask me one of the only ways to avoid Deca Durabolin’s unwanted facet effects,
I Will advise you to cease utilizing it completely and go the
natural route (more on this soon). However, knowing what to search for can help minimize the chance of problems.
If you’re taking Deca Durabolin and are beginning to lose your
hair, you will want to communicate to your doctor.
So while NPP might require far more pins than its nandrolone
cousin, you will not have to make use of it as long both.
One Other advantage that nandrolone phenylpropionate has
over deca is that it would not keep in your system as
lengthy. Given that it is quicker acting, NPP metabolites clear your physique inside months.
However, we now have seen such protocols lower power positive aspects due to the elimination of intracellular fluid (inside the muscle cell).
Moreover, we discover drugs that treat excessive estrogen levels can have
a negative effect on blood lipids (with the
exception of Nolvadex). Due to Dianabol’s aromatization effects and being
highly estrogenic, it’s a compound that causes important amounts of water retention. This can leave
users wanting puffy, bloated, and smooth-looking; therefore, why it’s sometimes used in the off-season. Secondly,
Dianabol can increase blood pressure as a end
result of an increase in water retention. As your physique retains more fluid
and your weight will increase, so does your blood strain (9).
Nonetheless, because of Dianabol inflicting some extracellular
fluid retention (water collecting outside the muscle cell),
we do not price it as the best steroid for enhancing vascularity.
The results of Deca Steroid on muscle growth and physical performance don’t happen in a single day.
Due to its lengthy ester, users would possibly start noticing bodily adjustments and strength
enhancements within 4 to 6 weeks of constant use.
However, the total advantages, particularly in muscle mass, are sometimes
noticed after eight to 12 weeks of use. Tren’s origins trace again to the Sixties when it was first created to
enhance feed effectivity in cattle — basically helping them acquire extra muscle from the food they
eat. Its transition into the realm of bodybuilding began when athletes acknowledged its potent anabolic results and started doping with veterinary-grade Trenbolone.
Though by no means accredited for human use by the FDA,
Tren has turn out to be a staple for competitive athletes looking for rapid power positive aspects and muscle
mass. As the first male sex hormone, testosterone
plays a central role in creating muscle mass, libido,
mood regulation, and secondary sexual characteristics.
From a safety perspective, we discover Winsol to be the higher possibility, with not certainly one of the above unwanted
side effects being a trigger for concern. Deca Durabolin is a popular injectable bulking steroid, usually used within the off-season. To do that, we entered ‘#crazybulk’ and #dbal, along with different product names, into Instagram, Twitter, and Fb.
We do not know what type of impacts on long-term health
MK-677 could have without long-term human research
yet existing. MK-677 does not trigger any androgenic side effects or any
unwanted effects associated to estrogen or DHT. That means adverse results like pimples
and hair loss shouldn’t be a difficulty with
this compound. Despite MK-677 not inflicting changes to estrogen, the development of water retention continues to be a risk,
and this happens for causes that I’ve outlined further beneath.
Many folks can use this compound without any fluid retention, and
it’s typically dosage-dependent.
Many turn to an injectable steroid for consistent, longer-lasting results that help them
keep in peak situation. D-Bal works by increasing nitrogen retention inside your muscle tissues,
a key factor for muscle protein synthesis. If you’re searching for the best
legal steroid different to Dianabol without the risks of unlawful
anabolic use, D-Balfrom CrazyBulk stands out as a best choice.
Designed to imitate the muscle-building power of
Dianabol, D-Bal offers massive positive aspects in muscle mass, power, and
performance — all without dangerous unwanted facet effects
or prescriptions.
The nickname “A-Bombs” for Anadrol is fitting, as its physiological effects resemble dropping
a bomb on the liver. We have seen extensive cycles and high doses cause extreme
quantities of hepatic harm and, in some circumstances, cause cirrhosis.
When Anadrol is taken in today’s dosages of 50–100 mg/day, we’ve discovered it to be one of many worst steroids for cholesterol and blood strain. There’s no higher hormonal setting for anyone looking
for peak efficiency in sports or in bodybuilding. Do you understand
what separates an everyday athlete or bodybuilder from a professional one?
The professionals typically run as a lot as totally different compounds at one time to unlock their
best physique and performance. The most tough a half of any chopping cycle is to get your
body to shed that stored body fat quick.
You will need to maintain any water retention in verify to ensure your positive aspects are high quality lean muscle.
Anabolic steroids on the market are essentially the most potent form
of gaining muscles rapidly. It features by enhancing the levels of testosterone within the body, which then will increase the speed and strength of rising muscular tissues.
If you want to pack on some critical measurement quick, that is your go-to tool.
Stacking steroids require a deep understanding of
how every steroid works, in addition to how they work synergistically.
However, masculinization is frequent if a feminine will increase the Winstrol dose or extends this cycle
beyond six weeks. Comparable to Anavar, Winstrol does not aromatize;
consequently, striations and muscle definition will become more prominent, as properly as vascularity.
This is a consequence of the depletion of extracellular water retention,
which is the fluid that accumulates between the dermis and
muscle.
Nolvadex and clomid are freely obtainable from pharmacies and may be purchased with
no prescription. In the preparation in your steroid cycles you have to take note
of the toxic properties of drugs. For example methandrostenolone has a toxic
effect of steroids on body; chirurgiemain.fr, on the
liver, from which it follows that it must be utilized only
in average or low doses.
The most typical use of Nandrolone is in bulking cycles the place it’s stacked with testosterone and different steroids; Dianabol
is another very generally used AAS with Deca or NPP.
One of the downsides of Deca’s long half-life is that should you reply
negatively to it, you’ll be waiting lengthy for opposed
effects to fade away. So, if it’s your first time utilizing Nandrolone,
it can be wise to begin with NPP as a substitute.
If things go downhill early on, you’ll find a way to cease and have that NPP leave your system inside days quite than weeks.
The use of anabolic steroids is extremely regulated in most
nations, together with the Usa. These substances are categorised
as Schedule III controlled substances underneath the Anabolic Steroid Control Act, and
their possession or distribution and not using a prescription is illegal.
Bloodwork 4–6 weeks post-PCT is crucial to
make sure your natural testosterone and general hormone profile have returned to baseline.
If not, additional restoration time — or physician-guided treatment — may be essential.
Some examples of those products are HGH-X2, TREN-MAX, D-Bal, and Anvarol.
Individuals who use CrazyBulk usually have a tendency to
acquire the ideal levels of muscular growth and body definition. In essence, unintentional doping can be averted and each effort have to be
made to prevent unintended doping instances. Examine on awareness of unintentional doping
among athletes showed that solely forty.6% refused
to eat an unfamiliar food that was given to them,
and only sixteen.1% learn the ingredients listing before consumption [136].
The best prevention is healthier analytical management, education, and data.
WADA established ADEL (Anti-Doping Training and Studying platform), a web-based platform that helps in education and supports the antidoping community.
Athletes and their groups need to obtain details about dietary
supplements and to make certain that labeled substances aren’t on the WADA
prohibited record [93].
These taking the supplement showed a big enchancment of their
lower physique muscular endurance. This article discusses what authorized steroids
are and whether or not they work. We also define different options for individuals wishing to improve their health.
The finest legal steroids are made with pure ingredients
like natural extracts and vitamins, and there are heaps of on this list to choose from.
Aspirin thins if and dissolves clots, so you you think, that it will still be good to make use of
on or off gear. Possibly somebody can piggy back off this question for a more precise answer.
Turkesterone and ecdysterone are among the many best supplements for these wanting to stay pure.
Anabolic steroids aren’t usually secure for human consumption exterior
the confines of drugs.
Low-density lipoprotein (LDL)—the bad—cholesterol
ranges increase, and high-density lipoprotein (HDL)—the good—cholesterol ranges lower.
Extreme cardiovascular issues, including hypertension,coronary heart assault, and blood clots,
are reported with the usage of anabolic steroids. Maintain in thoughts that the Meals and Drug Administration (FDA) regulates dietary dietary supplements
with a unique set of necessities than pharmaceutical medicine.
Manufacturers are liable for their own evaluation of the protection and labeling of their merchandise earlier
than advertising, which explains why prohormone supplements are technically
authorized regardless of the health risks. In addition to many natural steroids having security concerns, products
that corporations promote as dietary supplements usually are
not regulated in the same way as drugs.
After every week, I observed that my bench press was up 10%, and
my endurance had considerably increased. First of all, I was surprised by the instant boost
in exercise efficiency it gave me. I also had extra energy throughout
the day, which made it simpler to stay motivated and keep up with my intense lifting classes.
Glasgow, Could 03, (GLOBE NEWSWIRE) — Reaching
serious muscle development calls for more than simply time in the health club.
Whereas constant coaching and a strong diet plan kind the muse,
dietary supplements can offer an added edge. For athletes and
bodybuilders, the proper merchandise assist restoration,
improve strength, and help break by way of plateaus.
Together, they assist enhance protein synthesis, enhance nitrogen retention, increase testosterone ranges, and improve purple blood cell production—all key elements for sooner muscular development and superior workout efficiency.
These dietary dietary supplements are designed to simulate the effects of Anabolic Steroids Description (Dfestival.Festivaldemalaga.Com)
steroids corresponding to Anadrol, Dianabol, and Winstrol, amongst others
like them. According to the corporate’s website, CrazyBulk exclusively makes use of natural chemical substances
that have no unfavorable unwanted side effects. CrazyBulk is designed
to assist people in accomplishing their goals by giving them
a head start on the journey towards success.
In medical research, two males skilled SARM-induced hepatocellular-cholestatic injuries (1).
This was the consequence of taking LGD-4033 for 9 weeks and RAD-140 for
simply four weeks. When buying turkesterone dietary
supplements (or ajuga turkestanica extract), be positive
to examine the yield amount of the energetic
ingredient. Instead, it naturally happens in thistle-like
plants, most of which grow in Central Asia, similar to places like Siberia, Asia, Bulgaria and Kazakhstan. Crops that it’s commonly extracted from include those
referred to as leuzea or maral root and Ajuga turkestanica.
The numbers of implants, viable fetuses, and viable male fetuses have
been slightly decreased (not statistically significant) in the 30.0 mg/kg androstenedione group.
Whether such a hormonal enhance may also be observed in fetuses must be monitored for conclusive effects.
Finally, androstenedione publicity appears to have an result on implantation adversely;
nonetheless, further studies must be carried out with larger
numbers of animals or ideally in humans. Proteins, vitamins,
and creatine are the most important non-hormonal meals
dietary supplements [16]. Protein dietary supplements when combined with resistance coaching proved useful in enhancing fats fee mass and
strength in healthy people [17].
However getting older can cause ranges to drop, resulting in problems like weight acquire.
Elevated ATP manufacturing and lowered serotonin will allow you to smash plateaus and break private data easily.
Last but not least, protein synthesis mainly equals stronger muscle tissue, and D Bal Max delivers fairly well on this end.
The means of tearing down and rebuilding bigger, extra outlined muscle is enhanced, and you’ll begin seeing satisfactory outcomes every time you take the complement.
Adding high-quality protein from lean meats, dairy, and legumes gives the proper amino
acids for muscle repair and buildup.
These should prove that the saying ‘needles as soon as, features forever’
is nothing but an invite for a lifetime of trouble. It is recommended
to take Anavar dosage about minutes before figuring out.
This will allow enough time for the steroid to take effect and give you an additional enhance of power throughout your lifts.
Anavar has a half-life of roughly 9 hours, which means it stays in your system for
about hours. Nevertheless, it could be detected in drug exams for up
to 3-4 weeks after the final dose. PEG-MGF (Pegylated
Mechano Development Factor) – Enhances muscle restore and progress post-exercise.
Click On here to discover the mind-blowing before and after transformations of Trenbolone customers.
Remember, the protected utilization of Trenbolone requires accountable decision-making, adherence to
pointers, and ongoing communication with healthcare professionals.
Testosterone and Deca Durabolin complement Dianabol well, as they don’t pose as a lot hepatotoxicity as oral steroids.
We have found that an extra 10 lbs of mass could be gained when adding testosterone or Deca Durabolin to a Dianabol cycle.
For somebody who has by no means taken Dianabol earlier than, it might be sensible to run a Dianabol-only cycle
earlier than stacking it with other steroids so the body
can turn into accustomed to Dianabol first.
However, users with compromised liver operate or those planning on drinking alcohol or taking hepatotoxic medications at the aspect of
Winstrol are on the highest threat of liver damage.
Winstrol is a C-17 alpha-alkylated steroid and thus passes by way of
the liver before entering the bloodstream. As the workload of the liver increases, we see alanine aminotransferase (ALT) and aspartate aminotransferase
(AST) enzymes rise, indicating stress and harm to the organ.
Low-density lipoprotein (LDL) ldl cholesterol considerably rises, and high-density lipoprotein (HDL)
ldl cholesterol drops. This happens as a result of Winstrol failing to aromatize, in addition to stimulating hepatic lipase within the liver.
Hepatic lipase is an enzyme that has a detrimental impact
on blood lipids.
Legal steroid alternatives provide a safer, effective approach to achieve related
results. By understanding the benefits and risks of every possibility, you can also
make knowledgeable choices to support your bodybuilding
journey. Natural dietary supplements, a balanced food plan,
and a constant workout routine are the foundations of sustainable muscle development
and overall well being. This steroid is especially in style for its capacity
to assist customers achieve speedy weight gain, with some users reporting features of up to
25 lbs of muscle mass in as little as 4-6 weeks.
It is necessary to remember that these weight and power gains could come with different results,
similar to water retention and the chance of unwanted effects.
Anavar is famend for its constructive influence on power and endurance.
It stimulates the production of red blood cells,
improving oxygenation and nutrient delivery to the muscular tissues.
It heightens the body’s metabolic price, thus resulting
in the next rate of fat burning. So, beyond muscle constructing, it
aids in shedding excess fats – an important step in attaining outlined muscles.
If users utilize RAD one hundred forty for short-term use, such hair loss could reverse when hormones regulate again to normal post-cycle.
However, long-term use and sustained high ranges
of DHT improve the risk of androgenetic alopecia.
Anavar is usually thought-about to be one of many least potent steroids out there.
This may sound like a nasty thing due to course, it implies that the positive aspects are
additionally lesser.
The combination of fats loss and lean muscle
preservation makes it a well-liked choice amongst ladies pursuing their bodybuilding
and fitness targets. One of the primary advantages of Anavar is its ability to promote lean muscle progress.
By enhancing nitrogen retention and rising protein synthesis
inside the muscular tissues, it creates an setting conducive to muscle
growth.
The first few weeks I went loopy, it felt as if I may drill holes with my boner.
Good factor there’s apps nowadays and it’s simple
to get some relieve. This went nearer to normal over time, most likely additionally as
a end result of I killed my E2. For most of the cycle I stayed below my
TDEE, averaged at ~2500 kcal, with 280g protein,
180g carbs, low fats. Nevertheless, trenbolone just isn’t really
helpful for beginners due to its detrimental results on the HPTA (hypothalamic-pituitary-testicular axis) and its
increased danger of arteriosclerosis (4). Moreover, a small calorie deficit will maximize
fat burning when chopping on trenbolone. Nonetheless, we contemplate Winstrol’s unwanted effects to be harsh,
particularly on the center and liver.
Keep In Mind, anabolic steroids like this one are highly effective substances with vital implications.
Being accountable means arming your self with the best knowledge, acting underneath skilled steerage, and respecting your body’s limitations.
Those keen on exploring its benefits should achieve this with care and warning.
Just as we take care of ourselves through the steroid cycle, aftercare is equally pivotal.
This includes normalizing your hormone ranges and helping your body recuperate from
a cycle. Submit Cycle Remedy (PCT) is typically used to revive natural hormones, especially testosterone.
This helps in stopping undesirable effects post a steroid
cycle and ensuring a seamless transition after you’ve completed a cycle.
As you proceed to use Anavar, you’ll find that your results will continue to improve until you
reach the four-week mark. At this level, you’ll be able to anticipate to witness probably the
most exceptional adjustments in your physique, together with visible enhancements in muscle measurement and
definition. By the tip of the first month, you have to be at your peak
results, with a extremely defined and shredded physique and a big
improve in energy. Sermorelin – Encourages natural GH manufacturing,
supporting recovery and lean muscle positive aspects.
Enhanced Muscle Improvement – By blocking myostatin, ACE-031 eliminates the organic restrict
on muscle expansion, permitting for vital increases in lean muscle
mass.
Bodybuilders at our clinic who hyper-respond to trenbolone typically acquire extra
muscle and burn extra fats than those that are hypo-responders.
Observe how to buy steroid injections; drei90.de, the deltoids
and trapezius muscles are more outstanding within the after picture, a characteristic of trenbolone’s excessive androgenicity.
These two muscle teams have a high variety of androgen receptors, thus making
them more vulnerable to progress.
Anabolic steroids are artificial variations of the naturally occurring male
intercourse hormone, testosterone. They are more properly known as anabolic-androgenic steroids (AASs), because they have both bodybuilding (anabolic) results and
masculinizing (androgenic) results. The masculinizing effects of testosterone trigger male
characteristics to appear during puberty in boys, such as enlargement of the penis, hair growth on the face
and pubic space, muscular improvement, and deepened voice.
Females additionally produce natural testosterone, but ordinarily in much
smaller amounts than males. Epi 2.zero noticed a surge in growth this year amongst natural athletes and women looking to tone
up permitting it to climb up a spot in the Top 10.
It takes an progressive strategy to muscle development which is
Myostatin Inhibition.
Plus, our aggressive costs, coupled with regular reductions and promotions, make it straightforward to get the most effective value in your cash.
Correct cycle management and post-cycle therapy are important to reduce back these dangers.
There’s nothing wrong with enthusiasm, however diving in with zero data
or idea of what might go wrong, let alone HOW you
must be utilizing steroids, is going to show your steroid expertise into a residing nightmare.
The half-life of a steroid is a very totally different concept to detection time.
While the half-life does affect detection time to an extent, you cannot depend
on a steroid’s half-life to estimate just how long that steroid
may be able to be detected via drug testing. Some steroids will be more fat-soluble than others and
so detectable for longer periods due to them remaining
in the fat tissue. Anabolic compounds are very fat-soluble
compounds, which means these are substances which would possibly be absorbed with fats after which stored in fatty tissue.
Primobolan is mostly not thought of the most effective sole compound for this length cycle since you would typically need
to use it for greater than eight weeks. Steroids play a role in optimizing the nutrient pathways of the physique by successfully shuttling nutrients to muscle tissue that are giving the sign that protein synthesis
is being initiated21. So all those quality carbohydrates, wholesome fat,
protein, nutritional vitamins, and minerals are making their approach to the muscle tissue quicker and extra significantly than if you have been not using steroids.
It Is like adding rocket gas to your muscle-building efforts,
enhancing strength, libido, and general performance.
Feeding your physique with sufficient vitamins and minerals like magnesium for much less muscle injury, proteins, and good fat is vital for lasting muscle development.
Hormone substitute therapy is a possible technique for the safety of bones from postmenopausal osteoporosis [76].
Nevertheless, there are multiple disadvantages because of their
potential harmful unwanted aspect effects in other organs [77].
It is unclear whether or not androstenedione may influence the degrees of physiological hormones
by altering the liver enzyme activities that metabolize
steroid hormones or not.
In Accordance to Lyle, females can build muscle at about half
the rate outlined within the formulation. Sure, these surveys have been nameless, so perhaps all members had been trustworthy.
While these are stackers pills High
figures, the actual variety of steroid customers is likely even higher.
Steroids also can have (permanent) long-term side effects, such as liver disease, male-pattern baldness, heart dysfunction, and gynecomastia (breast development).
Incorporating these supplements responsibly entails diligent analysis and a
proactive approach to potential well being impacts.
Steroids are synthetic or natural natural compounds with a molecular
construction of 17 carbon atoms arranged in four rings.
They include intercourse hormones, adrenal cortical hormones, bile acids, and sterols.
Clenbuterol isn’t an anabolic steroid however is commonly used for fats loss due to its powerful thermogenic properties.
Equipoise increases purple blood cell production and offers steady muscle gains with fewer unwanted effects.
D-Bal is a natural supplement designed to copy the muscle-building effects of Dianabol without the harmful unwanted side effects.
Furthermore, there’s a lack of scientific proof to help many of
the makes use of of Tribulus. Subsequently, it is at all times
really helpful to consult with a healthcare professional before beginning any
new complement routine. Supplements are designed to boost your food regimen and training, not exchange them.
Whether Or Not or not they give you the outcomes you want
will depend if you’re training exhausting and consuming right.
Both Sapogenix and Ecdysterone are potent pure anabolics that may help optimize
muscle progress and power. If you’re bulking
it is a great compound to make use of, but it’s also useful
for cutting since it could increase power and
muscle protein synthesis. Equally to Sapogenix, Ecdysterone comes from a family of plant steroids that has been shown to help with muscle
positive aspects, protein synthesis and extra.
A current research printed within the Molecules journal has
highlighted the significant role of androstenedione, a naturally occurring
steroid hormone, in the manufacturing of estrogen and testosterone [16].
Youngsters with weight problems endure from larger levels of adrenal androgen than their normal counterparts,
which might lead to accelerated pre-pubertal development in these youngsters [73].
Kwon [74] and his colleagues examined the association between bone
age (BA) and chronological age (CA) ratio (BA/CA) and androstenedione and
testosterone levels.
You can add this grain to your meats dishes thrice per week
to reap its benefits. Doctors can only prescribe the best steroids
for patients with serious situations. Medical Doctors do not prescribe even just a little dose
of any common or the most effective steroid selection to wholesome or young folks simply to enhance their efficiency in athletics.
For example, a authorized steroid may be prescribed to hasten delayed puberty or revive testicular capabilities.
It’s also a DHT-derived oral steroid, however it’s thought of one of many
more milder compounds. If you’re going to do recurring cycles, your
off-cycle time must be at least equal to the size of the cycle itself.
This permits a full HPTA restoration (aided by PCT) and a sufficient break from all steroids
before getting back to another cycle.
CrazyBulk offers complete stacking choices for different fitness targets.
Whether you’re looking to bulk up, reduce fat, or achieve power, CrazyBulk has pre-designed stacks that combine the simplest supplements that will help you achieve your goals sooner.
CrazyBulk’s TREN-MAX (Trenbolone Alternative) is designed to
reinforce blood circulate and increase oxygen delivery to the muscles.
This leads to better endurance, sooner restoration, and improved muscle fullness.
Every one of them was developed in accordance with stringent parameters.
Legal steroids, along with healthy meals and regular exercises,
may help build your physique safely, avoiding the harm from illegal steroids.
Some elements, for example, DMAA, can push up your blood pressure, and the FDA says they’re no good.
Proteins and amino acids are important for constructing and repairing muscles, making
them must-haves for muscle growth. According to Sports Activities Med,
competitive bodybuilders focus on protein to keep muscle and assist with restoration. These vitamins are the constructing blocks that assist muscle development
and forestall muscle loss. Historically, safed musli has been used to boost efficiency, by
enhancing stamina and muscle strength.
As A End Result Of the “come down” on Anadrol is severe, we
typically see steroid customers go for a milder safe Steroid cycle
(repueblo.es) similar to Deca Durabolin after coming off to help this transition. Such
a protocol may assist customers retain extra strength and muscle positive aspects skilled from an Anadrol cycle.
A diuretic may be used to lower blood stress if a person experiences significant water retention. Nevertheless, this can end in much
less muscle fullness and decreased strength features due to less ATP manufacturing contained in the
muscle cell. To reduce liver danger, it’s important to use steroids on the lowest efficient dose for the shortest length necessary.
Additionally, common monitoring of liver operate and avoiding alcohol consumption may help.
Although it’s going to bypass the liver upon entry into the bloodstream,
it will have to course of through the liver upon exiting your physique.
Possession of Anadrol (or other steroids) can result in a 1-year prison sentence and a minimal fantastic of
$1,000. There are two explanations for why Anadrol doesn’t
trigger virilization in ladies. Firstly, we find that masculinization happens in girls when androgen ranges are high
and estrogen ranges are low.
Some folks consider Anadrol causes fats gain; however, this isn’t
accurate. All anabolic steroids, including Anadrol, are various sorts
of exogenous testosterone. Thus, totally different steroids will build muscle and burn fat to totally different degrees.
Nonetheless, we discover the lean muscle gains on Anadrol are nonetheless important, due to increased testosterone levels, protein synthesis, and nitrogen retention.
D-Bal accommodates components which may be recognized to
boost free testosterones in the blood. The formulation additionally guarantee that you’ve
enough protein to which that free testosterone can then connect.
D-Bal not only helps you put on lean muscle sooner however greatly will increase
muscle restoration and reduces soreness.
CrazyBulk’s loyalty program permits you to earn factors with each buy.
These points may be redeemed for reductions, free products, and unique merchandise-adding further worth to your
investment in the most effective authorized steroids in 2025.
What’s remarkable about CrazyBulk is not just the merchandise themselves but the group it has
created.
Long-lasting unwanted facet effects of prohormone use can embrace cardiovascular risks, irreparable liver
and kidney injury, and elevated cholesterol levels.
These supplements use natural things to give you comparable good
muscle results however with out nasty well being issues.
A research in 2018 even discovered that protein shakes and lifting weights may make muscles larger and stronger.
We should promote strategies for building muscle tissue which might be protected and healthy.
A detailed research in 2018 confirmed mixing protein supplements with resistance exercise can grow
muscles more. It Is crucial to keep your coaching various to keep away from hitting
a standstill and to keep enhancing. Resistance training is key for constructing muscular tissues and
boosting endurance. Regular coaching, especially with creatine, significantly will increase muscle size.
Long-term oral steroids often trigger skin issues corresponding
to slower therapeutic after accidents, thinning skin, and straightforward bruising.
Typically the steroid treatment is steadily stopped if the condition improves, and
restarted if it worsens. The kind mentioned in this leaflet is the pill form, taken by mouth, called oral steroids.
Corticosteroids differ in their relative amount of anti-inflammatory and mineralocorticoid potency and they’re used based on these results.
Corticosteroids, since they suppress the immune system, can result in an increase
in the price of infections and scale back the effectiveness of vaccines and antibiotics.
It doesn’t embody corticosteroids used within the eyes,
ears, or nostril, on the skin or which may be inhaled, although small amounts of these
corticosteroids can be absorbed into the
physique. Some sufferers who took prednisone or comparable
medicines developed a sort of most cancers referred to
as Kaposi’s sarcoma. Round 5 out of each one
hundred individuals (around 5%) experience severe mental well being problems after
they take steroids. Let your healthcare staff know should you discover any
changes in your emotional or psychological wellbeing.
Additionally let them know if you or any family members have ever had despair or manic despair (bipolar disorder).
They assist to manage many features including the immune system,
reducing inflammation and blood pressure.
Talk along with your doctor about whether prednisone is best for you.
Prednisone can sometimes cause harmful results in individuals who
have sure situations. Different components can also have an effect on whether or not prednisone is
an effective treatment choice for you. Before taking prednisone,
remember to tell your doctor about all drugs you’re taking (including prescription and over-the-counter types).
Your physician or pharmacist can tell you about any interactions this stuff might trigger with prednisone.
No, you’re unlikely to be prescribed prednisone
for a sinus an infection or cough.
A doctor can legally prescribe them in case your physique would
not make enough testosterone. Docs also prescribe them to males with low
testosterone and people who lose muscle mass due to
most cancers, AIDS, and different health conditions.
There is also a model that can be inhaled by way of your
nose.
Prednisone comes as an immediate-release tablet that you simply swallow.
(An immediate-release drug is launched into your physique right away.) Prednisone additionally
is out there in different forms taken by mouth, but these aren’t coated on this article.
Healthcare Insights are developed with healthcare industrial intelligence from the Definitive Healthcare
platform. Begin a free trial now and get entry to the most recent healthcare business intelligence on hospitals, physicians, and different healthcare providers.
In particular, individuals who have not had chickenpox
or measles in the past (and so usually are not immune) may be
advised to avoid individuals with chickenpox, shingles or measles.
Normally steroids are taken very first thing in the morning,
with food. Some glucocorticoids also along with their
anti-inflammatory actions have salt retaining properties but
they’re used largely for their anti-inflammatory effects.
Whether Or Not you’re a newbie looking to make your first
buy steroid Uk or an skilled lifter exploring new choices, this guide covers the
necessities. Let’s break down the top choices out there today and the way they can support your goals in a secure,
strategic means. Patients with respiratory syncytial virus (RSV) hospitalization have elevated odds
of any acute cardiovascular event compared with COVID-19 hospitalizations,…
Male athletes with high-volume train training have a better burden of calcified plaque than male
nonathletes, based on a review printed within the…
Prednisone may suppress growth and improvement, an unlucky effect that may be helped by alternate-day treatment or development hormone remedy.
Prednisone may also trigger sleeplessness and affect your moods.
Folks with diabetes may discover their blood glucose management is inferior
to it normally is whereas they’re taking prednisone.
Prednisone has long-lasting effects and is often prescribed once
day by day.
Anabolic steroids are manufactured drugs that carefully resemble the hormone testosterone
or other androgens. Aggressiveness and urge for
food might improve, particularly with excessive doses.
In younger adolescents, steroids can interfere with the development of arm and leg bones.
Prednisone makes you hungry and weight gain is a standard side effect.
Fat deposits may happen around your stomach, face, or back of your neck.
Fluid retention can also happen and may manifest as leg swelling and a sudden weight enhance.
If you’re on daily prednisone, experts recommend taking the dose in the morning, to scale back this risk.
Some researchers assume that inhaled corticosteroid medicine may slow progress charges in kids who use them for bronchial asthma.
Amongst all glucocorticoids, prednisone is not efficient in the body until it’s converted
to prednisolone by enzymes within the liver. For this cause
prednisone may not be very effective in people with liver illness due
to a reduction of their ability to convert prednisone to prednisolone.
Prednisone may increase your threat of harmful effects from a stay vaccine.
Live vaccines embrace measles, mumps, rubella (MMR), rotavirus, yellow fever, varicella
(chickenpox), one sort of the typhoid vaccine and nasal flu (influenza) vaccine.
This can help negate a few of the opposed results of Anadrol (including strain on the
heart). Nonetheless, we all know of bodybuilders and
men’s physique rivals that may cycle Anadrol simply earlier than a present to increase muscle size and fullness.
This may be done with out important increases in water retention if an individual is
lean and retains their diet clean. However, it’s not generally taken throughout a chopping cycle as a outcome
of extracellular water retention. This has the potential to decrease muscle definition and
enhance bloating, which will not be aesthetically pleasing
when trying to burn fat.
Prednisone belongs to a bunch of medicines
referred to as corticosteroids. It has anti-inflammatory effects and might regulate
the body’s metabolism and immune response. Normally
buying steroids online safe (Steffen)
can safely be used safely in pregnant or breastfeeding women. The lowest
dose attainable for the shortest potential period of time
can be used. Injected corticosteroids may cause momentary side effects near the location of the shot.
Synthetic steroids are illegal substances that you simply get on the black market.
You administer them yourself and hold track of your progress and any potential unwanted
aspect effects your self. Typically confused with steroids, testosterone boosters
don’t really comprise steroids or testosterone. They comprise elements which are capable of doing
two issues. They set off the production of testosterone indirectly
and/or they prevent the conversion of testosterone into different hormones, which is type
of a typical prevalence. This condition results
in male breasts creating and, in some instances, even turning into painful.
They additionally reported increased energy levels and reduced fatigue.
Medicines that interact with prednisone may both decrease its impact, have
an result on how long it works, enhance unwanted facet effects, or have much less
of an impact when taken with prednisone. An interaction between two medications doesn’t at all times mean that you have to cease taking one of the drugs;
nonetheless, typically it does. Speak to your doctor
about how drug interactions should be managed. Thus,
bodybuilders underneath our care generally utilize post-cycle remedy (PCT) to
efficiently speed up the restoration of their testosterone production.
It works to deal with patients with low levels of corticosteroids by changing steroids
which might be normally produced naturally by the physique.
It works to deal with other circumstances by decreasing swelling and redness and by altering the greatest
way the immune system works. When it involves the use of steroids,
it is necessary to understand the potential risks and legal issues.
Nevertheless, they’re additionally commonly misused for
their performance-enhancing effects in sports activities and bodybuilding.
The longer people are on steroids, the more likely they’re to expertise
unwanted aspect effects. The similar is true for individuals who have other health issues similar
to diabetes (steroids can elevate blood sugar) and osteoporosis (steroids can exacerbate bone density loss).
Whereas steroids can be beneficial for medical functions
when used under the supervision of a healthcare skilled, they also come with a range of potential risks and unwanted effects.
Some take one hundred occasions the dose legally prescribed for well being problems.
Scientifically, glucocorticoids are a particular subgroup of corticosteroids.
However they’re generally used as interchangeable
names for the same sorts of medication.
They might apply it regionally, which implies a focused dose to an actual location in or in your
physique. You may additionally take glucocorticoids systemically, which means in a way that spreads
them all through your whole body. Local steroids are extra
common as a end result of they have a lower aspect impact risk.
Inflammation (swelling) normally occurs when your immune system sends cells to
struggle infections or heal an injury. Health
conditions could make your immune system go into
overdrive. This can lead to irritation causing more problems than it helps.
Your doctor might resolve not to treat you with this treatment or
change a few of the different medicines you’re taking.
From sudden accidents to persistent conditions, Cleveland Clinic’s orthopaedic
providers can guide you through testing, remedy and beyond.
A particular person with Addison’s disease, for example,
can benefit from this remedy as their physique won’t produce sufficient cortisol.
Tell your healthcare supplier in case you are or
plan to turn out to be pregnant. Your healthcare supplier will advise you
should you should take prednisone while you are pregnant or making an attempt to get pregnant.
One of the major cons of Clenbuterol is that it may be very
dangerous and ineffective when consumed in higher doses.
While this substance was initially employed as a bronchodilator
in continual bronchial asthma treatment, it was also discovered to
have a thermogenic effect. It causes stimulation in the cells, making them launch
heat, which accelerates the body’s metabolic rate. Being a
sympathomimetic amine, Clenbuterol causes stimulation of the sympathetic
nervous system, delivering the identical results because the hormone
adrenaline (also acknowledged as epinephrine).
This class of natural compounds, steroids, performs an important role in treating situations
like eczema, hives, sciatica, and tennis elbow. Although this sort of fat is invisible (hidden internally
across the organs), it could trigger a distended look to the waistline.
This is as a end result of it’s among the best AAS
for muscular endurance; thus, cardiovascular efficiency will vastly improve
as a result of a notable enhance in red blood cell production.
For instance, 500 calories from white rice are very different from 500 energy from an avocado and three eggs.
Whereas rice provides plenty of quickly digested carbohydrates, eggs
contain high-quality protein and different nutrients wanted to construct lean physique mass, and avocados
are wealthy in wholesome fats, potassium, and fiber.
Each Brutal Pressure Sculpt Pack supplement is meticulously formulated
with natural ingredients to help particular fitness targets
similar to muscle definition, power enhancement, and muscle progress.
Personally, I think its an enormous stretch to take 8 capsules a day contemplating
all different vitamin dietary supplements that everyone who’s into health already takes.
Urge For Food suppressants are broadly favored and FDA-approved for over 12 weeks.
GLP-1 receptor agonists are well-regarded for weight problems treatment, with phentermine-topiramate
and GLP-1 agents (liraglutide, semaglutide) recognized as extremely effective.
/r/ankylosingspondylitis is a place for sufferers of ankylosing spondylitis and other axial spondyloarthritis.
These circumstances are autoimmune illnesses that trigger inflammatory arthritis of the lower
back, hips, and different joints. As mentioned earlier,
both AAS and corticosteroids can injury the liver
in the long run. There’s a purpose why bodybuilders and fitness fashions replenish on greens
at most meals.
He journey towards a more healthy life-style usually kicks off with a balanced food regimen, consistent exercise and loads of sleep.
We assessed the worth of every legal steroid for women to ensure it provides value for cash.
We looked for dietary supplements that are competitively
priced with out compromising on high quality.
If you’re not sure the place to begin, low-impact
exercise could be just what you should manage irritation (without going overboard or stressing your body out).
Also, potassium-rich meals are usually low in sodium, which might help reduce Prednisone-related bloating.
Fruits and vegetables tend to be high in potassium, including bananas,
cantaloupe, broccoli, carrots and acorn squash.
We have had sufferers who took steroids and didn’t train, and they
built muscle and burned fat regardless of being sedentary.
However, their outcomes are notably less in comparison with customers who
combine steroids with weightlifting. Nevertheless, such reductions are delicate in contrast to other
anabolic steroids.
Calcium may be found in some meals that have been fortified,
together with orange juice and cereal. Sardines are a beautiful source of calcium because
they come with their bones. Almonds offer each healthful unsaturated fat and a strong
source of calcium. The quantities and wishes of vitamins in the body may be impacted by steroid use in many ways.
As the amount of fat consumption is decreased, insulin perform will return to
its normal state. This imbalance will lead to a decreased intake of antioxidants, essential for combating oxidative
stress created by train. As A End Result Of the food regimen also lacks fiber, it
can result in an overgrowth of unhealthy intestine bacteria and
continual constipation. After 5 days of low-carb consumption,
the weekend part is designed to replenish carbohydrate shops within the body.
Of weekend energy, 60 to 80 % ought to come from carbohydrates, with 10 to 20 % from fat and 10 to 20 percent from protein. The bulk section then follows the induction part, with the primary objective of attaining a
desired bulk weight.
In Accordance to this research, published in The Journal of Asthma, the potency of inhaled corticosteroids,
used for the therapy of asthma, can influence the BMI of a patient.
In summary, Testo-Max is an all-natural legal steroid that can promote muscle growth, improve vitality levels,
and improve general bodily performance. Its secure and pure elements make it an appropriate various to
anabolic steroids. Testo-Max helps users reach health goals with out inflicting any
antagonistic side effects.
It’s additionally important to remain hydrated, get sufficient sleep, and manage stress levels to help weight reduction. Seek The Advice Of with a healthcare skilled
or registered dietitian for personalized recommendation and
guidance girls on steroids before And after the method to shed pounds safely
and sustainably. This cycle introduces Winstrol, top-of-the-line anabolic steroids for cutting.
The testosterone dose is greater to further help muscle preservation.
Growth components are extra essential for power and muscle mass in girls than in men. Since women have simply as a lot IGF-1
as women and men produce ~3 instances as much growth hormone
as men, this explains partly why having much less testosterone doesn’t limit how much muscle they will construct.
To make issues extra advanced, the sex hormones and development components work together and
all these hormones additionally work together along with your
genes.
Begin with low doses, pay close consideration to how your physique reacts, and don’t ignore any warning signs.
For ladies, the dangers can typically feel like they outweigh the rewards,
so each selection should align together with your health and long-term objectives.
Steroids are highly effective for lean muscle positive aspects,
however they come with risks that should never be
overlooked. Steroids, scientifically often identified as anabolic-androgenic steroids (AAS), are artificial derivatives of the male
sex hormone testosterone. They are designed to mimic the
effects of testosterone within the body, selling muscle development, enhancing athletic performance, and enhancing restoration instances between workouts.
Steroids are identified for their ability to extend muscle mass by
enhancing protein synthesis and nitrogen retention.
Thus, generally used compounds like Testosterone, Dianabol, and Trenbolone are not recommended for female use.
Whereas these steroids may not cause long-term physical health
points for ladies, the risk of virilization and its potential impact on psychological well-being in the long
run makes them unfavorable decisions. In our expertise,
Primobolan (methenolone) is probably considered one
of the greatest steroid cycles for females as a outcome of its mild nature.
It sometimes produces few unwanted aspect effects
however noteworthy modifications in body composition. Anavar causes reductions
in HDL ldl cholesterol, probably inflicting hypertension (high blood pressure) and increasing the risk of arteriosclerosis.
Nevertheless, in our lipid profile testing, Anavar solely causes delicate
cardiovascular strain in comparison with other anabolic steroids.
Due to decrease fan and media involvement, natural bodybuilding
pays less.
The analysis started with listening and studying the interviews in their entirety
with an open thoughts to facilitate an initial
understanding. After repeated studying, the transcript was divided
into meaning models to seek for meanings. From a phenomenological perspective and validity analysis should be meaning-oriented (van Wijngaarden et al., 2017).
When the interviews were emptied of all that means, the meanings were clustered collectively to seek out similarities and differences.
A sample of meanings slowly emerged and formed a meaningful construction that constitutes the essence of the phenomenon.
The aim is then to explain the variations and nuances of
the phenomenon, which means the constituents. This implies that the focus continues to be
on the phenomenon, however the nuances are illustrated with quotes from the informants.
Long-term use of those steroids can result in liver injury or dysfunction.
Ladies ought to opt for injectable steroids or those with a lower toxicity degree to
reduce this danger. Common liver perform exams are also recommended during a
steroid cycle.
These are just some things to look for, and it takes time and
experience to identify who is natural and who isn’t.
If it’s any consolation, you’ll solely ever have the power to say who is on steroids, as lots of the most prolific users (like long-distance cyclists) aren’t visibly in gear.
Steroids lead to excessive physique hair because of
hormones referred to as androgens. You should check the foundations and
guarantee your medicines or drugs, if any, are free of these substances.
Testosterone is an aggressive hormone and as with males, when women take testosterone they become more aggressive and prone
to robust emotions corresponding to exaggerated anger that
can lead to violence. Some women would possibly
develop neurological complications corresponding to hand and physique shaking as seen in folks with
Parkinson’s illness. Even within the much less muscular divisions,
many women are more and more turning to PED’s for an advantage.
Although it could appear silly to assume of bikini competitors
using steroids, I can guarantee you that not only does it happen,
however it occurs with regularity. This is not to recommend that each one bikini ladies use these drugs, as that definitely isn’t the case, but
the point right here is that if they’re being used in bikini, they’re getting used everywhere.
Whereas the Internet is actually a useful information useful resource, one must be able to separate the wheat from
the chaff so as to use it effectively.
Pairing it with calcium and vitamin D enhances its benefits for bone well
being, making it a triple threat for long-term well-being.
Goal for a minimal of 30 grams of protein at each meal to hold up lean mass and
optimize recovery. The higher the dosage and the longer the cycle, the upper the risk of unwanted side effects.
In our experience, profitable Anavar dosages for ladies vary from 5 to 10 mg/day.
We have had success in accelerating over the counter steroid (Geri) restoration of women’s endogenous testosterone when supplementing with DHEA, the official prescription medication for women with low androgen ranges.
Anavar suppresses endogenous testosterone, which isn’t simply problematic for males; testosterone remains an important hormone
for ladies as nicely. However, one of the best benefits of Anavar
is that it burns both visceral and subcutaneous fat, serving to ladies
achieve a smaller waist.
70918248
References:
stackers pills high, http://freeok.cn/home.php?mod=space&uid=7335920,
Every one works differently on the body, and each has its own potential side effects.
It is important to know what does Anabolic steroids do kind of
treatment you’re giving your canine and to recognize
the indicators of potential problems the drug may cause. Anabolic or “muscle-building” steroids are
artificial variations of testosterone, the male intercourse hormone.
Abusers inject or ingest steroids or apply lotions and gels at dosages that are up to one hundred occasions greater than therapeutic ranges.
Anabolic effects also embrace increased production of red blood cells.
Mineralocorticoids are responsible for maintaining the stability of
water and electrolytes within the physique while glucocorticoids play a role within the stress response.
When canine have Addison’s disease, their
adrenal glands do not produce enough of either of those two forms
of steroids – mineralocorticoids and glucocorticoids. Remedy for Addison’s illness entails day by day doses of a glucocorticoid, usually prednisone, and month-to-month injections of a mineralocorticoid, desoxycorticosterone (Percortin-V, Zycortal).
Because corticosteroids ease swelling and irritation, docs usually prescribe them to
treat circumstances like bronchial asthma, hives, or lupus.
Corticosteroids can provide substantial aid of signs, however include the risk of great unwanted effects, particularly if used long run.
One fingertip unit is the quantity of medicine distributed from the tip of the
index finger to the crease of the distal interphalangeal joint and covers roughly 2% physique surface space on an grownup.
Topical corticosteroids are applied a few times per
day for up to three weeks for super-high-potency corticosteroids or as a lot as
12 weeks for high- or medium-potency corticosteroids.
There is no specified time limit for low-potency topical corticosteroid use.
Some of the unwanted aspect effects of systemic corticosteroids
are swelling of the legs, hypertension, headache, simple bruising,
facial hair development, diabetes, cataracts, and puffiness of the
face. Glucocorticoids are the steroids most frequently prescribed by veterinarians for our canines.
Corticosteroids are a category of human-made or synthetic
medication utilized in nearly every medical specialty.
They decrease inflammation within the physique by lowering the manufacturing of sure chemical substances.
At greater doses, corticosteroids additionally cut back immune system
activity. In addition to bronchodilators, anti-inflammatory medicines, notably inhaled corticosteroids (ICS),
play a task in COPD management. While not typically beneficial as
a standalone treatment, ICS are sometimes prescribed
in combination therapy for these with average to extreme COPD.
A prescription for topical corticosteroids ought to include the medication name, focus,
formulation, directions for software, and amount. The selection of which agent,
concentration, and formulation to use depends on the condition being
handled, the location on the body, characteristics of the lesion, and patient components similar to age.
A brief course of oral steroids normally causes no side-effects.
Side-effects usually have a tendency to occur with a long course of oral steroids (more than 2-3 months), or
if quick programs are taken repeatedly. Typically the steroid remedy is gradually stopped if the situation improves,
and restarted if it worsens. The form mentioned in this leaflet
is the pill kind, taken by mouth, referred to as oral steroids.
As elite athletes are caught dishonest by utilizing anabolic steroids, perhaps their notion as positive function models
will fade and the usage of steroids decrease.
Increased stress to test athletes at younger ages
may decrease using steroids as nicely. However, so lengthy as adolescents perceive that anabolic steroids are required to compete
at sports activities, their use may continue in the foreseeable future.
This list just isn’t full and lots of different medication may have
an result on Decadron. Decadron is used to treat many various situations such as allergic issues, pores and skin situations,
ulcerative colitis, arthritis, lupus, psoriasis, or respiratory issues.
Corticosteroids are produced in the adrenal gland located above the kidney.
This medicine would possibly trigger thinning of the bones (osteoporosis)
or slow progress in kids if used for a really lengthy time.
Tell your physician if you have any bone pain or
if you have an elevated threat for osteoporosis.
If your baby is utilizing this medicine, tell the physician when you assume your youngster is not growing correctly.
Though sure medicines should not be used collectively in any respect, in other instances two different medicines may be used together even when an interaction would
possibly occur.
High blood sugar can cause fatigue, thirst, and frequent urination amongst different symptoms.
Corticosteroids also can interfere with many other
bodily processes, from your bones to your blood strain. Not everyone will develop side effects from
taking corticosteroids. Aspect effects are more
probably if corticosteroids are taken at a high dose over a protracted time period.
Tezepelumab-ekko (Tezspire) is a just lately FDA-approved biologic for folks with severe bronchial asthma.
This will vary relying on the steroid used and the condition for which they’re prescribed.
For short programs, usually a relatively high dose is prescribed each day for as much as every week, after which stopped abruptly at the
end of the course. If oral steroids are taken for longer than this it is essential to
reduce the dose steadily before stopping. Steroid medicines
(sometimes referred to as corticosteroids) are
man-made (synthetic) variations of steroid hormones produced by the body.